Test For Broken Symbolic Link Perl
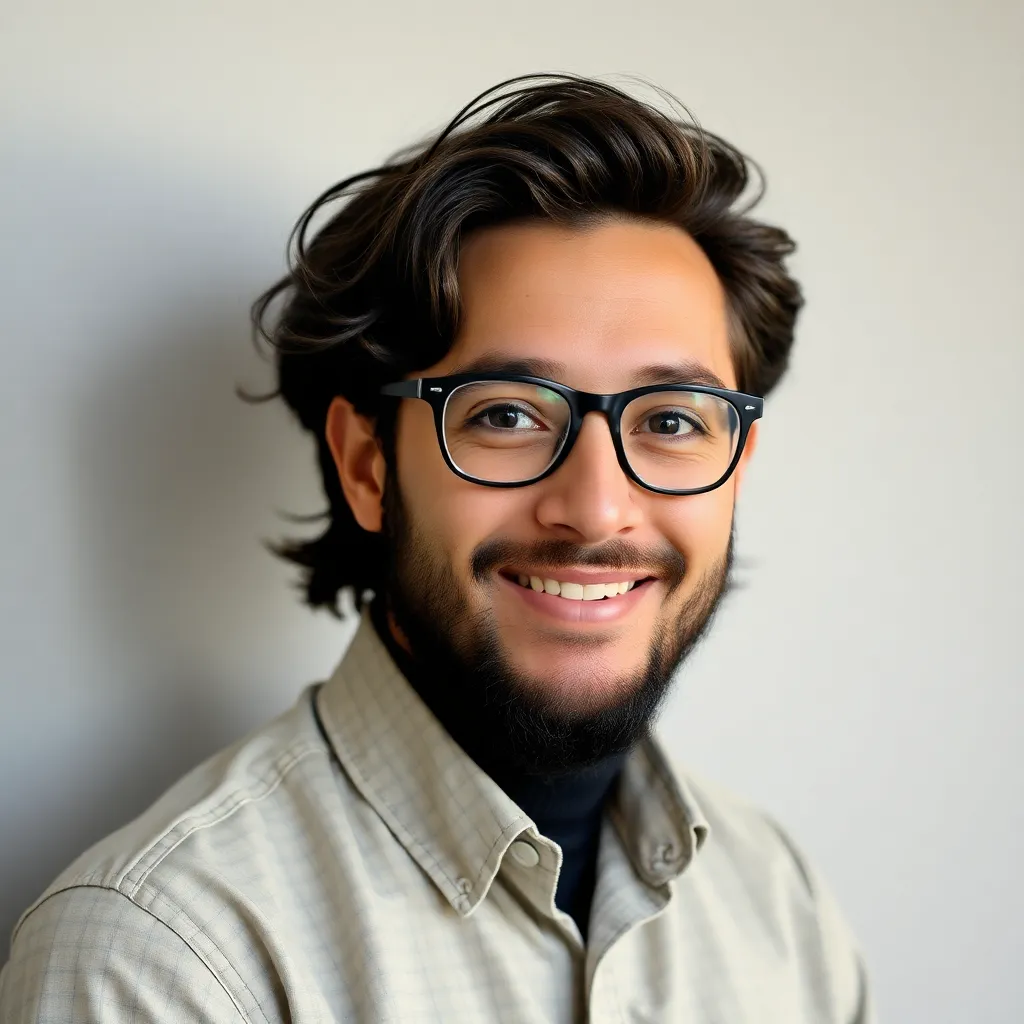
Kalali
May 23, 2025 · 3 min read
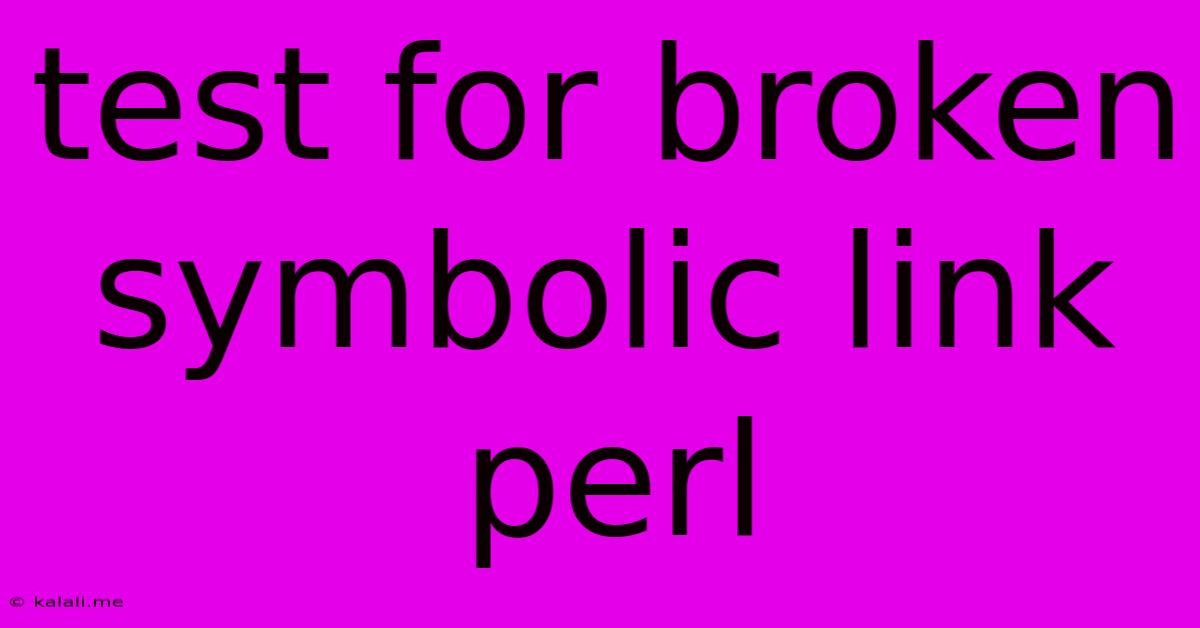
Table of Contents
Testing for Broken Symbolic Links in Perl
Finding and handling broken symbolic links is crucial for maintaining the integrity and reliability of your Perl scripts, especially when dealing with file system operations. A broken symbolic link, or dangling symbolic link, points to a target that no longer exists. This can lead to unexpected errors and program crashes. This article will guide you through various methods to effectively test for broken symbolic links in your Perl programs. We'll explore different approaches, emphasizing efficiency and readability.
Understanding Symbolic Links
Before diving into the testing methods, let's briefly review what symbolic links are. A symbolic link, or symlink, is a special type of file that acts as a pointer to another file or directory. When you access a symlink, the operating system follows the pointer to the actual target. If the target is missing, the link is considered "broken."
Methods for Detecting Broken Symbolic Links
Perl offers several ways to detect broken symbolic links. Here are the most common and reliable approaches:
1. Using -l
and -e
file test operators:
This is the most straightforward and widely used method. The -l
operator checks if a file is a symbolic link, and the -e
operator checks if a file or directory exists. By combining these, we can efficiently determine if a symlink is broken.
my $link = "/path/to/my/symlink";
if (-l $link) { # Check if it's a symbolic link
my $target = readlink($link); # Get the target path
if (! -e $target) { # Check if the target exists
print "$link is a broken symbolic link.\n";
} else {
print "$link is a valid symbolic link.\n";
}
} else {
print "$link is not a symbolic link.\n";
}
This code first verifies if the file is a symbolic link. If it is, it then uses readlink
to obtain the target path and checks if the target exists using -e
. This approach is concise and easy to understand.
2. Using readlink
and exception handling:
The readlink
function can also be used in conjunction with exception handling (using eval
) to gracefully manage potential errors. If readlink
encounters a broken symbolic link, it will typically throw an exception.
my $link = "/path/to/my/symlink";
eval {
my $target = readlink($link);
#Process the target if it exists.
};
if ($@) {
print "Error accessing $link: $@\n"; # Handle the exception
} else {
#Process successful readlink.
}
This method is advantageous because it can catch other potential errors besides broken symlinks that might occur during readlink
execution, offering more robust error handling.
3. Leveraging stat
for comprehensive information:
The stat
function provides detailed file information, including the type of file. While slightly more verbose, it allows you to check multiple file attributes simultaneously. This is particularly helpful if you need to perform other file checks alongside symlink verification.
use Fcntl qw(:seek);
my $link = "/path/to/my/symlink";
my $stat = stat($link);
if (defined $stat && $stat->type == 'link') {
my $target = readlink $link;
if (! -e $target) {
print "$link is a broken symbolic link\n";
} else {
print "$link is a valid symbolic link\n";
}
} else {
print "$link is not a symbolic link\n";
}
This example utilizes the stat
function to obtain file information and then uses readlink
for verification.
Choosing the Right Method
The best method depends on your specific needs and coding style. For simple checks, the combined use of -l
and -e
provides a clean and efficient solution. If you require more comprehensive error handling or need to check other file attributes, the eval
block with readlink
or the stat
function offer more robust options. Remember to always handle potential errors gracefully to avoid unexpected program termination. Using robust error handling enhances the reliability of your Perl scripts when dealing with the filesystem.
Latest Posts
Latest Posts
-
Where Is The 3 In Riddle Transfer
Jul 03, 2025
-
How Much Does A Water Bottle Weight
Jul 03, 2025
-
How Many Inches Is Half A Yard
Jul 03, 2025
-
How Old Are You If Your Born In 1996
Jul 03, 2025
-
How Many Water Bottles In 64 Ounces
Jul 03, 2025
Related Post
Thank you for visiting our website which covers about Test For Broken Symbolic Link Perl . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.