Timber Twig Check If Something Is False
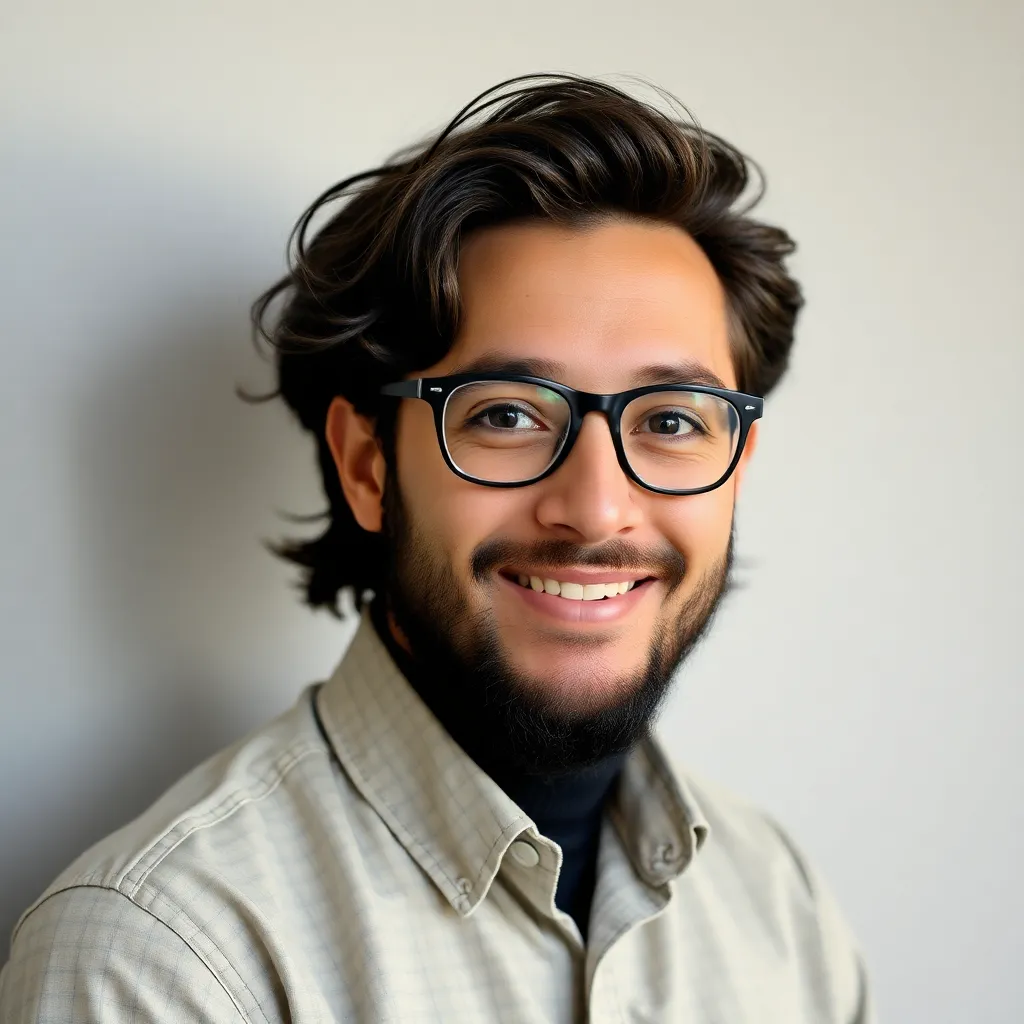
Kalali
May 24, 2025 · 3 min read
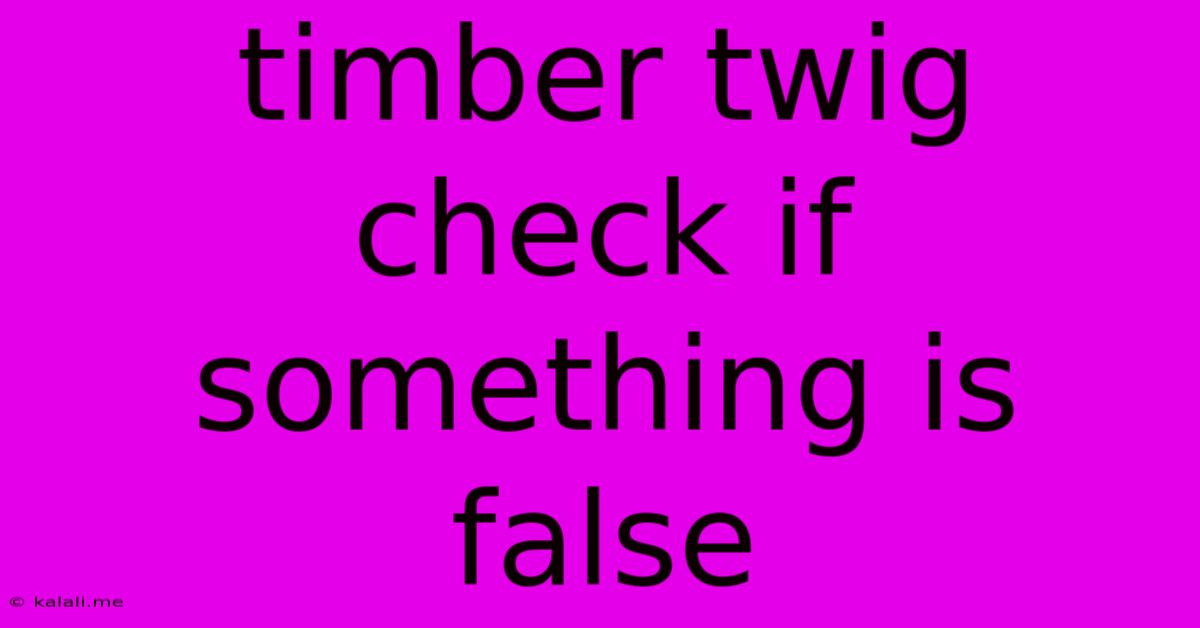
Table of Contents
Timber Twig: Checking for Falsehoods in Your Code
In the world of software development, robust error handling and validation are paramount. A single undetected false value can lead to cascading failures, unexpected behavior, and frustrating debugging sessions. While languages like Python offer built-in bool
types and truthy/falsy evaluations, sometimes you need a more explicit and visually clear way to check for falsehoods. This is where a technique inspired by the visual simplicity of a "timber twig"—easily broken if flawed—comes in handy. We'll explore how to implement this concept for clearer, more maintainable code. This article will cover different approaches to effectively identify and handle false conditions in your programming projects.
This article will focus on implementing a clear and concise method for checking for false values in your code, borrowing the visual metaphor of a "timber twig"—easily broken if flawed. We’ll explore this approach in several popular programming languages, highlighting best practices for readability and maintainability.
Understanding the "Timber Twig" Concept
The core idea behind the "timber twig" approach is to create a visual representation of a check for a false value. Imagine a twig—if it's flawed (false), it snaps. Similarly, our code should clearly indicate when a false condition is detected, ideally halting execution or triggering an appropriate response. This avoids subtle bugs that might only surface later in the application's lifecycle.
Implementing Timber Twig Checks in Different Languages
The specific implementation will differ slightly depending on your chosen language, but the underlying principle remains the same: a clear, explicit, and easily understandable check for a false value.
Python
Python's built-in boolean system is quite intuitive. However, we can enhance clarity with custom functions:
def check_timber_twig(value, message="Timber! False value detected!"):
"""Checks if a value is false and raises an exception if so."""
if not value:
raise ValueError(message)
return True
#Example Usage
result = check_timber_twig(False) # Raises ValueError
result = check_timber_twig(True) # Returns True
result = check_timber_twig(0) #Raises ValueError - 0 is considered falsy in Python
result = check_timber_twig("") #Raises ValueError - empty string is falsy
result = check_timber_twig(None) #Raises ValueError - None is falsy
try:
check_timber_twig(False)
except ValueError as e:
print(f"Caught exception: {e}")
This function leverages Python's inherent falsy values (0, None, False, empty strings, empty lists, etc.) and provides a customizable error message for improved debugging.
JavaScript
JavaScript's truthy/falsy system works similarly. We can create a function that mimics the Timber Twig approach:
function timberTwigCheck(value, message = "Timber! False value detected!") {
if (!value) {
throw new Error(message);
}
return true;
}
// Example Usage
try {
timberTwigCheck(false); // Throws an Error
timberTwigCheck(0); // Throws an Error - 0 is falsy
timberTwigCheck(""); // Throws an Error - empty string is falsy
timberTwigCheck(null); // Throws an Error - null is falsy
timberTwigCheck(true); // Returns true
} catch (error) {
console.error(error);
}
This JavaScript function mirrors the Python example, providing a clear and explicit check, and throwing an error for false values.
Best Practices for Timber Twig Implementation
- Clear Error Messages: Always include informative error messages that specify the context of the failure.
- Specific Checks: Avoid overly general checks. If possible, target specific false conditions relevant to your application logic.
- Logging: Integrate logging to record false condition occurrences, aiding in debugging and monitoring.
- Graceful Degradation: Consider implementing fallback mechanisms for scenarios where a false value is expected or can be gracefully handled.
By adopting the "timber twig" approach, you can improve your code's robustness, readability, and maintainability by making checks for false values more explicit and easier to identify, ultimately leading to more reliable and stable applications. Remember, the goal is not just to detect falsehoods but to do so in a way that clearly communicates the problem to developers for easier troubleshooting and prevention.
Latest Posts
Latest Posts
-
How Long Does Tuna Salad Keep In The Fridge
May 24, 2025
-
Testing Continuity With A Dmm Requires That
May 24, 2025
-
How Do You Say This In Japanese
May 24, 2025
-
How To Get Playdough Out Of Carpet
May 24, 2025
-
Multiplying Negative Time By A Positive Rate Results In A
May 24, 2025
Related Post
Thank you for visiting our website which covers about Timber Twig Check If Something Is False . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.