Sql Loop[ Thoug For Each Balue In The List
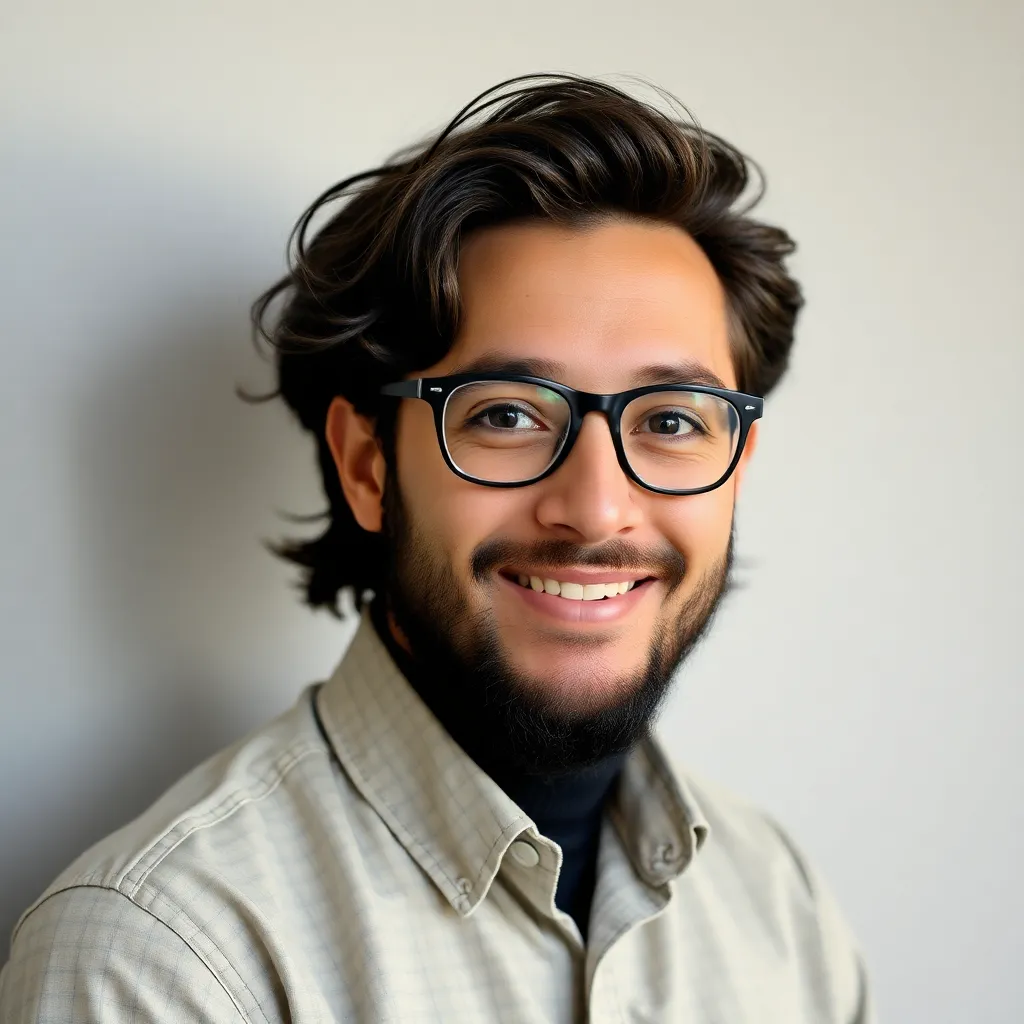
Kalali
May 25, 2025 · 3 min read
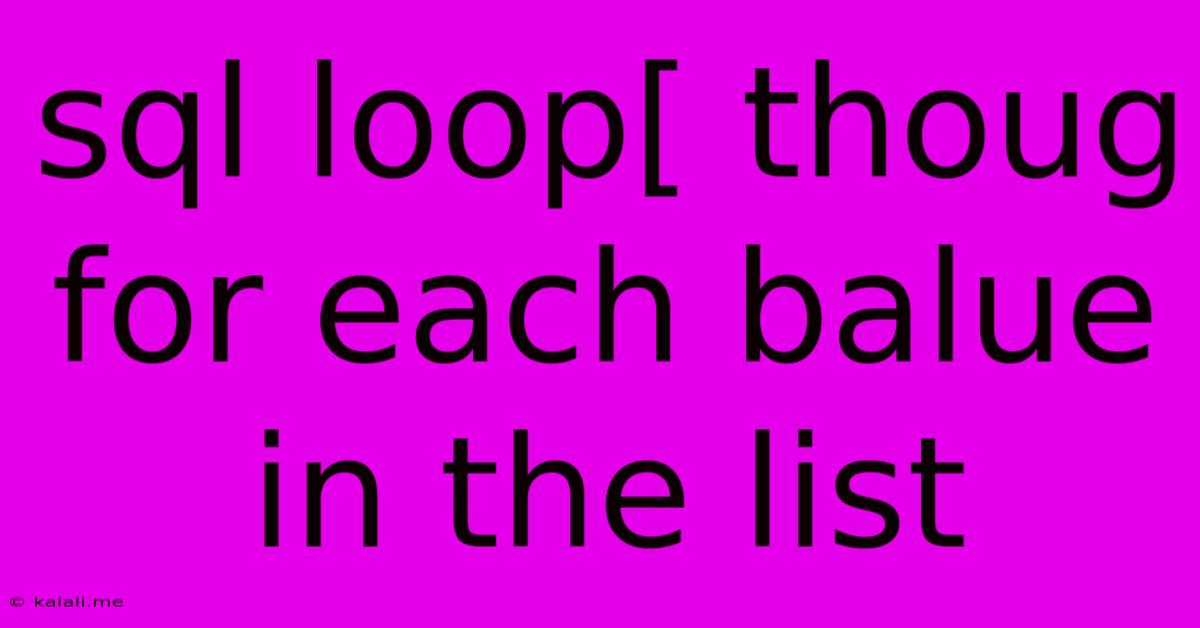
Table of Contents
SQL Loops: Iterating Through Lists and Processing Data
SQL doesn't have explicit loop constructs like for
or foreach
found in procedural programming languages. However, there are several ways to achieve iterative processing of data within a SQL database. The best approach depends on your specific needs and the database system you're using. This article explores common techniques for handling iterative tasks in SQL, focusing on processing lists of values.
Meta Description: Learn how to iterate through lists and process data in SQL, despite the lack of explicit loop constructs. This guide explores various techniques, including cursors, recursive CTEs, and procedural extensions, offering solutions for different database systems and scenarios.
Understanding the Limitations and Alternatives
Traditional loops aren't directly supported in SQL because it's designed for set-based operations. Instead of processing data row by row, SQL optimizes for processing entire sets at once. This makes it significantly faster for large datasets. However, there are situations where iterative processing seems necessary. The following sections outline the most effective workarounds.
Method 1: Using Cursors (Least Efficient, But Sometimes Necessary)
Cursors provide a way to iterate through a result set row by row. They are generally considered the least efficient method, especially for large datasets, because they bypass the optimized set-based processing of SQL. However, they are sometimes necessary for complex logic that can't be easily expressed with set-based operations.
Example (Illustrative – syntax may vary slightly depending on your database system):
DECLARE @Value INT;
DECLARE @Cursor CURSOR;
SET @Cursor = CURSOR FOR
SELECT Value FROM MyList;
OPEN @Cursor;
FETCH NEXT FROM @Cursor INTO @Value;
WHILE @@FETCH_STATUS = 0
BEGIN
-- Process @Value here (e.g., update another table)
UPDATE AnotherTable SET SomeColumn = @Value WHERE SomeCondition;
FETCH NEXT FROM @Cursor INTO @Value;
END;
CLOSE @Cursor;
DEALLOCATE @Cursor;
Important Note: Cursors should be used sparingly due to their performance implications. Consider alternatives whenever possible.
Method 2: Recursive Common Table Expressions (CTE) (More Efficient for Hierarchical Data)
Recursive CTEs are a powerful technique for handling hierarchical or recursive data. While not a direct loop, they can be used to process a list of values iteratively by recursively calling themselves until a termination condition is met. This is often a more efficient solution than cursors.
Example (Illustrative – syntax may vary):
WITH RecursiveCTE AS (
-- Anchor member: Starting point
SELECT Value, 1 as Level FROM MyList WHERE SomeCondition
UNION ALL
-- Recursive member: Calls itself until no more rows match
SELECT MyList.Value, Level + 1
FROM MyList
INNER JOIN RecursiveCTE ON MyList.SomeKey = RecursiveCTE.SomeKey
WHERE RecursiveCTE.Level < 10 -- Termination Condition
)
SELECT * FROM RecursiveCTE;
Method 3: Using Procedural Extensions (Database-Specific)
Most database systems offer procedural extensions, such as stored procedures or functions, written in languages like PL/SQL (Oracle), T-SQL (SQL Server), or PL/pgSQL (PostgreSQL). These languages provide traditional loop constructs like FOR
loops, allowing for more explicit control over iterative processes. This approach is often more efficient than cursors for complex logic.
Example (Illustrative – T-SQL for SQL Server):
CREATE PROCEDURE ProcessList (@List VARCHAR(MAX))
AS
BEGIN
DECLARE @Value VARCHAR(100);
DECLARE @Pos INT;
SET @Pos = CHARINDEX(',', @List);
WHILE @Pos > 0
BEGIN
SET @Value = SUBSTRING(@List, 1, @Pos - 1);
-- Process @Value here
PRINT @Value; -- Example: Print the value
SET @List = SUBSTRING(@List, @Pos + 1, LEN(@List) - @Pos);
SET @Pos = CHARINDEX(',', @List);
END;
-- Process the last value
PRINT @List;
END;
Choosing the Right Approach
The best approach depends on your specific needs:
- Simple operations on a small list: Consider using
UNION ALL
orCASE
statements for efficient set-based processing. - Hierarchical or recursive data: Recursive CTEs are often the most efficient and elegant solution.
- Complex logic requiring explicit iteration: Use procedural extensions or, as a last resort, cursors. Always prioritize optimizing for set-based operations whenever possible for better performance.
- Large Datasets: Avoid cursors at all costs. Recursive CTEs or optimized set-based operations are preferred.
Remember to always profile your queries to determine the most efficient method for your specific data and workload. Prioritize set-based operations whenever possible for optimal performance with SQL.
Latest Posts
Latest Posts
-
Imagery Or Figurative Language From Romeo And Juliet
Jul 02, 2025
-
What Is A Quarter Of A Million
Jul 02, 2025
-
Which Of The Following Is True Concerning A Dao
Jul 02, 2025
-
How Long Can Catfish Live Out Of Water
Jul 02, 2025
-
Is Kanye West Related To Cornel West
Jul 02, 2025
Related Post
Thank you for visiting our website which covers about Sql Loop[ Thoug For Each Balue In The List . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.