Run Bash Script From Bash Script
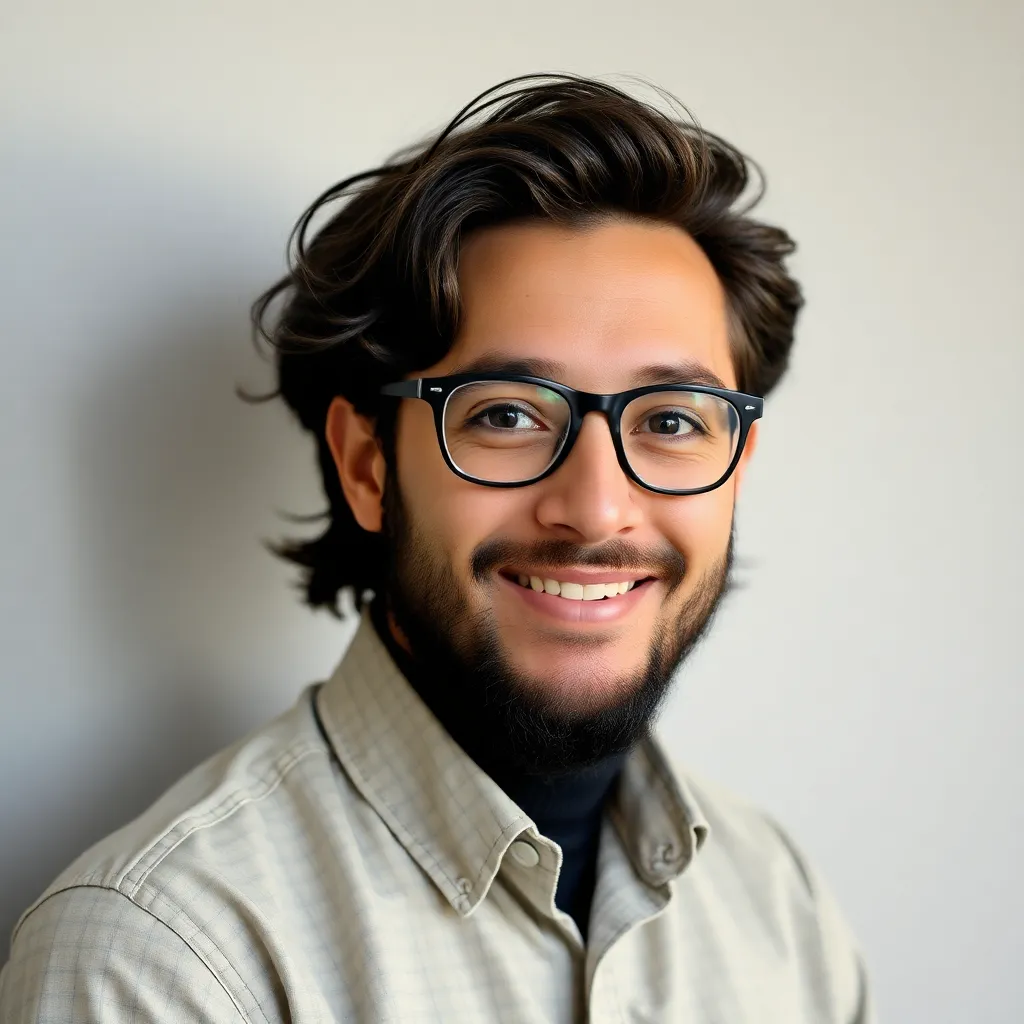
Kalali
May 19, 2025 · 3 min read
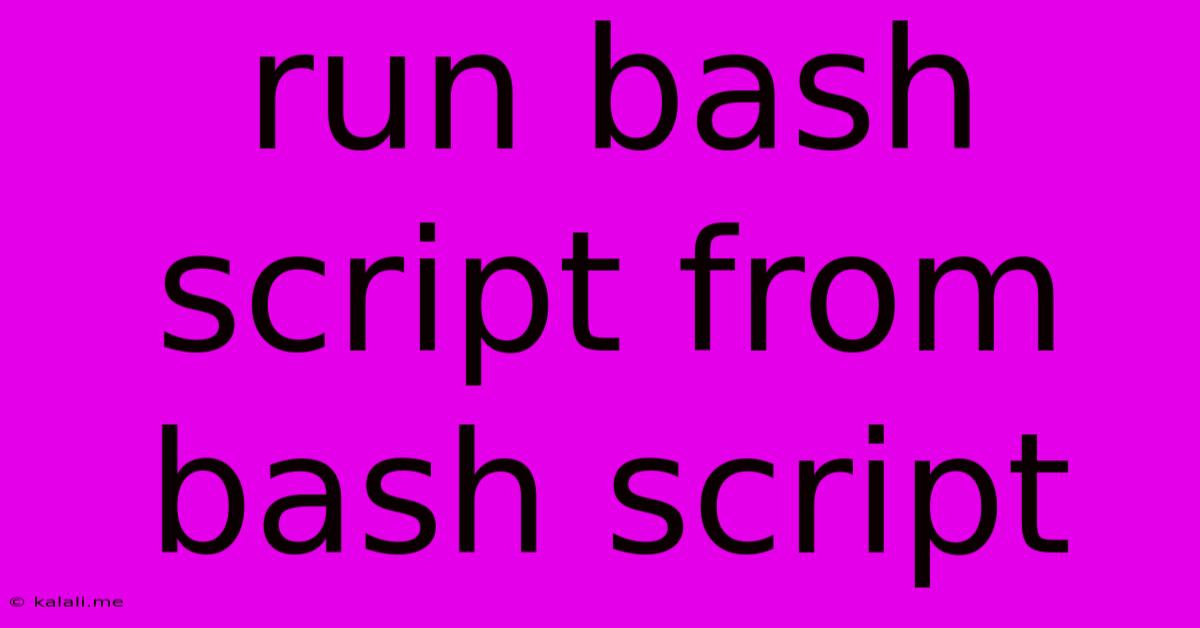
Table of Contents
Running Bash Scripts from Within Bash Scripts: A Comprehensive Guide
This article provides a comprehensive guide on how to execute one Bash script from another. This is a common task in scripting, allowing for modularity, reusability, and cleaner code organization. We'll explore various methods, best practices, and troubleshooting techniques. Understanding this process is crucial for any serious Bash scripting endeavor.
Why Run Bash Scripts from Other Bash Scripts?
Running a Bash script from another offers several advantages:
- Modularity: Break down complex tasks into smaller, manageable scripts. This improves readability and maintainability.
- Reusability: Create reusable functions and scripts to avoid code duplication.
- Organization: Improve the overall structure and organization of your scripting projects.
- Efficiency: Avoid repeating the same commands across multiple scripts.
Methods for Executing Bash Scripts:
There are several ways to run a Bash script from another:
- Using
source
(or.
): This method executes the script within the current shell environment. Changes made to variables within the called script will persist in the calling script.
source ./my_script.sh # or . ./my_script.sh
- Using
bash
(orsh
): This method executes the script in a subshell. Changes to variables within the called script will not affect the calling script. This is generally the preferred method for better isolation.
bash ./my_script.sh # or sh ./my_script.sh
- Using
exec
: This replaces the current shell process with the called script. Use this with caution, as it will terminate the original script.
exec ./my_script.sh
Passing Arguments to Sub-scripts:
You can easily pass arguments to the called script using standard command-line argument syntax:
#!/bin/bash
argument1="hello"
argument2="world"
./my_script.sh "$argument1" "$argument2"
Within my_script.sh
, you can access these arguments using $1
, $2
, etc.
Best Practices:
- Shebang: Always include a shebang at the beginning of your scripts to specify the interpreter:
#!/bin/bash
. - Error Handling: Implement proper error handling to gracefully manage potential issues. Check exit codes using
$?
. - Absolute Paths: Use absolute paths to your scripts to avoid ambiguity.
- Quoting: Always quote variables to prevent word splitting and globbing issues.
- Clear Variable Names: Use descriptive variable names for better readability.
- Comments: Add comments to explain the purpose of each script and its functionality.
Example:
Let's say we have two scripts: main.sh
and sub.sh
.
main.sh
:
#!/bin/bash
name="John Doe"
greeting="Hello"
./sub.sh "$name" "$greeting"
echo "Back in main script"
sub.sh
:
#!/bin/bash
name="$1"
greeting="$2"
echo "$greeting, $name!"
Running main.sh
will output:
Hello, John Doe!
Back in main script
Troubleshooting:
- Permission Errors: Ensure your scripts have execute permissions using
chmod +x my_script.sh
. - Path Issues: Double-check the paths to your scripts. Use absolute paths if necessary.
- Syntax Errors: Carefully review your scripts for syntax errors. Use a text editor with syntax highlighting for Bash.
By following these guidelines and understanding the various methods for executing Bash scripts, you can create more efficient, modular, and maintainable Bash scripting projects. Remember to prioritize clear code, robust error handling, and well-defined functions to ensure the long-term success of your scripting endeavors.
Latest Posts
Latest Posts
-
Can You Drink Water In Greece
May 20, 2025
-
Why Is Forking A Lawn Bad
May 20, 2025
-
The Lion The Witch And The Wardrobe Jadis
May 20, 2025
-
Signs My Dog Wants To Kill My Cat
May 20, 2025
-
Can Stainless Steel Go In The Oven
May 20, 2025
Related Post
Thank you for visiting our website which covers about Run Bash Script From Bash Script . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.