Valueerror: A Linearring Requires At Least 4 Coordinates.
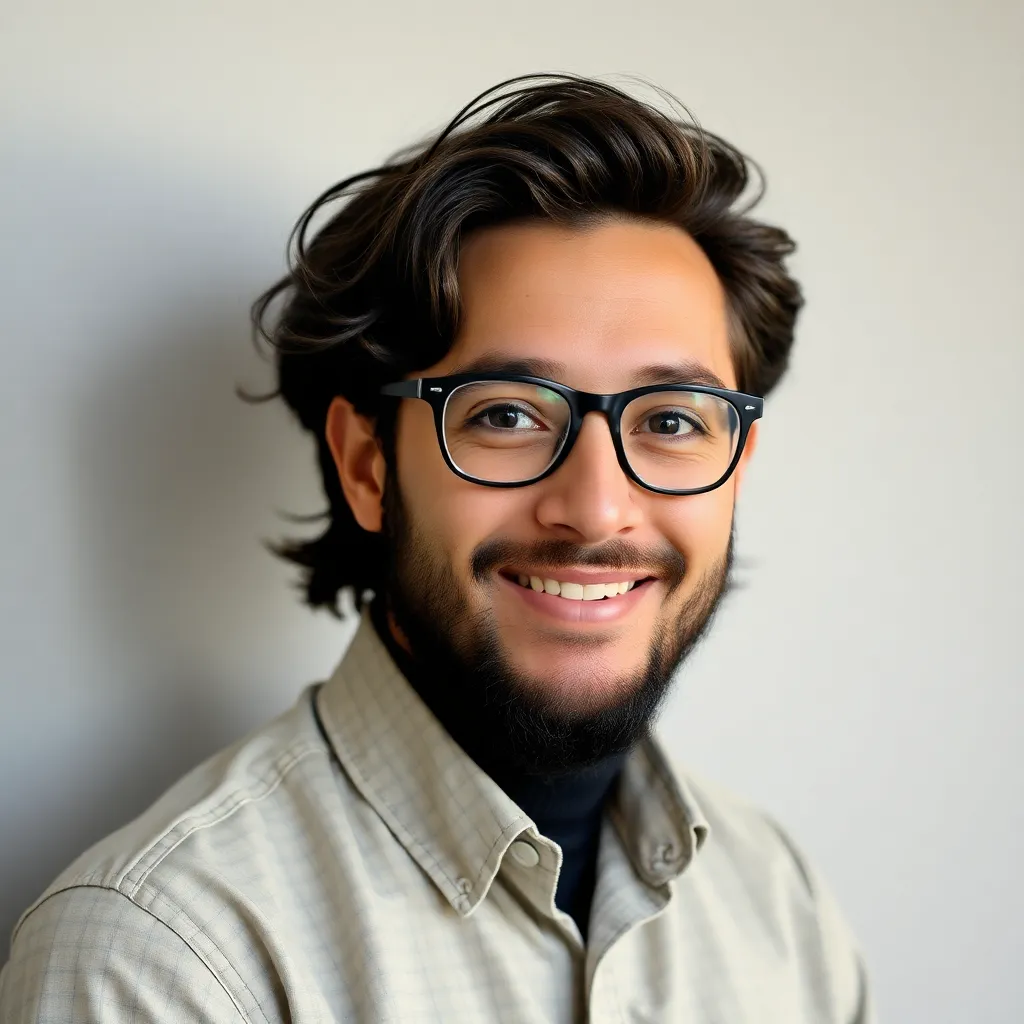
Kalali
May 23, 2025 · 4 min read
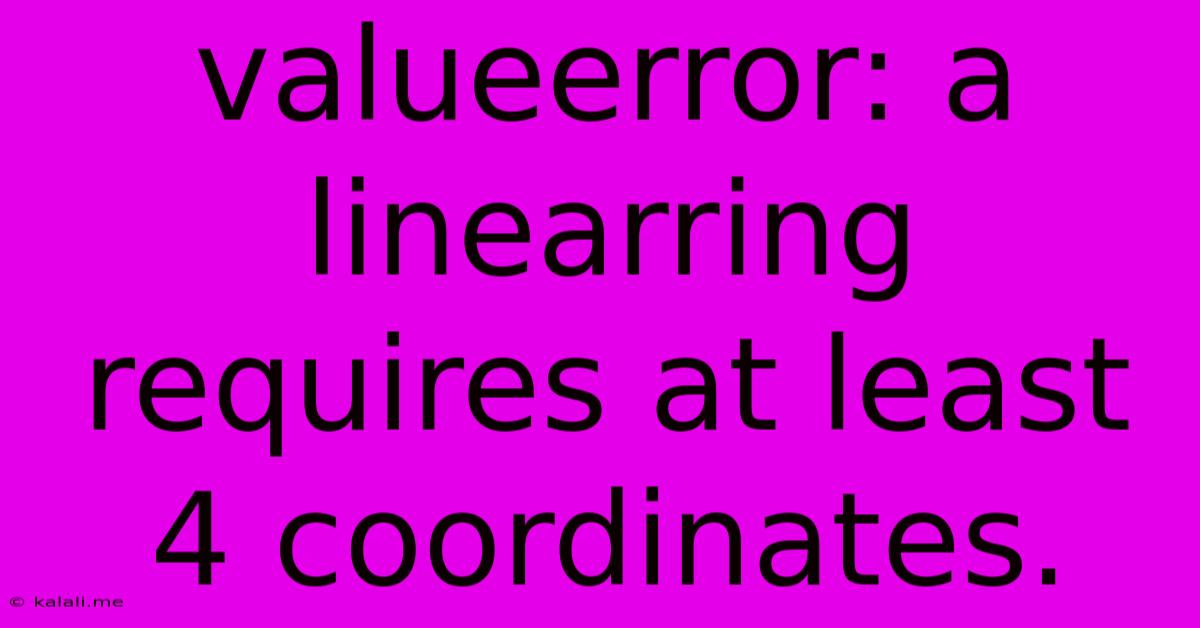
Table of Contents
ValueError: A LinearRing requires at least 4 coordinates
The error "ValueError: A LinearRing requires at least 4 coordinates" is a common issue encountered when working with spatial data and the Shapely library in Python. This error arises when you attempt to create a LinearRing
object using fewer than four coordinate pairs. This article will delve into the root cause of this error, explore common scenarios where it occurs, and provide practical solutions to resolve it. Understanding this error is crucial for anyone working with geographic information systems (GIS) or handling geometric shapes in Python.
Understanding LinearRings and Shapely
Shapely is a powerful Python library for manipulating planar geometric objects. A LinearRing
in Shapely represents a closed polygon, essentially a line that starts and ends at the same point. Crucially, a closed polygon needs at least three points to define a closed shape (a triangle). However, Shapely requires at least four coordinates to create a valid LinearRing
. This additional coordinate is likely a consequence of internal checks and optimization within the library related to efficiency and robustness of geometric operations. It's not about the minimum number of points to define a closed shape geometrically, but the minimum required for Shapely's internal processes.
Common Causes of the Error
The ValueError
most frequently arises from these scenarios:
-
Incorrect Number of Coordinates: This is the most straightforward cause. You might be providing a list or tuple containing fewer than four coordinate pairs (e.g., only two points for a line segment). Remember each coordinate pair represents a point (x, y) or (longitude, latitude).
-
Data Input Errors: Issues with data loading or preprocessing can lead to incomplete or malformed coordinate lists. This could involve missing data, incorrect data formats, or errors in data cleaning steps. Incorrect data parsing from external sources (like CSV files or databases) is a common culprit.
-
Logic Errors in Code: A more subtle problem could exist within your code's logic, where the coordinates are generated dynamically, and a condition results in fewer than four coordinates being passed to the
LinearRing
constructor. This may involve loops, conditional statements, or functions that manipulate coordinates. -
Empty or Invalid Geometries: Attempts to construct a
LinearRing
from an empty geometry or a geometry with invalid coordinates will invariably result in this error.
Troubleshooting and Solutions
Here's a systematic approach to debugging and fixing the error:
-
Verify Coordinate Count: The first step is to meticulously check the number of coordinate pairs you're supplying to the
LinearRing
constructor. Print the coordinates before creating theLinearRing
to confirm they are what you expect. -
Inspect Data Sources: If you're loading data from an external file, carefully examine the data format and ensure all coordinates are present and correctly parsed. Look for missing values, incorrect delimiters, or inconsistencies in the data structure.
-
Debug Code Logic: If the coordinates are generated programmatically, use a debugger to step through your code and inspect the values at each step. This will help you identify the point where the number of coordinates becomes insufficient.
-
Handle Edge Cases: In your code, implement error handling to explicitly check for cases with fewer than four coordinates. You can use a conditional statement to gracefully handle such situations, perhaps by:
- Raising a more descriptive error message
- Returning a default geometry (e.g.,
None
or an empty geometry) - Using a fallback mechanism (e.g., creating a
LineString
instead of aLinearRing
)
-
Data Validation and Cleaning: Before creating any Shapely objects, validate and clean your data to remove any invalid or missing coordinates. This includes checking for
NaN
values or extreme outliers.
Example illustrating a solution:
from shapely.geometry import LinearRing, Polygon
coordinates = [(0, 0), (1, 1), (1, 0)]
try:
ring = LinearRing(coordinates) # This will raise the ValueError
polygon = Polygon(ring)
print("Polygon created successfully:", polygon)
except ValueError as e:
print(f"Error: {e}")
if len(coordinates) < 4:
print("Not enough coordinates to create a LinearRing. Adding a duplicate point.")
coordinates.append(coordinates[0]) #Simple fix: Duplicate the first point
try:
ring = LinearRing(coordinates)
polygon = Polygon(ring)
print("Polygon created successfully after fix:", polygon)
except ValueError as e:
print(f"Error after fix: {e}")
By systematically following these steps, you can effectively diagnose and resolve the "ValueError: A LinearRing requires at least 4 coordinates" error and ensure your spatial data processing runs smoothly. Remember to always validate your input data and carefully review your code's logic to prevent this error from recurring.
Latest Posts
Latest Posts
-
How Did Jack Die Brokeback Mountain
May 24, 2025
-
Bounded Variation But Not Absolutely Continuous
May 24, 2025
-
Garage Door Goes Up But Not Down
May 24, 2025
-
Docker Compose Non String Key In Services Dokuwiki Environment 0
May 24, 2025
-
How To Abreviated Error In 3 4 Letters
May 24, 2025
Related Post
Thank you for visiting our website which covers about Valueerror: A Linearring Requires At Least 4 Coordinates. . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.