Python Transform Covariates With Some Basis Function
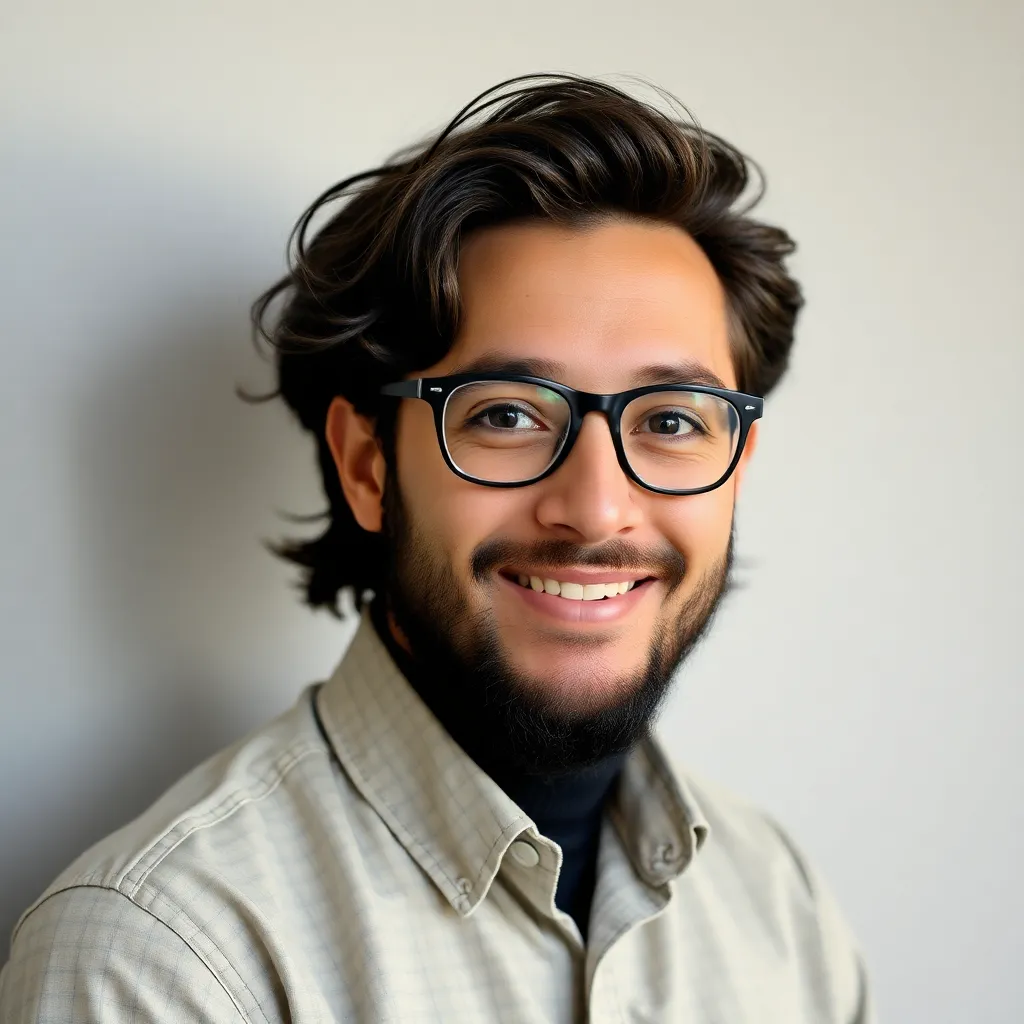
Kalali
May 25, 2025 · 3 min read
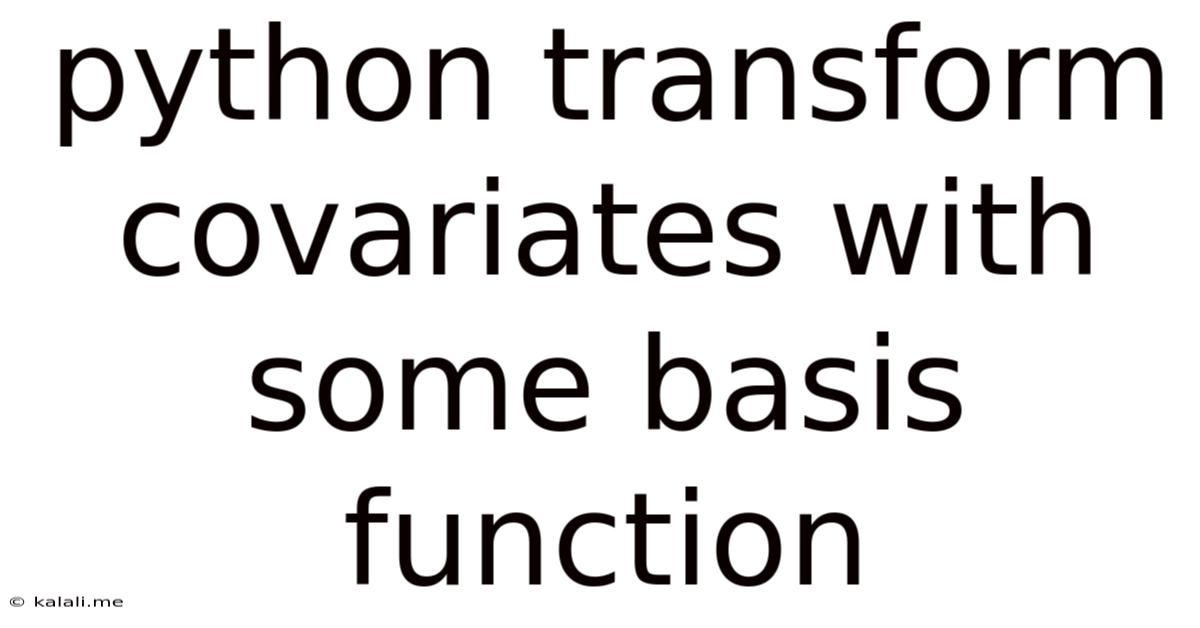
Table of Contents
Transforming Covariates with Basis Functions in Python: Enhancing Model Performance
This article explores the powerful technique of transforming covariates using basis functions within the context of statistical modeling and machine learning in Python. Understanding how to effectively use basis functions can significantly improve the predictive power and interpretability of your models. We'll cover various basis function types, their applications, and practical implementations using popular Python libraries. This guide is ideal for data scientists and analysts aiming to enhance their model building skills.
Why Transform Covariates?
Often, the relationship between a response variable and its predictors (covariates) isn't linear. Simply using the raw covariates in a linear model might lead to poor predictions and misinterpretations. Basis functions allow us to model non-linear relationships by transforming the covariates into a higher-dimensional space where linear relationships might exist. This enables us to capture complex patterns in the data.
Popular Basis Functions
Several basis function types are commonly used, each with its strengths and weaknesses:
-
Polynomial Basis: Creates polynomial terms of the covariates (e.g., x, x², x³). This is suitable for capturing smooth, curved relationships. Higher-order polynomials can model more complex curves but are prone to overfitting if the degree is too high.
-
Spline Basis: Splines are piecewise polynomial functions joined together at points called knots. They offer flexibility in modeling complex relationships while avoiding the overfitting issues associated with high-degree polynomials. Common types include cubic splines and B-splines.
-
Radial Basis Functions (RBFs): These functions are centered around specific points and decay with distance. They are effective for capturing localized patterns in the data. Gaussian RBFs are frequently used due to their smooth and localized nature.
-
Fourier Basis: Uses sinusoidal functions (sine and cosine) to model cyclical or periodic patterns in the data. This is particularly useful for time series data or data with repeating patterns.
Implementing Basis Functions in Python
Python offers various libraries to facilitate the implementation of basis functions:
-
NumPy: Provides fundamental array operations for creating and manipulating the transformed covariates.
-
SciPy: Offers functions for creating splines and other basis functions.
-
scikit-learn: Includes tools for polynomial feature expansion and other pre-processing steps.
Example: Polynomial Basis with scikit-learn
import numpy as np
from sklearn.preprocessing import PolynomialFeatures
from sklearn.linear_model import LinearRegression
# Sample data
X = np.array([[1], [2], [3], [4], [5]])
y = np.array([2, 4, 1, 3, 5])
# Create polynomial features (degree 2)
poly = PolynomialFeatures(degree=2)
X_poly = poly.fit_transform(X)
# Fit linear regression model
model = LinearRegression()
model.fit(X_poly, y)
# Make predictions
predictions = model.predict(X_poly)
print(predictions)
This code snippet demonstrates how to create polynomial features using PolynomialFeatures
and then fit a linear regression model. You can adapt this code to use other basis functions from SciPy or custom functions.
Example: Spline Basis with SciPy
Implementing spline basis functions requires a bit more setup involving choosing the knots and using SciPy's interpolate
module. The specifics depend on the type of spline chosen.
Considerations and Best Practices
-
Regularization: When using higher-order basis functions, regularization techniques (like ridge or lasso regression) are often crucial to prevent overfitting.
-
Knot Selection: For spline basis functions, the placement of knots significantly impacts the model's performance. Careful consideration should be given to knot selection strategies.
-
Basis Function Selection: The choice of basis function depends heavily on the nature of the data and the expected relationship between the covariates and the response variable. Experimentation and domain knowledge are valuable in selecting the most appropriate basis function.
-
Interpretability: While basis functions improve model flexibility, they can sometimes reduce interpretability. Careful consideration of the model's output and visualization techniques are important for understanding the model's behavior.
Conclusion
Transforming covariates using basis functions is a powerful technique for enhancing the predictive capability and flexibility of statistical and machine learning models. By carefully selecting and implementing the appropriate basis functions and addressing potential issues such as overfitting, you can significantly improve the performance and insights gained from your models. Remember to carefully consider the type of data, the expected relationship between variables, and the interpretability of your final model.
Latest Posts
Latest Posts
-
How Long Are Beans Good For In The Fridge
May 25, 2025
-
Does Whatsapp Use Your Phone Number
May 25, 2025
-
How To Remove Stickers From Car
May 25, 2025
-
Do Not Grieve The Holy Spirit
May 25, 2025
-
Email Or E Mail Or Email
May 25, 2025
Related Post
Thank you for visiting our website which covers about Python Transform Covariates With Some Basis Function . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.