Run Bash Script In Bash Script
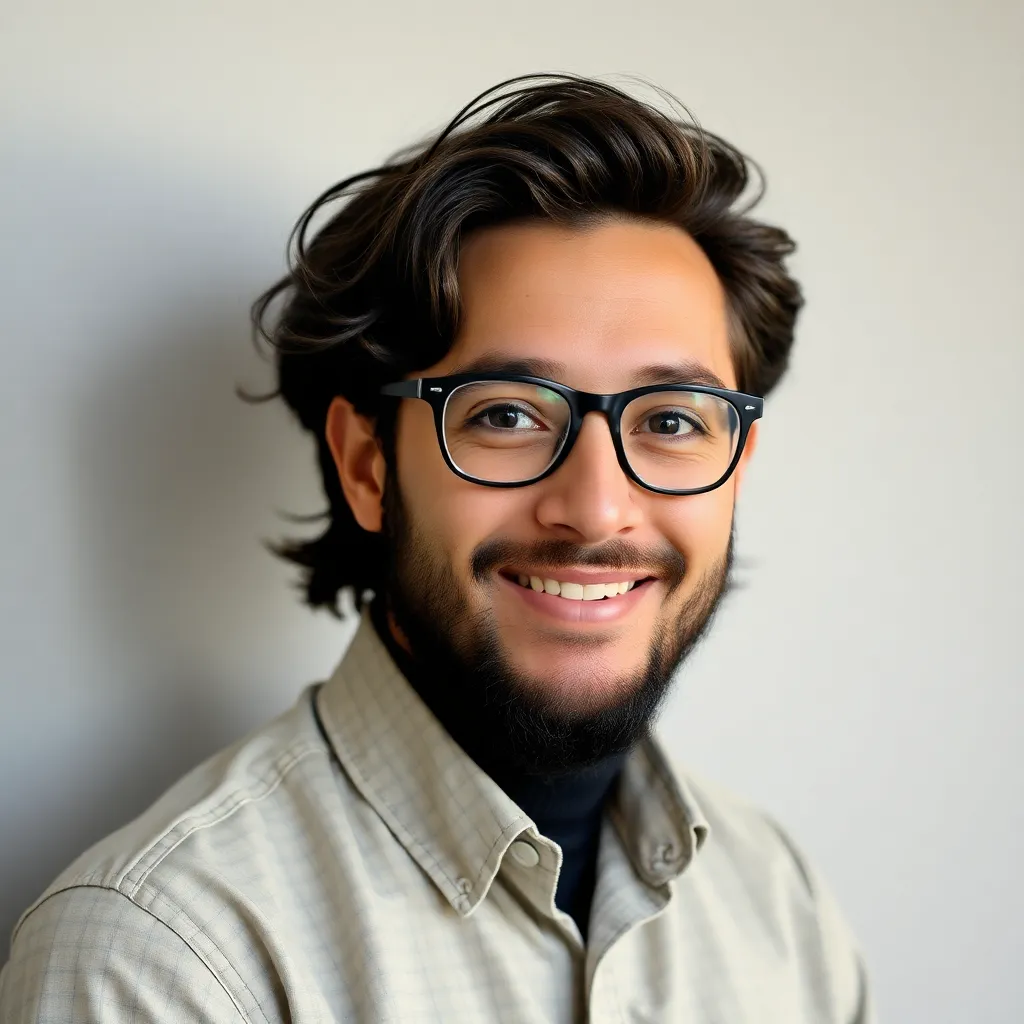
Kalali
May 25, 2025 · 3 min read
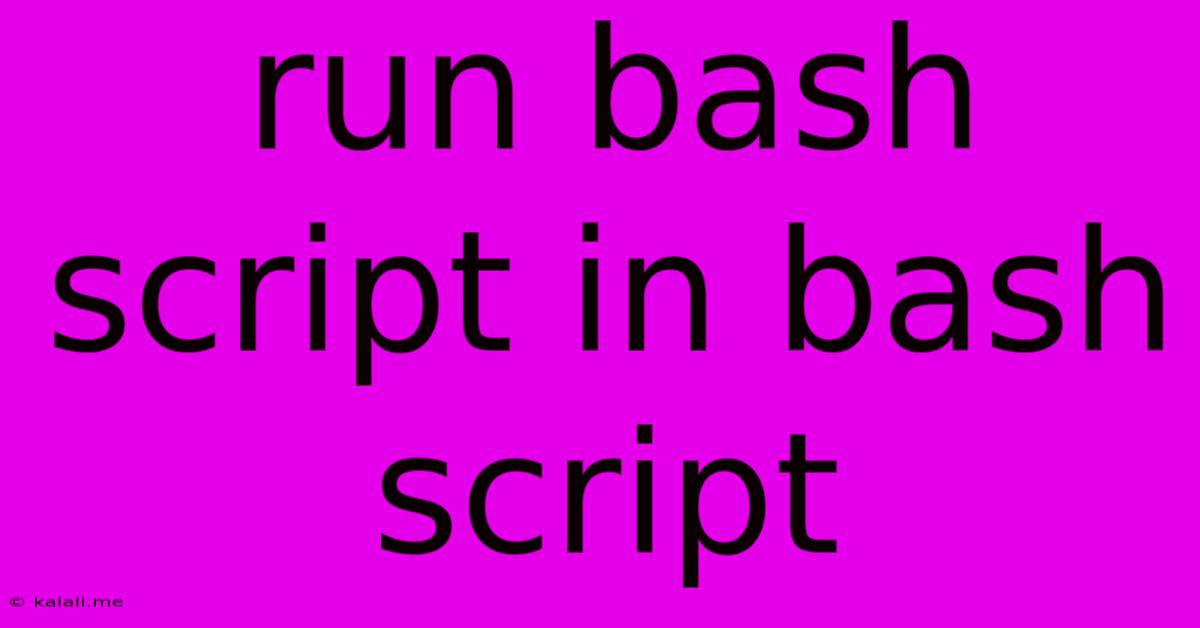
Table of Contents
Running Bash Scripts from Within Bash Scripts: A Comprehensive Guide
This guide delves into the intricacies of executing one bash script from another, a common practice in shell scripting that streamlines automation and improves code organization. We'll explore various methods, best practices, and potential pitfalls to ensure your scripts run smoothly and efficiently. This is crucial for anyone building complex automation workflows or managing server-side processes.
Why Run a Bash Script from Another?
Executing a bash script within another provides several key advantages:
- Modularity: Breaking down large tasks into smaller, manageable scripts improves readability, maintainability, and reusability.
- Reusability: Common functions can be encapsulated in separate scripts and called repeatedly, avoiding code duplication.
- Organization: A well-structured project with multiple scripts is far easier to understand and debug than a single monolithic script.
- Error Handling: Individual scripts can have their own error handling mechanisms, making debugging simpler.
- Parallel Processing: You can run multiple scripts concurrently to speed up processing of large tasks.
Methods for Executing Bash Scripts
There are several ways to run a bash script from within another:
1. Using source
(or .
):
This method executes the script within the current shell environment. Changes made to environment variables within the called script will persist in the calling script.
source ./my_script.sh
# or
. ./my_script.sh
Advantages: Environment variable changes are preserved. Faster execution since a new subshell isn't created.
Disadvantages: Errors in the called script can directly impact the calling script, potentially leading to cascading failures. Not suitable for scripts that need to run independently.
2. Using bash
:
This method executes the script in a separate subshell. Changes to environment variables within the called script will not affect the calling script.
bash ./my_script.sh
Advantages: Independent execution; errors in one script won't necessarily crash the other. Better for scripts with potential side effects.
Disadvantages: Slightly slower due to subshell creation. Environment variable changes are not shared.
3. Using sh
:
Similar to bash
, but uses the system's default shell, which might not always be bash. This is generally less preferred unless you have a specific reason to use the default shell.
sh ./my_script.sh
4. Passing Arguments:
You can pass arguments to the called script using standard command-line argument syntax:
bash ./my_script.sh argument1 argument2
Inside my_script.sh
, you would access these arguments using $1
, $2
, etc.
Best Practices and Considerations
- Shebang: Always include a shebang at the beginning of your scripts to specify the interpreter (e.g.,
#!/bin/bash
). - Error Handling: Implement robust error handling using
$?
(exit status) to check if the called script executed successfully. - Absolute Paths: Use absolute paths (e.g.,
/path/to/my_script.sh
) to avoid ambiguity and potential issues with the current working directory. - Clear Naming Conventions: Choose descriptive names for your scripts and functions.
- Modular Design: Break down complex tasks into smaller, reusable modules.
- Input Validation: Validate any input received from the called script to prevent unexpected behavior.
Example:
Let's say we have two scripts: greet.sh
and main.sh
.
greet.sh
:
#!/bin/bash
echo "Hello, $1!"
main.sh
:
#!/bin/bash
name="World"
bash ./greet.sh "$name"
echo "Script execution completed."
Running main.sh
will output:
Hello, World!
Script execution completed.
By following these guidelines, you can effectively and safely incorporate the practice of running bash scripts within bash scripts, enhancing your shell scripting capabilities and creating more sophisticated and maintainable automation solutions. Remember to always prioritize clear code, robust error handling, and a well-defined structure for your projects.
Latest Posts
Latest Posts
-
30 X 30 Is How Many Square Feet
Jul 12, 2025
-
How Much Does A Half Oz Weigh
Jul 12, 2025
-
Calories In An Omelette With 3 Eggs
Jul 12, 2025
-
How Do You Say Great Grandmother In Spanish
Jul 12, 2025
-
What Is 873 Rounded To The Nearest Hundred
Jul 12, 2025
Related Post
Thank you for visiting our website which covers about Run Bash Script In Bash Script . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.