Python Strip Trailing Commas From Json String
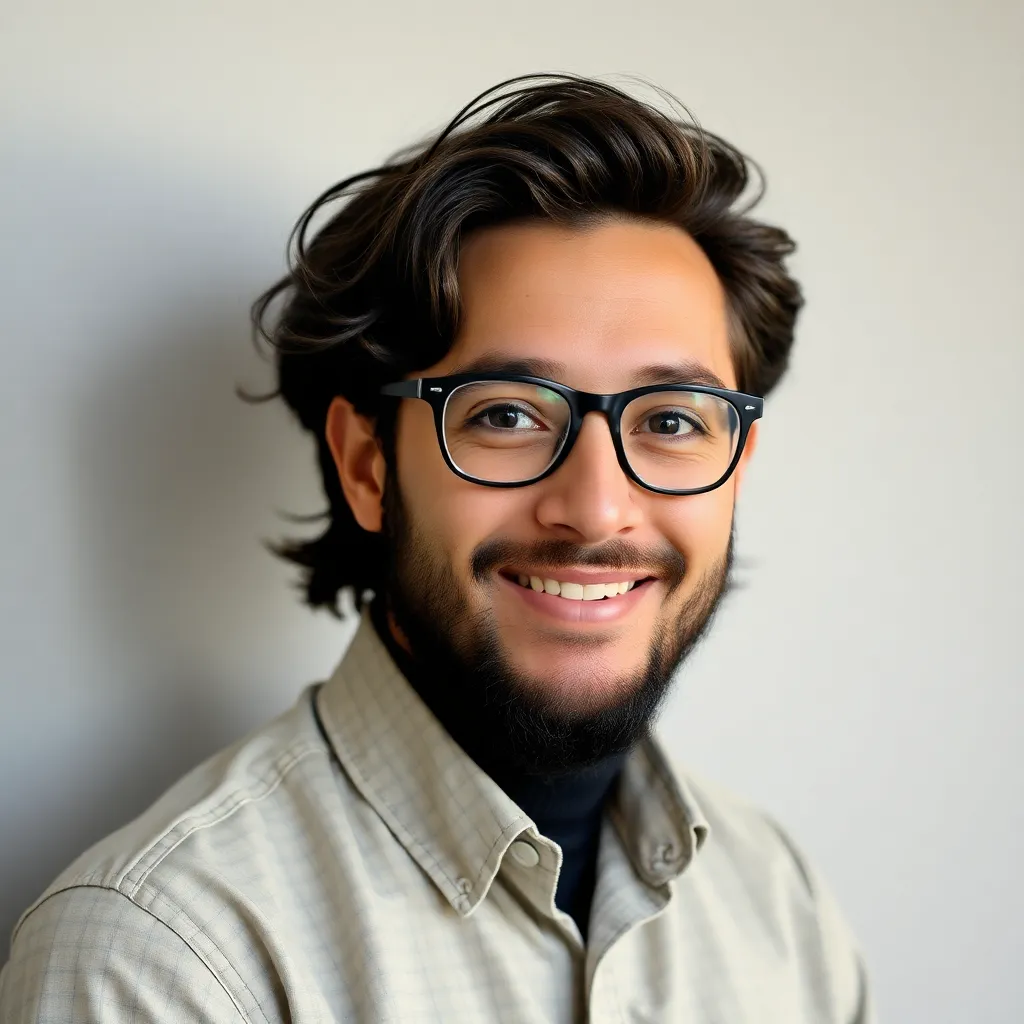
Kalali
May 27, 2025 · 3 min read
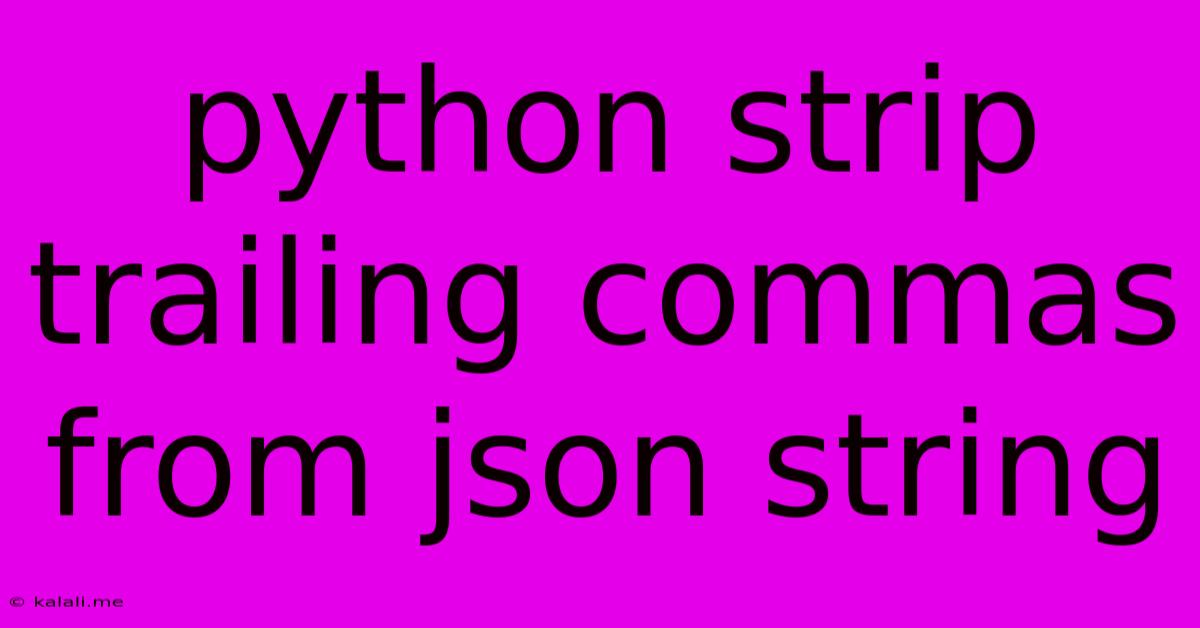
Table of Contents
Python: Stripping Trailing Commas from JSON Strings
Dealing with JSON data often involves parsing strings. Sometimes, you might encounter JSON strings with trailing commas, which are invalid according to the JSON specification. This article will guide you through several efficient methods to remove these trailing commas in Python, ensuring your JSON data is properly formatted and parsable. We'll explore various approaches, from simple string manipulation to more robust solutions handling potential complexities.
The presence of a trailing comma can render your JSON string unparsable, leading to errors when attempting to load it using Python's json
library. Understanding how to effectively clean this up is crucial for reliable data processing.
Understanding the Problem: Why Trailing Commas are Invalid
JSON (JavaScript Object Notation) has a strict syntax. A trailing comma after the last element in an array or object is not allowed. For example:
{"name": "John Doe", "age": 30,} // Invalid due to trailing comma
or
[1, 2, 3,] // Invalid due to trailing comma
These strings will cause a json.JSONDecodeError
if you try to parse them with the standard json.loads()
function.
Method 1: Using String Manipulation (Simple Cases)
For simple JSON strings with a clear trailing comma at the end, a straightforward string manipulation approach can suffice. This involves checking the last character and removing it if it's a comma.
import json
def remove_trailing_comma_simple(json_string):
if json_string.endswith(','):
return json_string[:-1]
return json_string
json_string_with_trailing_comma = '{"name": "John Doe", "age": 30,}'
cleaned_json_string = remove_trailing_comma_simple(json_string_with_trailing_comma)
try:
data = json.loads(cleaned_json_string)
print(data)
except json.JSONDecodeError as e:
print(f"Error decoding JSON: {e}")
Note: This method is only suitable for very simple cases. It will fail if the trailing comma is not at the very end of the string or if the JSON structure is more complex.
Method 2: Using Regular Expressions (More Robust)
Regular expressions offer a more robust solution for handling various scenarios. This method can detect and remove trailing commas in both objects and arrays, even within nested structures. However, it still doesn't guarantee perfect handling of all malformed JSON.
import re
import json
def remove_trailing_comma_regex(json_string):
return re.sub(r',\s*}', '}', re.sub(r',\s*\]', ']', json_string))
json_string_with_trailing_comma = '{"name": "John Doe", "age": 30,}'
cleaned_json_string = remove_trailing_comma_regex(json_string_with_trailing_comma)
try:
data = json.loads(cleaned_json_string)
print(data)
except json.JSONDecodeError as e:
print(f"Error decoding JSON: {e}")
complex_json = '[1,2,3,4,5,]'
cleaned_complex_json = remove_trailing_comma_regex(complex_json)
try:
data = json.loads(cleaned_complex_json)
print(data)
except json.JSONDecodeError as e:
print(f"Error decoding JSON: {e}")
This uses regular expressions to find and replace trailing commas within curly braces ({}
for objects) and square brackets ([]
for arrays).
Method 3: Parsing and Re-Serialization (Most Reliable)
The most reliable approach involves parsing the JSON (handling potential errors gracefully) and then re-serializing it using the json
library. This ensures correct formatting and handles more complex JSON structures effectively.
import json
def remove_trailing_comma_parse_reserialize(json_string):
try:
data = json.loads(json_string)
return json.dumps(data)
except json.JSONDecodeError as e:
print(f"Error decoding JSON: {e}")
return None # Or handle the error appropriately
json_string_with_trailing_comma = '{"name": "John Doe", "age": 30,}'
cleaned_json_string = remove_trailing_comma_parse_reserialize(json_string_with_trailing_comma)
print(cleaned_json_string)
complex_json = '[1,2,3,4,5,]'
cleaned_complex_json = remove_trailing_comma_parse_reserialize(complex_json)
print(cleaned_complex_json)
This method attempts to parse the JSON. If successful, it re-serializes it, guaranteeing valid JSON output. Error handling is included to manage cases where the input is not valid JSON even after removing the trailing comma.
Choosing the Right Method
The best method depends on the context. For simple scenarios and quick fixes, string manipulation might suffice. For more robustness, regular expressions provide a good balance. However, for maximum reliability and error handling, parsing and re-serialization is the recommended approach. Always prioritize robust error handling when dealing with external data sources. Remember to choose the method that best suits your needs and the complexity of your JSON data.
Latest Posts
Latest Posts
-
What Size Breaker For Mini Split
May 27, 2025
-
How To Clean Rusty Grill Grates
May 27, 2025
-
Ae Systome Cant Pull Items Out
May 27, 2025
-
Should You Say Goddamn As A Christian
May 27, 2025
-
How Long Does It Take Grout To Harden
May 27, 2025
Related Post
Thank you for visiting our website which covers about Python Strip Trailing Commas From Json String . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.