Statsmodels Compute R2 Score On Test Set
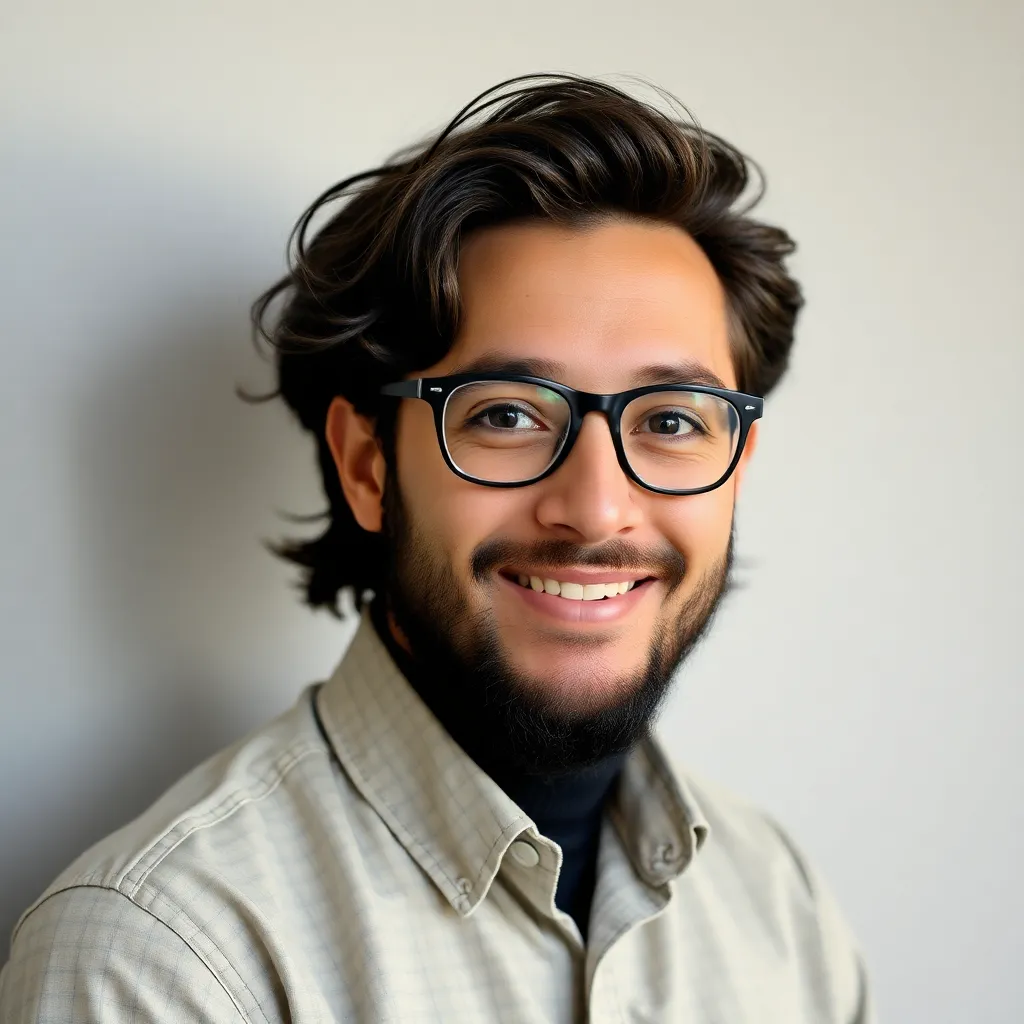
Kalali
May 23, 2025 · 4 min read
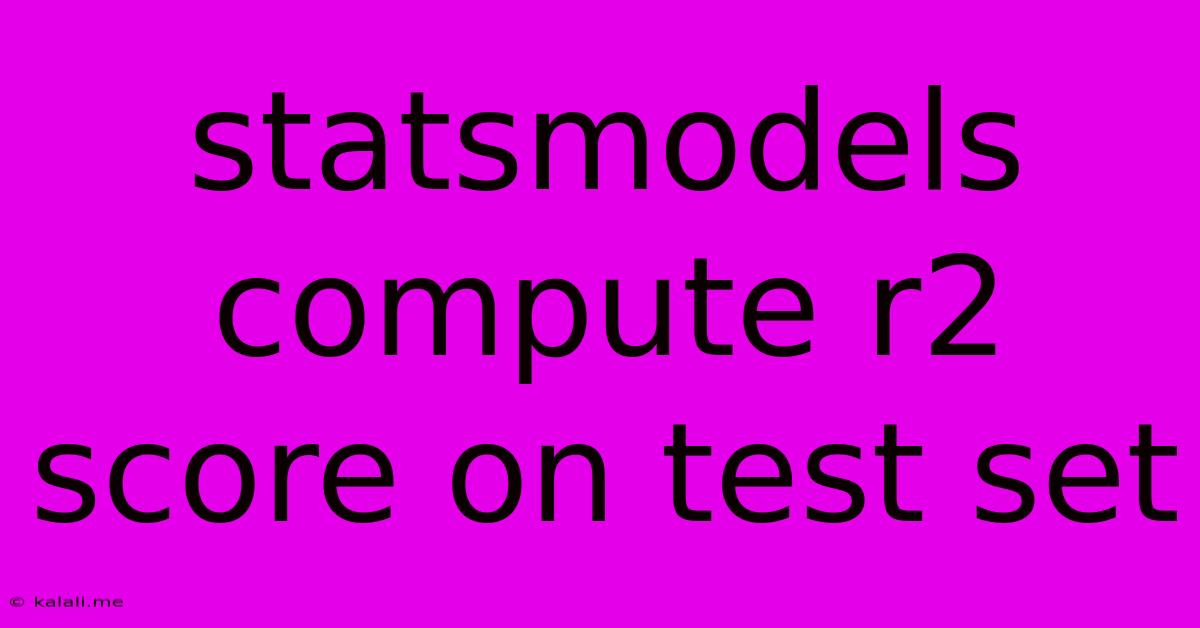
Table of Contents
Computing R-squared on a Test Set Using Statsmodels in Python
This article explains how to calculate the R-squared score on a test set using the Statsmodels library in Python. R-squared, or the coefficient of determination, is a crucial metric for evaluating the goodness of fit of a regression model. It represents the proportion of variance in the dependent variable that is predictable from the independent variables. While Statsmodels doesn't directly provide an R-squared function for test sets like scikit-learn, we can easily calculate it using the model's predictions and the true values. This guide will walk you through the process step-by-step.
Understanding R-squared and its Importance in Model Evaluation
Before diving into the code, let's briefly recap the significance of R-squared. A higher R-squared value (closer to 1) generally indicates a better fit, meaning the model explains a larger portion of the variability in the data. However, it's crucial to remember that a high R-squared doesn't necessarily imply a good model; overfitting can lead to high R-squared on training data but poor generalization to unseen data (like your test set). That's why evaluating the R-squared on a held-out test set is vital for assessing the model's predictive power.
Calculating R-squared on the Test Set with Statsmodels: A Practical Guide
We'll use a simple linear regression example to demonstrate the calculation. Assume you've already built your model using Statsmodels' OLS
(Ordinary Least Squares) function.
import numpy as np
import pandas as pd
import statsmodels.api as sm
from sklearn.model_selection import train_test_split
from sklearn.metrics import r2_score
# Sample Data (Replace with your actual data)
np.random.seed(42)
X = np.random.rand(100, 1) * 10
y = 2*X[:, 0] + 1 + np.random.randn(100)
# Add a constant to the independent variable for the intercept
X = sm.add_constant(X)
# Split data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Fit the model using Statsmodels
model = sm.OLS(y_train, X_train).fit()
# Make predictions on the test set
y_pred = model.predict(X_test)
# Calculate R-squared using scikit-learn's r2_score
r2 = r2_score(y_test, y_pred)
# Print the R-squared score
print(f"R-squared on the test set: {r2}")
# Accessing other Statsmodels metrics
print(model.summary())
Explanation:
- Import necessary libraries: We import
numpy
,pandas
,statsmodels
,train_test_split
, andr2_score
.train_test_split
is used for splitting data, andr2_score
(from scikit-learn) conveniently calculates the R-squared. - Generate or load data: Replace the sample data with your own dataset. Ensure your independent variables (X) are correctly formatted.
- Add a constant: Statsmodels requires a constant term for the intercept, so we use
sm.add_constant()
. - Split data: We split the data into training and testing sets using
train_test_split
. Adjusttest_size
as needed. - Fit the model: We fit the OLS model using the training data.
- Make predictions: We use
model.predict()
to get predictions on the test set. - Calculate R-squared: We use
r2_score
from scikit-learn to compute the R-squared score on the test set, comparing the predicted values (y_pred
) against the actual values (y_test
). - Print the results: The script prints the calculated R-squared value. The
model.summary()
provides a comprehensive summary of the model's statistics, including the R-squared on the training data.
Important Considerations:
- Data Preprocessing: Ensure your data is properly preprocessed before model fitting, including handling missing values and scaling features if necessary.
- Model Selection: The choice of regression model (linear, polynomial, etc.) depends on the nature of your data and the relationship between variables.
- Overfitting and Underfitting: A low R-squared on the test set might indicate underfitting, while a large discrepancy between training and test R-squared suggests overfitting. Consider using regularization techniques or different model structures to address these issues.
- Other Evaluation Metrics: While R-squared is a valuable metric, consider using other evaluation metrics like Adjusted R-squared, Mean Squared Error (MSE), and Root Mean Squared Error (RMSE) for a more comprehensive assessment.
This detailed guide enables you to effectively compute the R-squared score on a test set using Statsmodels, enhancing your model evaluation process and leading to more robust and reliable predictive models. Remember to always prioritize understanding your data and choosing the appropriate statistical methods.
Latest Posts
Latest Posts
-
How To Update Rhel Minor Version
May 23, 2025
-
How Do You Say It In Japanese
May 23, 2025
-
Componentprofiler Component Level Profiling Has Not Been Enabled Lwc
May 23, 2025
-
Toilet Keeps Running On And Off
May 23, 2025
-
How To Find The Area From Perimeter
May 23, 2025
Related Post
Thank you for visiting our website which covers about Statsmodels Compute R2 Score On Test Set . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.