Test If A File Exists Bash
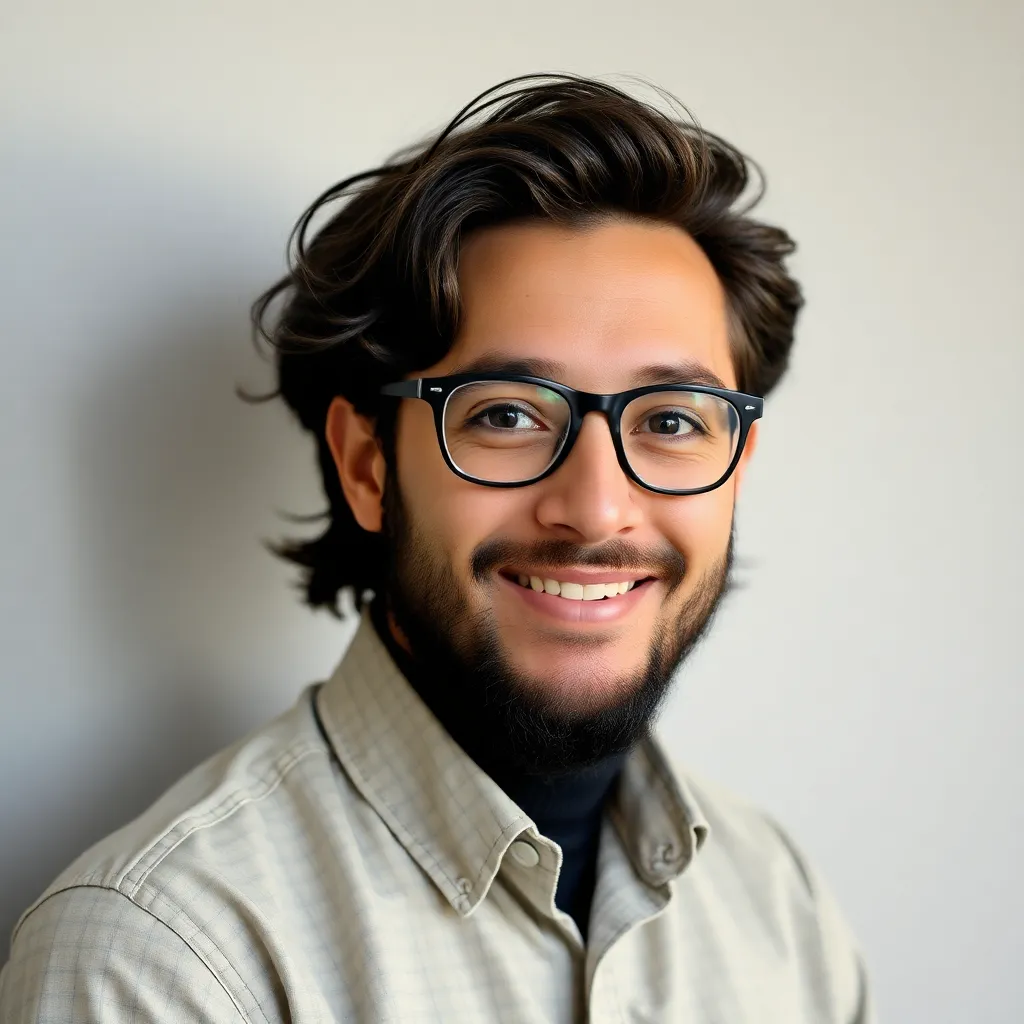
Kalali
May 20, 2025 · 3 min read
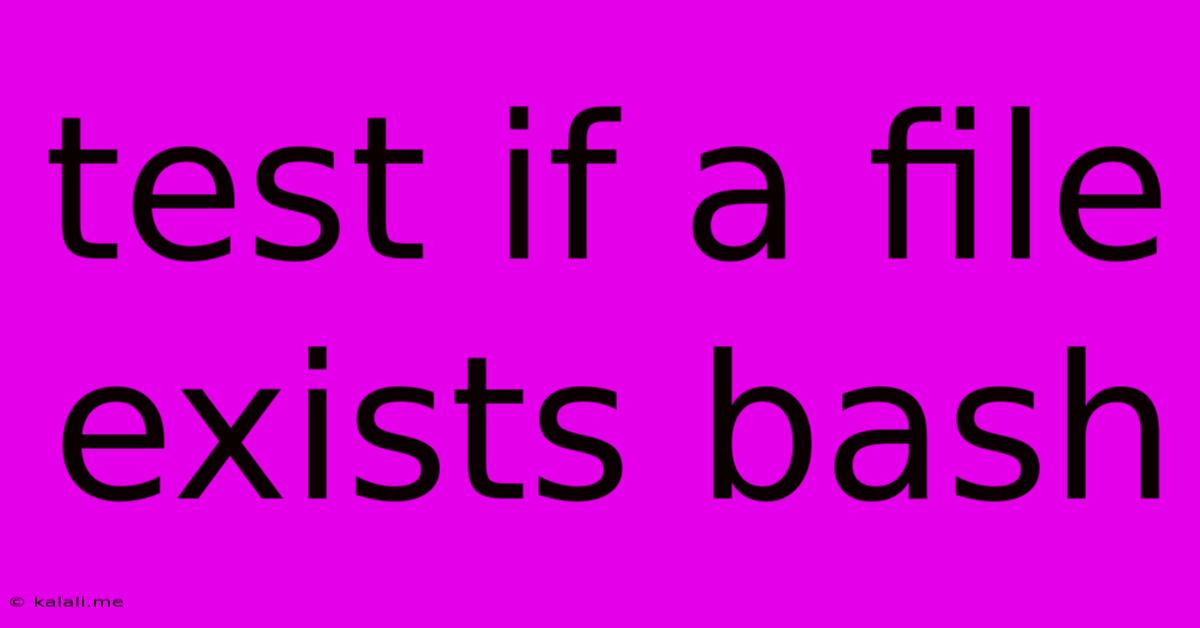
Table of Contents
How to Test if a File Exists in Bash: A Comprehensive Guide
This guide provides several methods to check for the existence of a file in a Bash script, covering various scenarios and levels of detail. Knowing how to reliably determine file existence is crucial for writing robust and error-free shell scripts. We'll explore different approaches, their strengths, and when to use them.
Why is checking file existence important? Before your script attempts to process a file, it's essential to verify its presence. Attempting operations on a non-existent file will typically lead to errors, halting your script's execution. This can be especially problematic in automated tasks or production environments.
Method 1: Using the -f
test operator
This is the most straightforward and commonly used method. The -f
operator checks if a given path is a regular file (not a directory, symbolic link, etc.).
if [ -f "/path/to/your/file.txt" ]; then
echo "File exists!"
else
echo "File does not exist."
fi
Replace /path/to/your/file.txt
with the actual path to the file you want to check. The square brackets [
are equivalent to the test
command. This method provides a clear and concise way to test file existence.
Method 2: Using the [[ ]]
test construct (Bash-specific)
Bash provides a more advanced test construct [[ ]]
that offers some advantages over the older [ ]
syntax. It handles word splitting and globbing differently, leading to more robust behavior.
if [[ -f "/path/to/your/file.txt" ]]; then
echo "File exists!"
else
echo "File does not exist."
fi
The functionality remains the same, but [[ ]]
is generally preferred for its improved handling of special characters and spaces in filenames.
Method 3: Checking the return code of a command
You can indirectly check file existence by attempting to access the file and examining the return code of the command. For instance, using the stat
command:
stat -t /path/to/your/file.txt &>/dev/null
if [ $? -eq 0 ]; then
echo "File exists!"
else
echo "File does not exist."
fi
The stat
command provides file information. &>/dev/null
redirects both standard output and standard error to /dev/null
, suppressing any output. The $?
variable holds the return code of the last executed command; 0 indicates success (file exists), while any other value indicates failure. This approach is less direct but can be useful in more complex scripts.
Handling Symbolic Links
If you need to check if a symbolic link exists and points to a valid file, use the -L
operator instead of -f
. The -L
operator verifies that the path is a symbolic link and that the target file exists. -e
will check if a file or directory exists, regardless of type.
if [[ -L "/path/to/your/symlink.txt" && -f "/path/to/your/symlink.txt" ]]; then
echo "Symbolic link exists and points to a file!"
fi
This combination ensures both the link's existence and the target file's existence.
Error Handling and Best Practices
Always include appropriate error handling in your scripts. If the file doesn't exist, your script should gracefully handle this situation rather than crashing. Consider using informative error messages to aid debugging.
Remember to always use absolute paths (starting with /
) to avoid ambiguity and potential errors caused by the script's current working directory. This makes your script more portable and reliable.
By employing these methods and best practices, you can ensure your Bash scripts effectively and reliably handle file existence checks, leading to more robust and maintainable code.
Latest Posts
Latest Posts
-
How Many Cups Is 1 Pound Of Cheese
Jul 12, 2025
-
30 X 30 Is How Many Square Feet
Jul 12, 2025
-
How Much Does A Half Oz Weigh
Jul 12, 2025
-
Calories In An Omelette With 3 Eggs
Jul 12, 2025
-
How Do You Say Great Grandmother In Spanish
Jul 12, 2025
Related Post
Thank you for visiting our website which covers about Test If A File Exists Bash . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.