Read A File Line By Line Bash
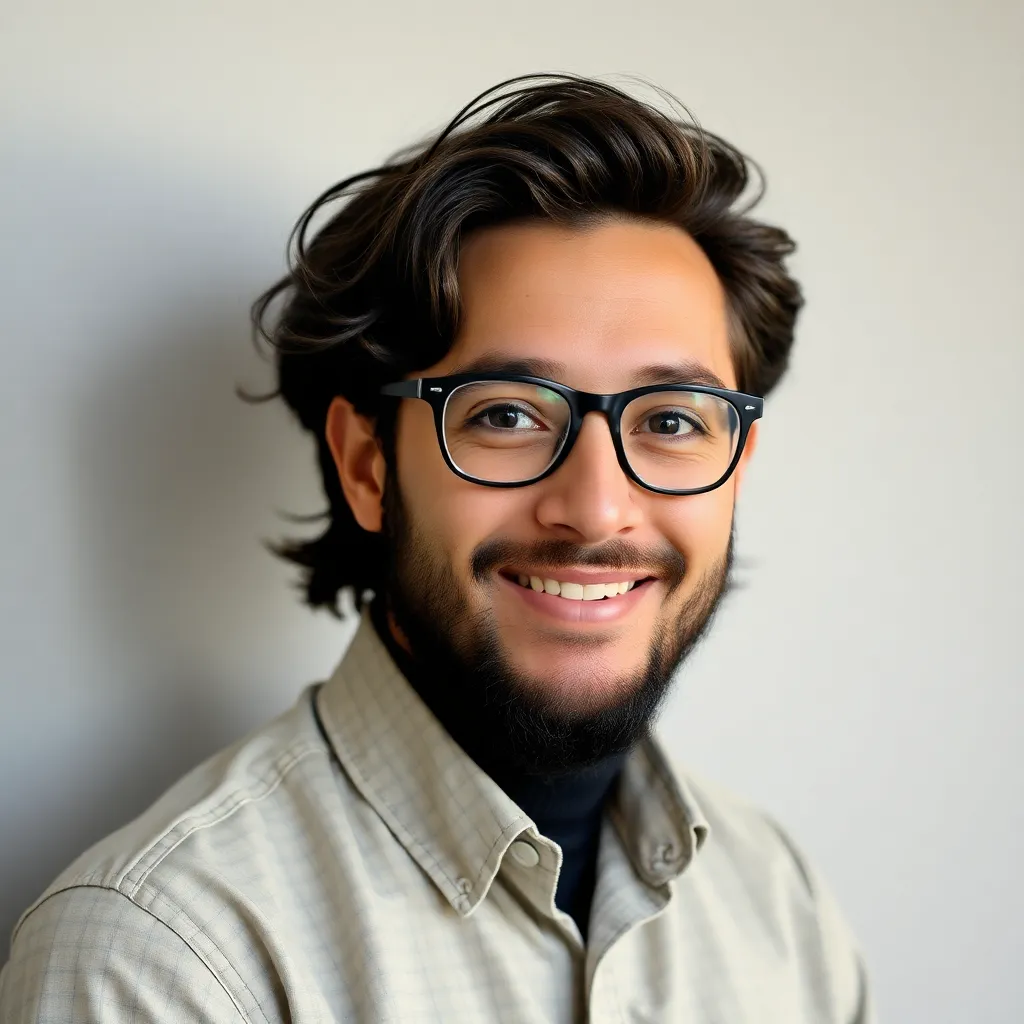
Kalali
May 20, 2025 · 3 min read
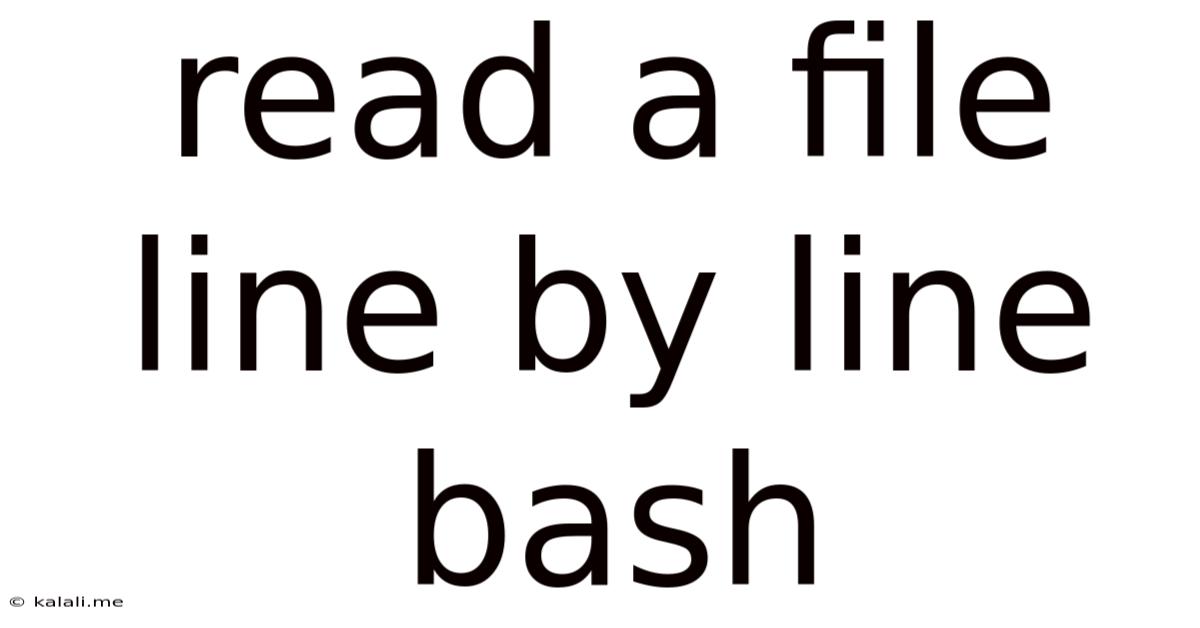
Table of Contents
Reading a File Line by Line in Bash: A Comprehensive Guide
Reading files line by line is a fundamental task in Bash scripting. This guide provides various methods, from simple loops to more advanced techniques, to efficiently process text files line by line, enhancing your Bash scripting capabilities. This includes handling large files effectively and incorporating error handling for robustness.
This article covers several ways to read files line by line in bash, from basic while
loops to more advanced techniques using readarray
and process substitution. We'll also look at handling potential errors and improving efficiency when dealing with large files.
Method 1: The while
loop with read
This is the most straightforward approach. The while
loop reads the file line by line until the end of the file is reached. The read
command assigns each line to a variable.
#!/bin/bash
filename="my_file.txt"
while IFS= read -r line; do
# Process each line here
echo "Line: $line"
done < "$filename"
#Check if the file exists
if [ ! -f "$filename" ]; then
echo "Error: File '$filename' not found."
exit 1
fi
This script first checks if the file exists before processing it. IFS= read -r line
is crucial; IFS=
prevents word splitting, and -r
prevents backslash escapes from being interpreted. Each line is then printed; you would replace echo "Line: $line"
with your desired processing logic.
Method 2: Using readarray
for improved efficiency
For larger files, readarray
offers a significant performance boost by reading the entire file into an array at once.
#!/bin/bash
filename="my_file.txt"
if [ ! -f "$filename" ]; then
echo "Error: File '$filename' not found."
exit 1
fi
readarray -t lines < "$filename"
for line in "${lines[@]}"; do
# Process each line
echo "Line: $line"
done
This method is particularly beneficial when you need to access lines multiple times or perform operations requiring random access to specific lines within the file. The -t
option removes trailing newlines from each line.
Method 3: Process Substitution for Flexibility
Process substitution offers a flexible way to handle file input, especially when dealing with commands that generate output.
#!/bin/bash
while IFS= read -r line; do
# Process each line
echo "Line: $line"
done < <(command_that_generates_lines)
Replace command_that_generates_lines
with any command that produces output line by line, such as ls -l
, grep pattern my_file.txt
, or the output of another script. This approach avoids creating temporary files.
Handling Errors and Large Files
Robust scripts should include error handling. We've already shown checking for file existence. For extremely large files, consider processing lines in chunks to manage memory usage effectively. You could modify the while
loop to read and process a specific number of lines at a time, instead of reading the entire file at once.
Conclusion
This guide explored various methods for reading files line by line in Bash. Choosing the optimal method depends on file size, processing requirements, and the overall script design. Remember to always prioritize error handling and efficient memory management, especially when working with large datasets. By understanding these techniques, you can significantly improve your Bash scripting skills and handle file processing tasks with greater efficiency and robustness.
Latest Posts
Latest Posts
-
In What Episode Of Bleach Does Ichigo Ask Orihime Out
Jul 13, 2025
-
How Much Is 4 Oz Chocolate Chips
Jul 13, 2025
-
How Many Times Does 9 Go Into 70
Jul 13, 2025
-
4 Pics 1 Word Cheat 8 Letters
Jul 13, 2025
-
220 Kilometers Per Hour To Miles Per Hour
Jul 13, 2025
Related Post
Thank you for visiting our website which covers about Read A File Line By Line Bash . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.