Remove First Character From String Python
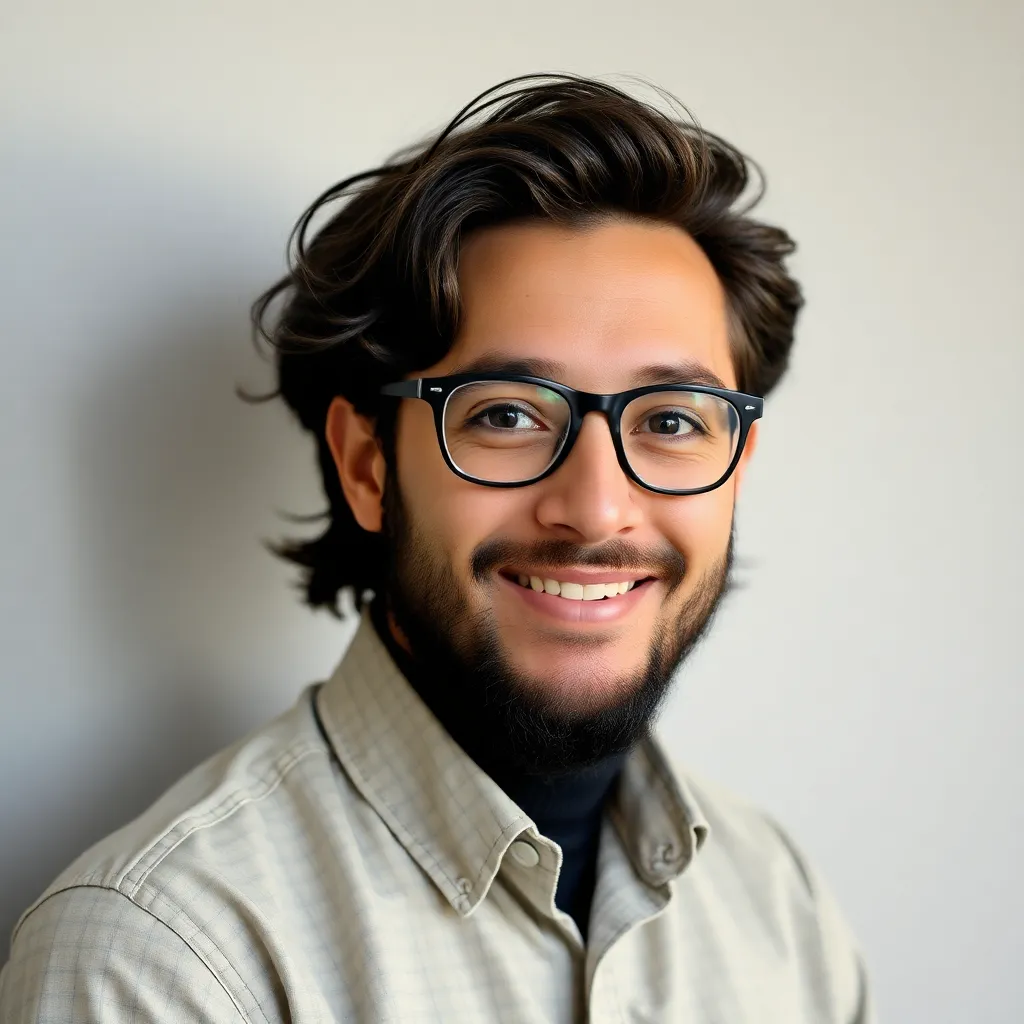
Kalali
May 22, 2025 · 3 min read
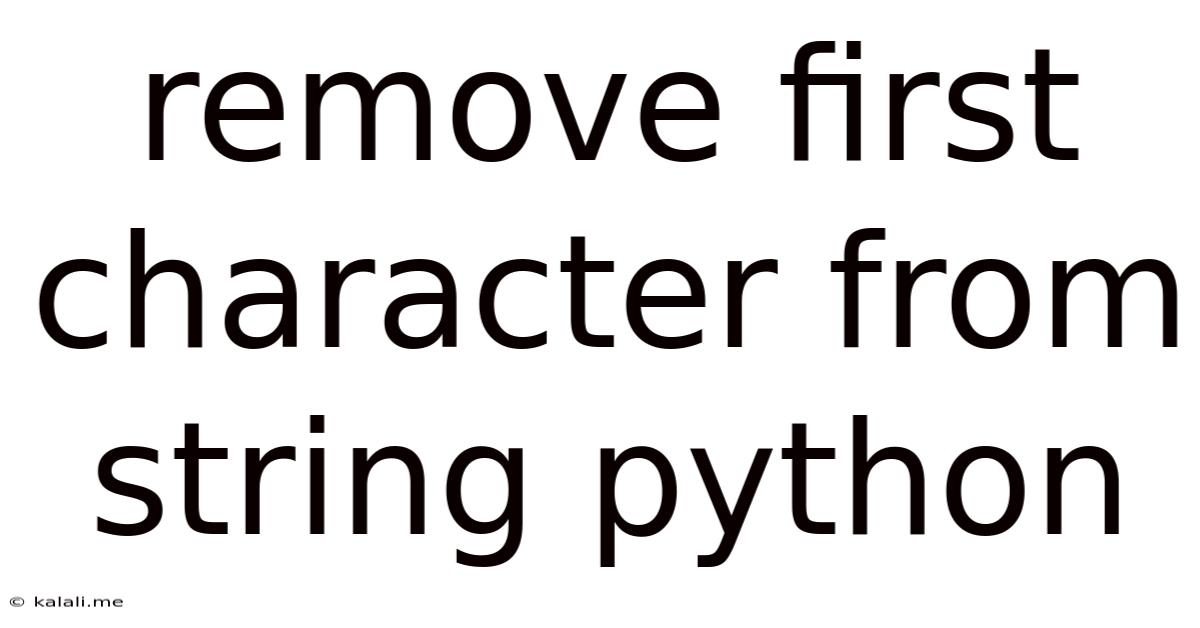
Table of Contents
Removing the First Character from a String in Python: A Comprehensive Guide
This article provides a comprehensive guide on how to remove the first character from a string in Python. We'll explore several methods, each with its own advantages and disadvantages, catering to different scenarios and coding styles. Understanding these methods will enhance your Python string manipulation skills and improve the efficiency of your code.
Why remove the first character? This operation is surprisingly common in various programming tasks, from data cleaning and preprocessing to handling user input and parsing strings from files. Often, the first character might be an unnecessary prefix, a delimiter, or simply unwanted data.
Method 1: String Slicing
This is arguably the most Pythonic and efficient way to remove the first character. String slicing allows you to extract a portion of a string, and by excluding the first character's index (0), we effectively remove it.
my_string = "Hello, world!"
new_string = my_string[1:] # Slice from index 1 to the end
print(new_string) # Output: ello, world!
This method is concise, readable, and performs well, especially with larger strings. It directly creates a new string without modifying the original. This is generally preferred for immutability reasons in Python.
Method 2: Using lstrip()
for specific character removal
The lstrip()
method removes leading characters from a string. While primarily designed for whitespace removal, it can be used to remove a specific character if it happens to be at the beginning.
my_string = "XHello, world!"
new_string = my_string.lstrip('X') #Removes leading 'X'
print(new_string) # Output: Hello, world!
This approach is particularly useful when you want to remove a specific leading character and are unsure if it exists. If the specified character is not at the beginning, lstrip()
won't modify the string. It's less efficient than slicing if you know the first character must be removed.
Method 3: Handling Edge Cases: Empty Strings and Strings with one character.
It's crucial to consider edge cases. Attempting to remove the first character from an empty string or a string with only one character will raise an IndexError
with the slicing method. To handle these gracefully, add error handling:
my_string = "" # Or "A"
try:
new_string = my_string[1:]
except IndexError:
new_string = my_string # Or handle it appropriately - e.g., return an empty string.
print(new_string)
This try-except
block prevents the code from crashing, ensuring robustness. Alternatively, you could check the string length beforehand:
my_string = "A"
if len(my_string) > 0:
new_string = my_string[1:]
else:
new_string = my_string
print(new_string)
This approach is more explicit about the handling of edge cases.
Method 4: Using replace()
(Less Efficient)
While possible, using replace()
to remove the first character is generally less efficient than slicing, particularly for larger strings. It replaces all occurrences of the first character, which is unnecessary if you only want to remove the initial one.
my_string = "Hello, world!"
first_char = my_string[0]
new_string = my_string.replace(first_char, "", 1) #Only replaces first occurrence
print(new_string) # Output: ello, world!
This method is less efficient because it scans the entire string. It's recommended to use string slicing instead for better performance.
Conclusion
Removing the first character from a string in Python is a straightforward task with multiple approaches. String slicing (my_string[1:]
) is generally the preferred and most efficient method due to its clarity, conciseness, and performance. Remember to handle edge cases (empty strings or single-character strings) gracefully to ensure your code is robust. Choosing the right method depends on your specific needs and coding style but prioritize readability and efficiency.
Latest Posts
Latest Posts
-
How To Turn Off A Burglar Alarm
May 22, 2025
-
6 Month Old Puppy Peeing In House Again
May 22, 2025
-
Can A Candle Heat Up A Room
May 22, 2025
-
What Key Is An Alto Saxophone In
May 22, 2025
-
Who Was Riley In The Saying Life Of Riley
May 22, 2025
Related Post
Thank you for visiting our website which covers about Remove First Character From String Python . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.