Runtimeerror: Mat1 And Mat2 Shapes Cannot Be Multiplied
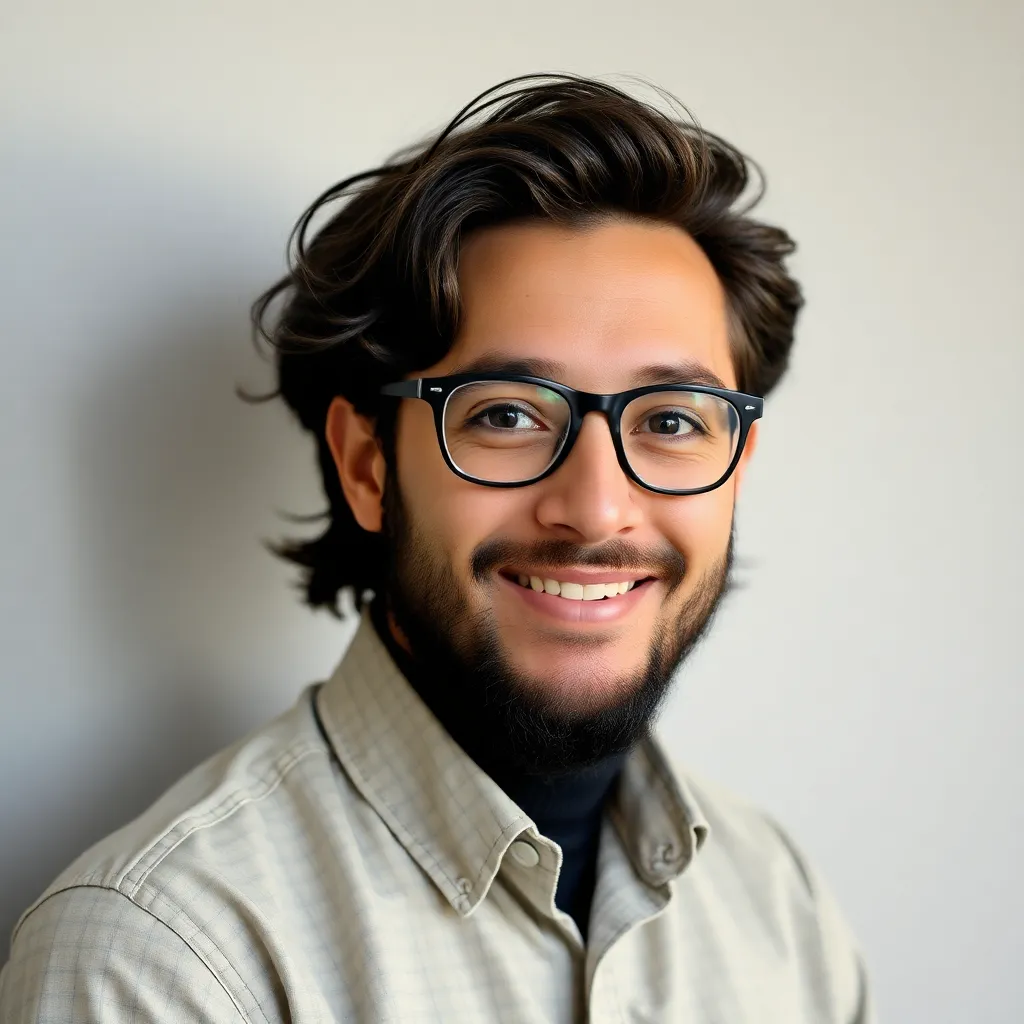
Kalali
May 19, 2025 · 4 min read
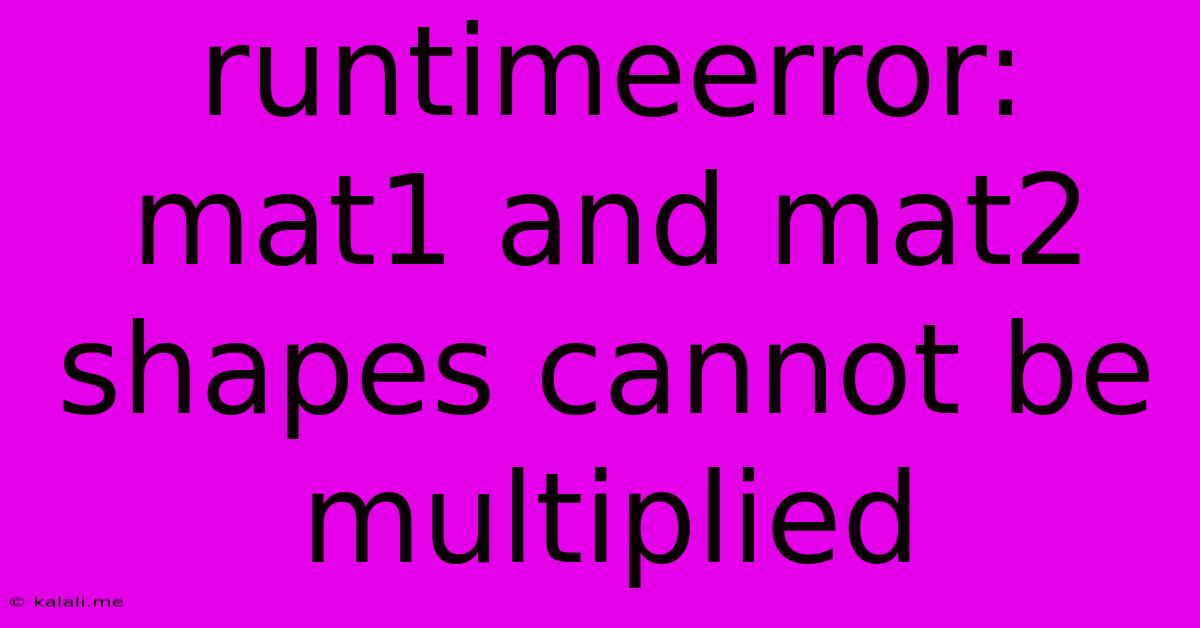
Table of Contents
RuntimeError: mat1 and mat2 shapes cannot be multiplied: A Comprehensive Guide to Troubleshooting Matrix Multiplication in Python
This error, RuntimeError: mat1 and mat2 shapes cannot be multiplied
, is a common headache for anyone working with matrix operations in Python, particularly when using libraries like NumPy. This comprehensive guide will dissect the root cause of this error, provide clear explanations, and offer practical solutions to get your matrix multiplication working flawlessly. Understanding matrix dimensions and the rules of multiplication is crucial for avoiding this frustrating issue.
Understanding Matrix Multiplication
Before diving into the error itself, let's refresh the fundamental rules of matrix multiplication. Two matrices can only be multiplied if the number of columns in the first matrix (mat1) is equal to the number of rows in the second matrix (mat2). The resulting matrix will have the same number of rows as mat1 and the same number of columns as mat2.
For example:
- A matrix with shape (3, 2) can be multiplied by a matrix with shape (2, 4). The result will have a shape of (3, 4).
- A matrix with shape (2, 3) cannot be multiplied by a matrix with shape (3, 2). This will result in the
RuntimeError
.
Causes of the RuntimeError
The RuntimeError: mat1 and mat2 shapes cannot be multiplied
error arises precisely when this fundamental rule is violated. The dimensions of your matrices are incompatible for multiplication. Here's a breakdown of common scenarios:
-
Incorrect Matrix Dimensions: This is the most frequent cause. Carefully check the shape of each matrix using the
.shape
attribute in NumPy. Ensure that the number of columns in the first matrix matches the number of rows in the second. -
Data Type Mismatch: Although less common, ensure both matrices are of compatible numerical data types (e.g., both
float64
orint32
). Inconsistent data types can sometimes lead to unexpected errors, potentially masked as shape mismatches. -
Accidental Reshaping: If you've performed operations that reshape your matrices unintentionally (e.g., slicing, transposing without proper handling), this can lead to shape inconsistencies. Double-check all operations that modify the matrix dimensions.
-
Typos or Logical Errors: Simple typos in indexing or accessing matrix elements can result in unexpected shapes. Carefully review your code for any errors.
Debugging and Troubleshooting Strategies
Here’s a systematic approach to identifying and resolving the error:
-
Print Matrix Shapes: Begin by printing the shapes of both matrices using
print(mat1.shape)
andprint(mat2.shape)
. This immediately reveals if the dimensions are incompatible. -
Inspect Matrix Creation: Examine the code that creates your matrices. Are the dimensions being initialized correctly? Are there any potential off-by-one errors?
-
Step Through the Code: Use a debugger (like pdb in Python) to step through your code line by line. This allows you to inspect the values and shapes of your matrices at each stage, isolating the source of the problem.
-
Transpose Matrices: If the shapes are close but not quite right, you may need to transpose one of the matrices using the
.T
attribute. Transposing swaps rows and columns, potentially making the multiplication possible. However, ensure this aligns with the intended mathematical operation. -
Verify Data Types: Confirm that both matrices use the same numeric data type. If not, you might need to cast them to a compatible type.
Example Scenario and Solution:
Let's imagine you have the following code:
import numpy as np
mat1 = np.array([[1, 2], [3, 4]]) # Shape (2, 2)
mat2 = np.array([[5, 6, 7], [8, 9, 10]]) # Shape (2, 3)
result = np.dot(mat1, mat2)
print(result)
This code will not produce an error, because the number of columns in mat1
(2) equals the number of rows in mat2
(2). However, if we change mat2
to:
mat2 = np.array([[5, 6], [7, 8], [9, 10]]) # Shape (3, 2)
Then attempting np.dot(mat1, mat2)
will raise the RuntimeError
because now the number of columns in mat1
(2) does not equal the number of rows in mat2
(3). To fix it, you might need to transpose mat2
or reconsider the intended matrix multiplication.
By understanding the rules of matrix multiplication and employing these debugging techniques, you can effectively resolve the RuntimeError: mat1 and mat2 shapes cannot be multiplied
and confidently perform matrix operations in your Python programs. Remember to always double-check your matrix dimensions!
Latest Posts
Latest Posts
-
How Many Grams Of Sugar In A Pound
Jul 12, 2025
-
7am To 11am Is How Many Hours
Jul 12, 2025
-
If Your 35 What Year Was You Born
Jul 12, 2025
-
How Many Cups Is 1 Pound Of Cheese
Jul 12, 2025
-
30 X 30 Is How Many Square Feet
Jul 12, 2025
Related Post
Thank you for visiting our website which covers about Runtimeerror: Mat1 And Mat2 Shapes Cannot Be Multiplied . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.