Unity 2d How To Freeze Player When Using Skills
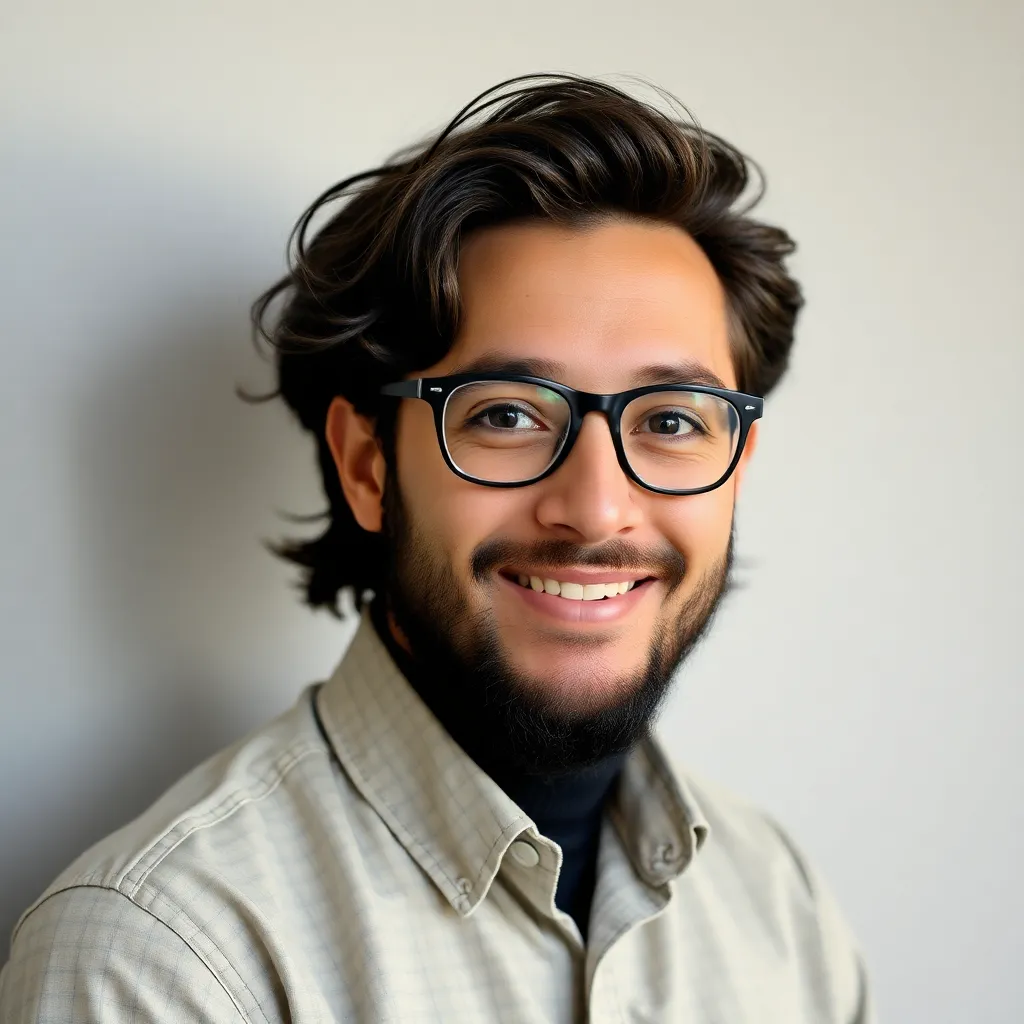
Kalali
May 23, 2025 · 3 min read
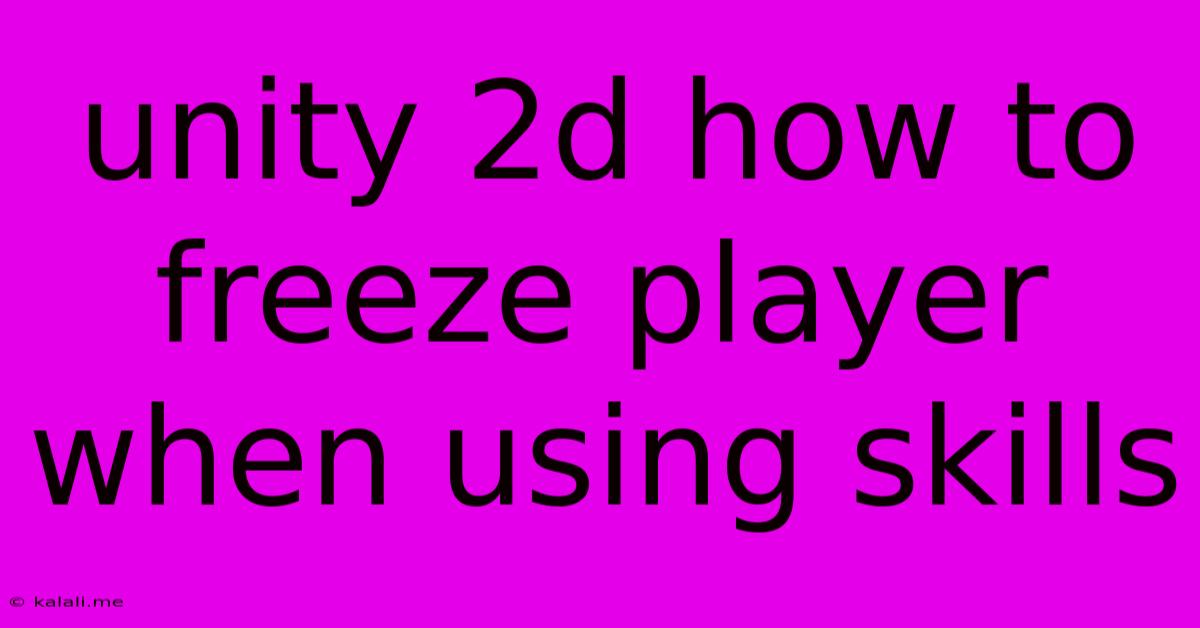
Table of Contents
Unity 2D: How to Freeze Player Movement During Skill Use
This article will guide you through several methods for freezing player movement in Unity 2D while using skills. This is a crucial aspect of many 2D games, ensuring smooth gameplay and preventing unintended actions during ability activation. We'll explore different approaches, comparing their advantages and disadvantages to help you choose the best solution for your project. This includes understanding the core mechanics, coding implementation, and best practices for a clean and efficient solution.
Understanding the Problem: Freezing player movement during skill use prevents the player from moving while their character is performing an action, such as attacking, casting a spell, or using a special ability. This is essential for preventing unintended movement that could break the game's logic or create frustrating gameplay experiences.
Method 1: Disabling the Rigidbody2D
This is a straightforward approach, particularly effective for physics-based movement. By temporarily disabling the Rigidbody2D
component, you effectively halt all physics-related movement.
Implementation:
-
Create a boolean variable: Add a boolean variable to your player script, for example,
isUsingSkill
. This variable will track whether the player is currently using a skill. -
Trigger the boolean: In your skill activation function, set
isUsingSkill
totrue
. -
Disable the Rigidbody2D: Add the following code within your
Update()
function:
if (isUsingSkill) {
GetComponent().simulated = false;
} else {
GetComponent().simulated = true;
}
- Re-enable the Rigidbody2D: After the skill animation or effect is complete, set
isUsingSkill
back tofalse
.
Advantages: Simple, efficient, and works well with physics-based movement.
Disadvantages: Abrupt halt in movement. May not be suitable for all movement types (e.g., purely transform-based movement).
Method 2: Setting Velocity to Zero
This method maintains the Rigidbody2D's simulation but directly manipulates its velocity. This can provide a smoother transition compared to abruptly disabling the Rigidbody2D.
Implementation:
-
Use the same
isUsingSkill
boolean as in Method 1. -
Modify your
Update()
function:
if (isUsingSkill) {
GetComponent().velocity = Vector2.zero;
}
- Re-enable movement by setting
isUsingSkill
tofalse
after skill completion.
Advantages: Smoother transition than disabling the Rigidbody2D.
Disadvantages: Still relies on Rigidbody2D. External forces might still subtly affect the player's position.
Method 3: Using Coroutines for Timed Freezing
This method offers more control and allows for precise timing of the freeze. Coroutines allow you to pause execution for a specific duration before resuming movement.
Implementation:
- Create a coroutine function:
IEnumerator FreezePlayer(float freezeDuration) {
isUsingSkill = true;
yield return new WaitForSeconds(freezeDuration);
isUsingSkill = false;
}
- Call the coroutine when a skill is activated:
StartCoroutine(FreezePlayer(1f)); // Freeze for 1 second
- Use the
isUsingSkill
variable to control movement as in previous methods.
Advantages: Precise control over freeze duration. Well-suited for animations and timed effects.
Disadvantages: Requires understanding of coroutines.
Choosing the Right Method
The best method depends on your game's specific needs and movement system. For simple physics-based movement, disabling the Rigidbody2D
(Method 1) might be sufficient. For smoother transitions, setting velocity to zero (Method 2) is preferable. For more complex scenarios with animations and timed effects, using coroutines (Method 3) provides the most flexibility. Remember to consider the overall feel and responsiveness you want to achieve in your game. Experiment with different approaches to find the optimal solution for your project. Remember to always test thoroughly to ensure smooth and intuitive gameplay.
Latest Posts
Latest Posts
-
Can Number Fields Be Replaced By Function Fields
May 23, 2025
-
Latex Tikz Homology On Double Torus
May 23, 2025
-
Magento 2 Minicart Not Showing Count
May 23, 2025
-
Word For Who Does What Job
May 23, 2025
-
Gears Of War 4 Trading Weapons
May 23, 2025
Related Post
Thank you for visiting our website which covers about Unity 2d How To Freeze Player When Using Skills . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.