Test For Failed Symbolic Link Perl
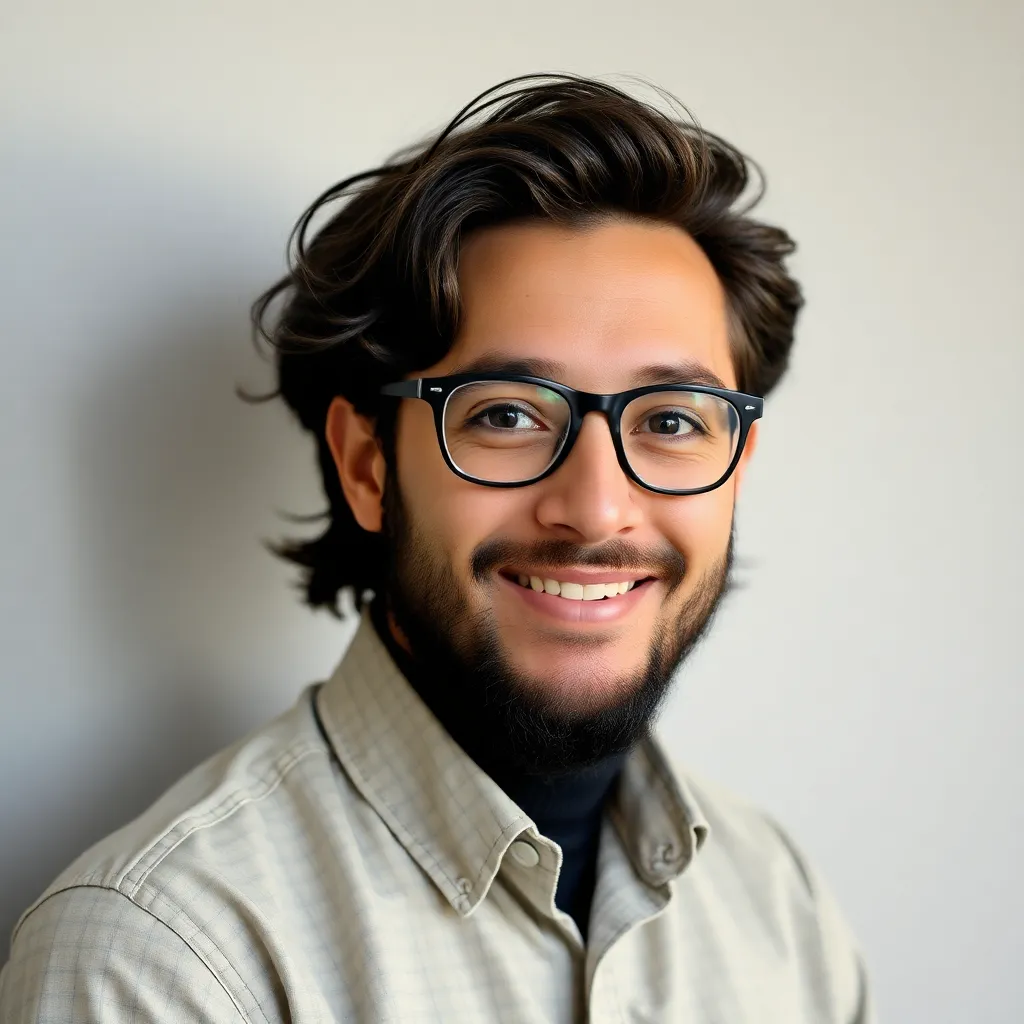
Kalali
May 26, 2025 · 3 min read
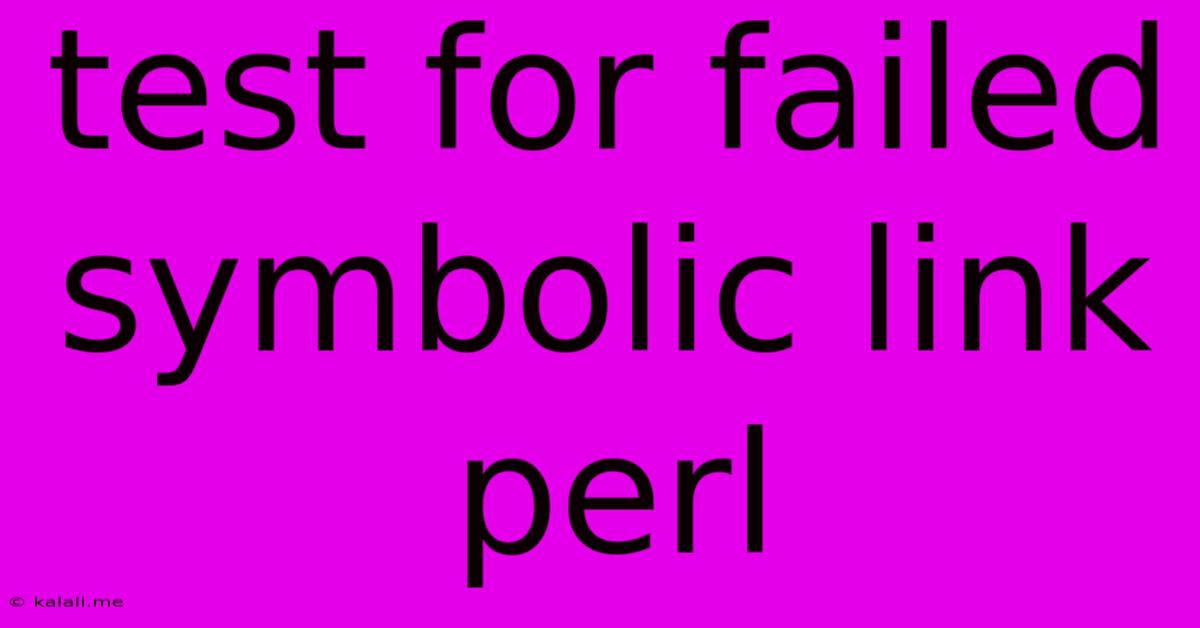
Table of Contents
Testing for Failed Symbolic Links in Perl
This article explores various methods for checking if a symbolic link creation in Perl has failed, focusing on robust error handling and best practices. Creating symbolic links is a common task, and understanding how to handle potential failures is crucial for writing reliable and resilient Perl scripts. We'll cover several approaches, from simple checks to more advanced techniques leveraging Perl's powerful file system capabilities.
Symbolic links, or symlinks, are pointers to other files or directories. If the target of a symlink doesn't exist, or there's a permissions issue preventing its creation, the symlink
function will fail. Ignoring these failures can lead to unexpected behavior and errors in your script. This article demonstrates several ways to detect and handle such failures gracefully.
Basic Error Handling with symlink
Perl's built-in symlink
function doesn't inherently return a boolean value indicating success or failure. Instead, it relies on Perl's exception handling mechanism. The simplest way to check for failure is to use eval
:
my $target = "/path/to/target";
my $link = "/path/to/link";
eval {
symlink($target, $link);
};
if ($@) {
print "Error creating symbolic link: $@\n";
# Handle the error appropriately, e.g., log the error, exit the script, etc.
} else {
print "Symbolic link created successfully.\n";
}
This eval
block captures any exceptions thrown during the symlink
operation. $@
will contain the error message if the symlink
call fails. This is a straightforward approach for detecting errors.
Checking for Link Existence and Target Validity
A more proactive approach involves verifying the link's existence and the target's validity after attempting to create the symlink:
my $target = "/path/to/target";
my $link = "/path/to/link";
symlink($target, $link) or die "Could not create symbolic link: $!";
if (-l $link) { #Check if it's a symbolic link
if (-e readlink($link)) { # Check if target exists
print "Symbolic link created successfully and target exists.\n";
} else {
print "Symbolic link created, but target '$target' does not exist.\n";
#Handle the situation where the link exists but target is missing
unlink $link; # Consider removing the broken symlink
}
} else {
print "Failed to create symbolic link: $!\n";
}
This code first attempts to create the symlink. If it fails, die
will terminate the script with an informative error message. If it succeeds, it then checks if the link exists (-l
) and if the target of the link exists (-e readlink($link)
). This provides more granular control and allows for specific error handling based on the cause of failure.
Using File::Spec
for Platform Independence
For better platform independence, use the File::Spec
module to handle path manipulations:
use File::Spec;
my $target = File::Spec->catfile("/path", "to", "target");
my $link = File::Spec->catfile("/path", "to", "link");
symlink($target, $link) or die "Could not create symbolic link: $!";
# ... rest of the validation code as above ...
File::Spec
ensures that path concatenation works correctly across different operating systems.
Advanced Error Handling and Logging
For production-level scripts, implementing comprehensive error logging is crucial. Consider using a logging module like Log::Log4perl
to record detailed error messages including timestamps, severity levels, and potentially even the backtrace for debugging purposes.
By combining the techniques presented here, you can create robust and reliable Perl scripts that handle symbolic link creation failures gracefully and informatively. Remember to choose the approach that best suits the complexity and requirements of your specific application. Prioritize clear error messages and appropriate error handling to ensure the stability and maintainability of your code.
Latest Posts
Latest Posts
-
How Many Cups In A Pound Of Hamburger Meat
Jul 02, 2025
-
Imagery Or Figurative Language From Romeo And Juliet
Jul 02, 2025
-
What Is A Quarter Of A Million
Jul 02, 2025
-
Which Of The Following Is True Concerning A Dao
Jul 02, 2025
-
How Long Can Catfish Live Out Of Water
Jul 02, 2025
Related Post
Thank you for visiting our website which covers about Test For Failed Symbolic Link Perl . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.