Print Bash Array One Per Line
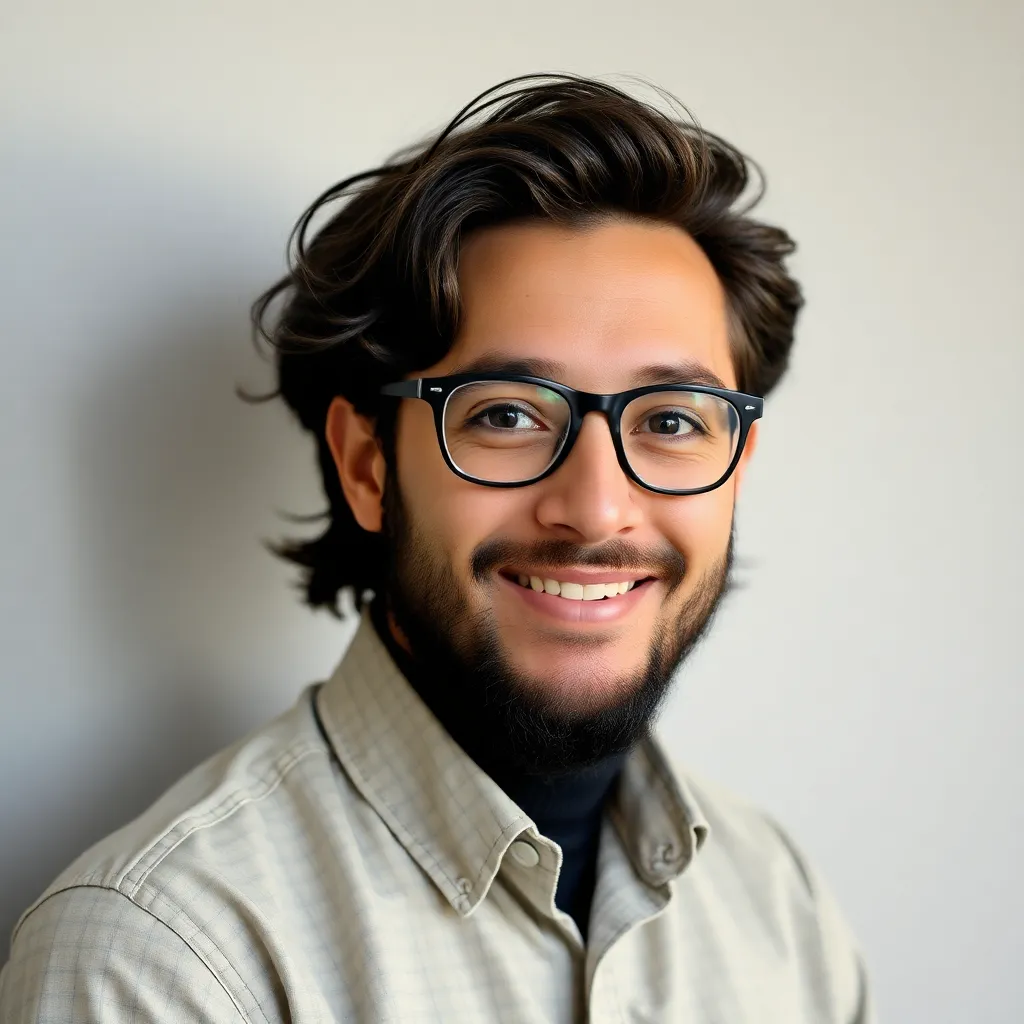
Kalali
May 24, 2025 · 3 min read
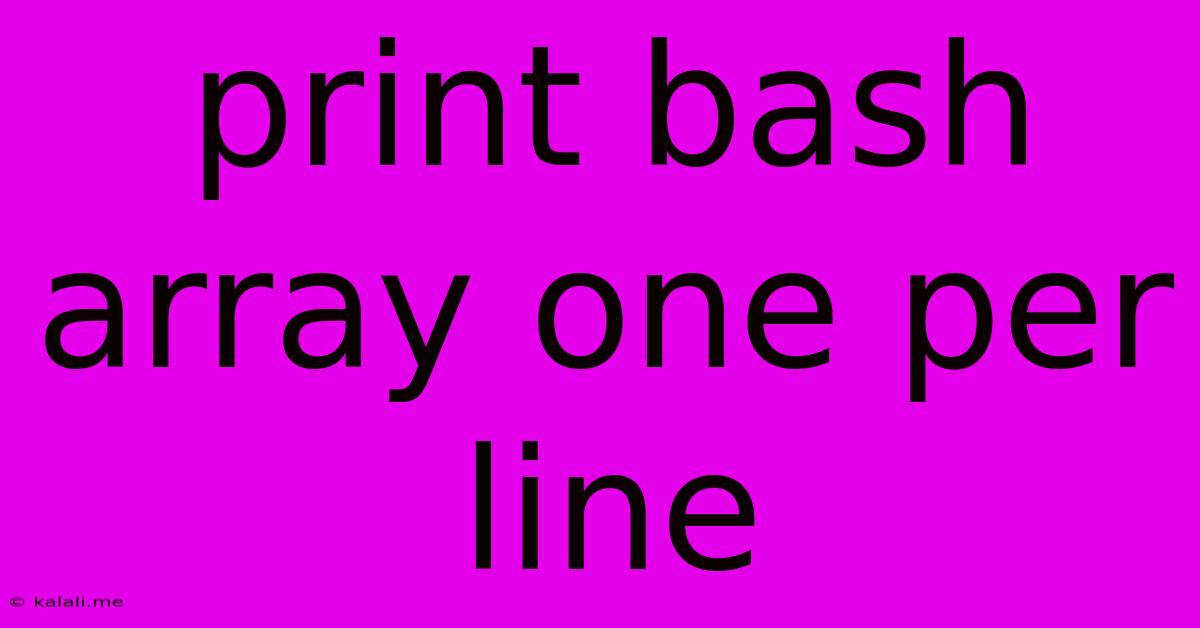
Table of Contents
Print Bash Array: One Element Per Line – A Comprehensive Guide
Printing a bash array, one element per line, is a common task, yet achieving elegant and efficient solutions requires understanding different approaches. This guide provides several methods, comparing their strengths and weaknesses, enabling you to choose the best approach for your specific needs. This will cover different scenarios and edge cases, ensuring you can handle any bash array output effectively.
Why Print One Element Per Line?
Printing array elements individually offers several advantages:
- Readability: Output is cleaner and easier to parse, both for humans and scripts.
- Debugging: Ideal for inspecting array contents during development.
- File Output: Allows easy writing of array elements to a file, one per line.
- Compatibility: Works consistently across different bash versions.
Methods to Print a Bash Array, One Element Per Line
Here are various methods to achieve this, with explanations and code examples:
Method 1: Using a for
loop
This is the most straightforward and widely understood method. It iterates through each element of the array and prints it to a new line.
my_array=("apple" "banana" "cherry" "date")
for element in "${my_array[@]}"; do
echo "$element"
done
This method is highly readable and works reliably even with elements containing spaces or special characters. The crucial use of "${my_array[@]}"
ensures proper handling of elements with spaces.
Method 2: Using printf
printf
offers more control over formatting. While this example is similar to the for
loop approach, it demonstrates the flexibility printf
provides.
my_array=("apple" "banana" "cherry" "date")
for element in "${my_array[@]}"; do
printf "%s\n" "$element"
done
%s
is the format specifier for strings, and \n
adds a newline character. This achieves the same output as the for
loop but showcases an alternative approach.
Method 3: Using Parameter Expansion with echo
(for simple cases)
For arrays with simple elements (no spaces or special characters), a more concise, albeit less robust, method exists:
my_array=("apple" "banana" "cherry" "date")
echo "${my_array[@]}" | tr ' ' '\n'
This uses tr
to replace spaces with newlines. However, this method fails if array elements contain spaces themselves. Avoid this for anything beyond very simple arrays.
Handling Empty Arrays
It's crucial to handle scenarios where the array might be empty. A simple check can prevent errors.
my_array=() # Empty array
if [ "${#my_array[@]}" -gt 0 ]; then
for element in "${my_array[@]}"; do
echo "$element"
done
else
echo "Array is empty"
fi
This example checks the array's length ("${#my_array[@]}"
) before iterating. If the array is empty, it prints a message; otherwise, it proceeds with the printing.
Conclusion
This article demonstrates several ways to print a bash array, one element per line. The for
loop method is generally recommended for its readability, robustness, and reliability in handling various array contents. The printf
method offers more formatting flexibility. Remember to handle potential empty arrays to create robust and error-free scripts. Choosing the right method depends on your specific needs and the complexity of your array data. Remember to always prioritize clear, readable code that effectively handles various scenarios.
Latest Posts
Latest Posts
-
How Much Is 4 Oz Chocolate Chips
Jul 13, 2025
-
How Many Times Does 9 Go Into 70
Jul 13, 2025
-
4 Pics 1 Word Cheat 8 Letters
Jul 13, 2025
-
220 Kilometers Per Hour To Miles Per Hour
Jul 13, 2025
-
Which Number Produces A Rational Number When Added To 0 25
Jul 13, 2025
Related Post
Thank you for visiting our website which covers about Print Bash Array One Per Line . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.