Salesforce Aura Component Run Another Action When Action Finished
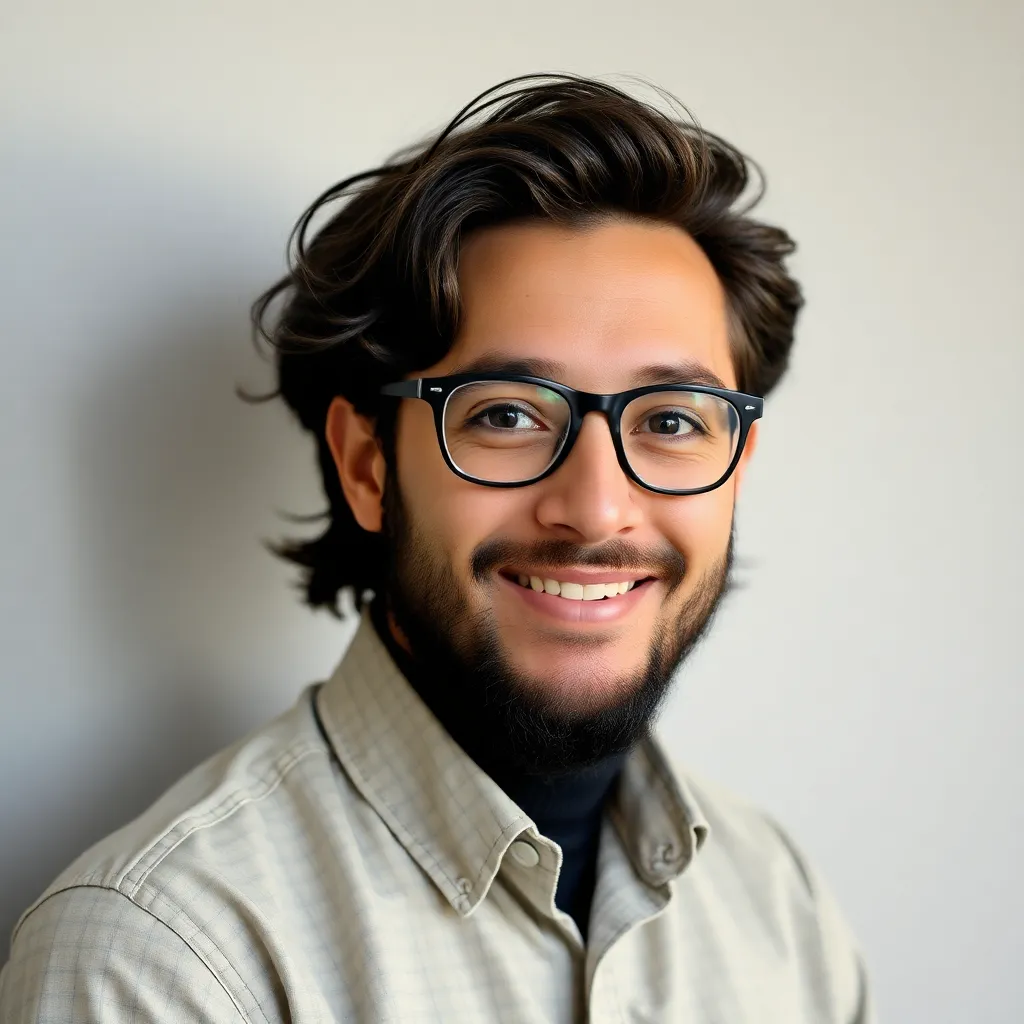
Kalali
May 22, 2025 · 3 min read
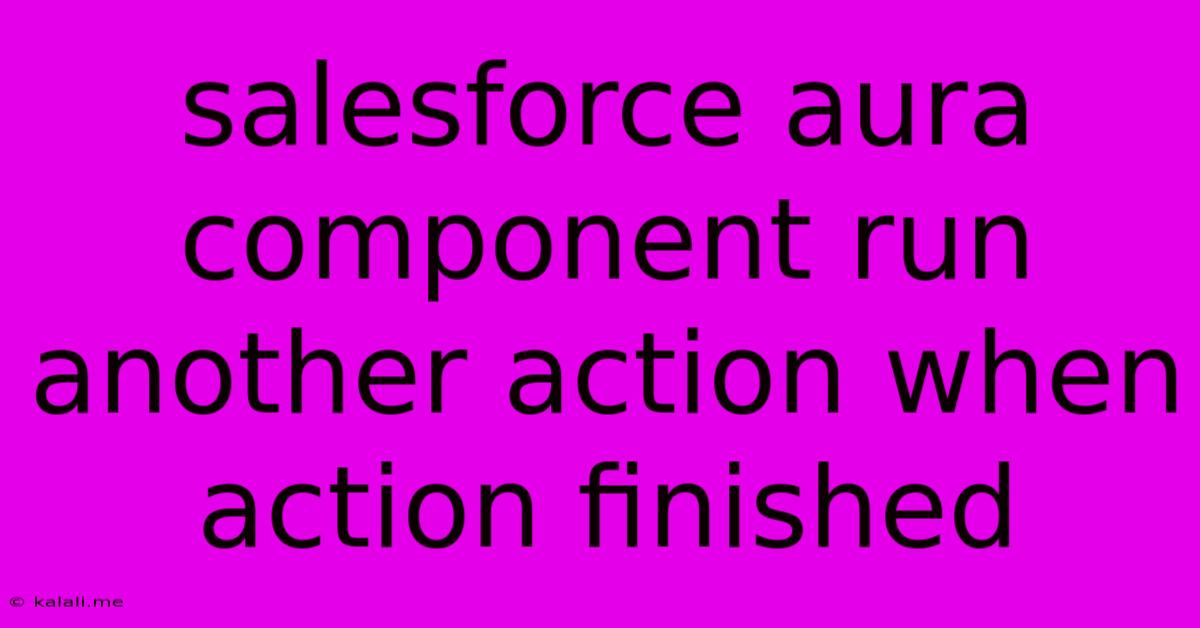
Table of Contents
Salesforce Aura Component: Chaining Actions for Seamless User Experience
Salesforce Aura components offer a powerful framework for building dynamic and interactive user interfaces. Often, you'll need one action to complete before another can begin, creating a smooth and intuitive user experience. This article explains how to chain Aura component actions, ensuring one action successfully finishes before the next begins. This is crucial for tasks like data saving, followed by a UI update reflecting the saved data. We'll explore various approaches to achieve this, improving the overall efficiency and responsiveness of your application.
This technique is important for preventing race conditions and ensuring data integrity within your Salesforce applications. By properly sequencing actions, you eliminate the possibility of unintended consequences resulting from asynchronous operations. This leads to a more reliable and user-friendly experience.
Understanding Asynchronous Operations in Aura
Aura components often utilize asynchronous operations, particularly when interacting with the Salesforce server. These operations, such as $A.get
, $A.enqueueAction
, or server-side Apex calls, don't block the execution flow. This means the next line of code executes before the asynchronous operation completes. This can lead to unpredictable behavior if not handled correctly.
Method 1: Using Callbacks
The simplest and most common method is leveraging callbacks. Callbacks are functions executed after an asynchronous operation finishes. This allows you to chain actions sequentially.
// Example Aura Controller JavaScript
({
myAction : function(component, event, helper) {
var action = component.get("c.myApexMethod"); // Apex method to perform first action
action.setCallback(this, function(response) {
var state = response.getState();
if (state === "SUCCESS") {
// First action successful, execute the second action
var secondAction = component.get("c.mySecondApexMethod");
secondAction.setCallback(this, function(secondResponse) {
var secondState = secondResponse.getState();
if (secondState === "SUCCESS") {
// Both actions successful, update UI
component.set("v.message", "Both actions completed successfully!");
} else {
// Handle errors for the second action
console.error("Error in second action: ", secondResponse.getError());
}
});
$A.enqueueAction(secondAction);
} else {
// Handle errors for the first action
console.error("Error in first action: ", response.getError());
}
});
$A.enqueueAction(action);
}
})
This code demonstrates a nested callback structure. The second action only executes after the first action successfully completes. Error handling is included for both actions, enhancing robustness.
Method 2: Promises (More Advanced)
For more complex scenarios involving multiple asynchronous operations, using Promises provides a cleaner and more manageable approach. Promises offer a more structured way to handle asynchronous operations and their results. However, this requires a deeper understanding of JavaScript Promises. While not directly supported by the Aura framework's built-in functions, you can still leverage the Promise API within your Aura controller. This allows for better readability and maintainability, especially with more chained actions.
(Note: Providing a complete Promise-based example here would be lengthy and detract from the core concept of chaining actions. However, researching JavaScript Promises and their integration within Aura controllers will provide the necessary knowledge to implement this more sophisticated approach.)
Best Practices for Chaining Actions
- Error Handling: Always include comprehensive error handling to gracefully manage failures in any action.
- User Feedback: Provide visual feedback to the user, such as loading indicators, to inform them of the ongoing process.
- Keep Actions Atomic: Design your Apex methods to perform single, well-defined tasks. This improves maintainability and debugging.
- Consider Transactions (Apex): If the actions need to be treated as a single unit of work (all succeed or all fail), wrap them in a single Apex transaction.
By implementing these strategies, you can create Aura components with a more responsive and reliable user experience, ensuring a seamless flow between different actions and minimizing the risk of errors or unexpected behavior. Remember to choose the method best suited to your complexity and proficiency. For simple chaining, callbacks are sufficient; for more involved processes, Promises provide a robust solution.
Latest Posts
Latest Posts
-
If Your 35 What Year Was You Born
Jul 12, 2025
-
How Many Cups Is 1 Pound Of Cheese
Jul 12, 2025
-
30 X 30 Is How Many Square Feet
Jul 12, 2025
-
How Much Does A Half Oz Weigh
Jul 12, 2025
-
Calories In An Omelette With 3 Eggs
Jul 12, 2025
Related Post
Thank you for visiting our website which covers about Salesforce Aura Component Run Another Action When Action Finished . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.