Valueerror Columns Must Be Same Length As Key
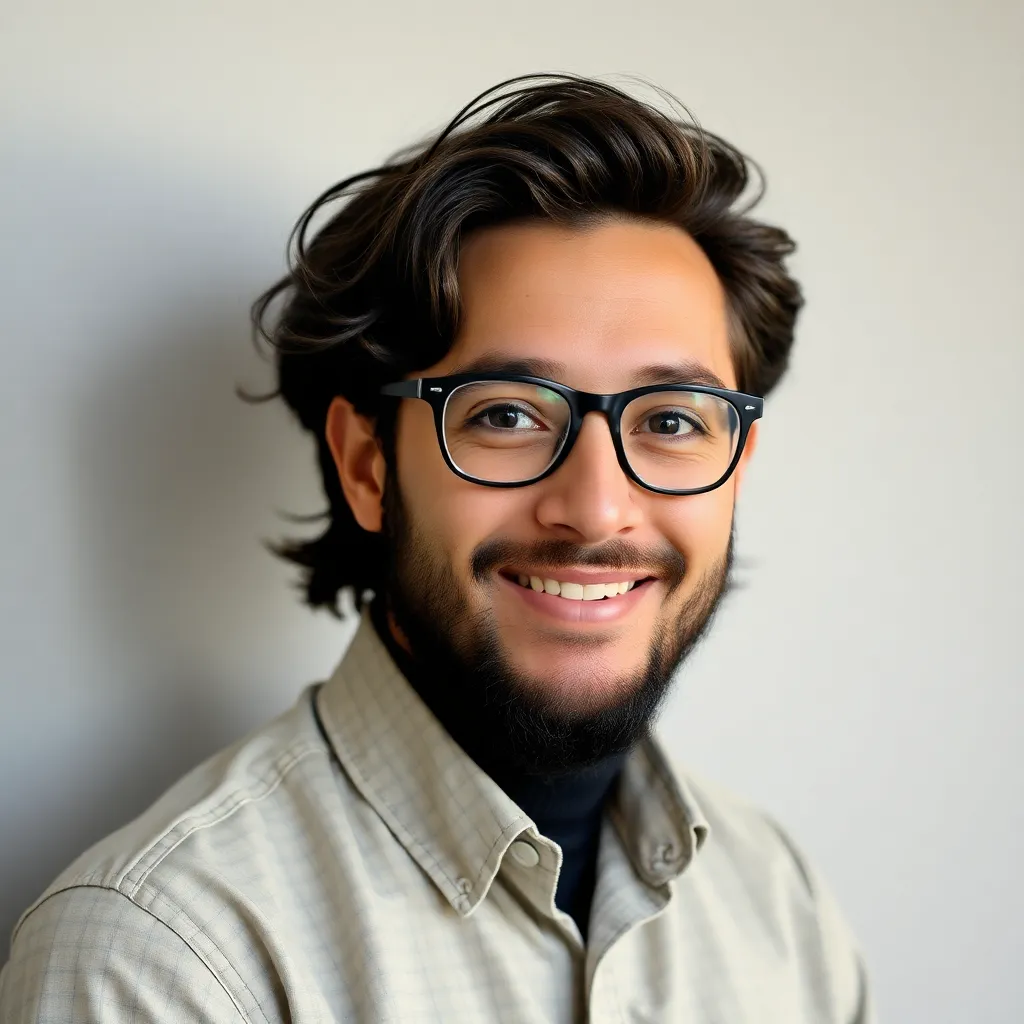
Kalali
May 25, 2025 · 3 min read
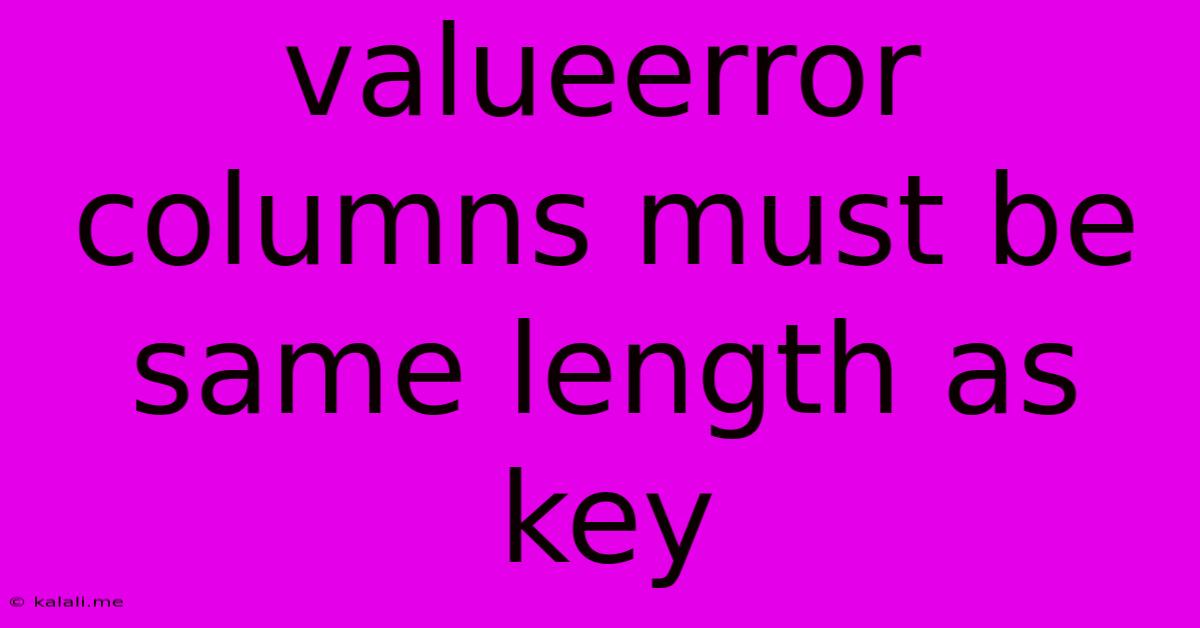
Table of Contents
Decoding the ValueError: Columns Must Be Same Length as Key
The dreaded ValueError: Columns must be same length as key
is a common error encountered when working with Pandas DataFrames in Python. This comprehensive guide will dissect the root causes of this error, providing clear explanations and practical solutions to help you troubleshoot and resolve it efficiently. This error typically arises when you're trying to create a DataFrame, assign data to columns, or manipulate existing columns using methods that require a consistent number of elements across all involved components.
This error message indicates a mismatch between the number of elements in your column data and the number of elements specified in your keys (column names). This often happens when you’re using dictionaries to create DataFrames or when you're performing operations that modify existing columns. Let's delve into the common scenarios and their fixes.
Understanding the Error
The core problem lies in the fundamental structure of a Pandas DataFrame. Each column represents a series of data, and these series must all have the same length. The error arises when you violate this rule, for example, by attempting to create a DataFrame where one column has more or fewer entries than another. The keys (column names) essentially define the structure, and the data must perfectly match this structure.
Common Causes and Solutions
Here are some common scenarios leading to this error, along with their solutions:
1. Mismatched Dictionary Lengths:
This is perhaps the most frequent cause. When creating a DataFrame from a dictionary, the lists or arrays assigned to each key (column name) must be of equal length.
- Incorrect:
data = {'col1': [1, 2, 3], 'col2': [4, 5]}
df = pd.DataFrame(data) #Raises ValueError
- Correct:
data = {'col1': [1, 2, 3], 'col2': [4, 5, 6]}
df = pd.DataFrame(data) # Works correctly
Solution: Ensure all lists or arrays within your dictionary have the same length. You might need to add placeholder values (like np.nan
for numerical data or an empty string for text data) to shorter lists to match the length of the longest list.
2. Assigning Data to Columns with Different Lengths:
Attempting to assign data to an existing column with a length different from the column's current length will also trigger this error.
- Incorrect:
df = pd.DataFrame({'col1': [1, 2, 3]})
df['col2'] = [4, 5] #Raises ValueError
- Correct:
df = pd.DataFrame({'col1': [1, 2, 3]})
df['col2'] = [4, 5, 6] # Works correctly
#Alternatively, using loc for precise assignment
df.loc[0:1, 'col2'] = [4,5]
df.loc[2,'col2'] = np.nan # Handle missing values
Solution: Before assigning new data, ensure it’s the same length as the existing columns. If you need to add data selectively, consider using .loc
or .iloc
for precise indexing to avoid length mismatches. Handle missing data gracefully using np.nan
.
3. Incorrect use of concat
or other DataFrame manipulation functions:
When concatenating or merging DataFrames, ensure that the columns being combined have compatible lengths or use appropriate options to handle mismatched lengths (like ignore_index=True
).
4. Errors in Data Cleaning or Preprocessing:
Inconsistent data cleaning steps might inadvertently lead to columns of differing lengths. Thoroughly review your data preparation stages, paying close attention to filtering, data imputation, or other transformations.
5. Inconsistent Data Loading:
If you're loading data from multiple sources (e.g., CSV files, databases), verify that all sources provide data with consistent column lengths. Data inconsistencies are a common source of errors.
Debugging Strategies
-
Print Data Shapes: Use
df.shape
to check the dimensions of your DataFrame and individual columns to quickly identify length discrepancies. -
Inspect Data: Carefully examine your data to spot missing values or any irregularities that might cause length mismatches.
-
Use Debugging Tools: Employ Python's debugging tools (like pdb) to step through your code and pinpoint the exact line where the error occurs.
By understanding the underlying causes and applying these solutions, you can effectively prevent and resolve the ValueError: Columns must be same length as key
error and ensure the smooth operation of your Pandas data manipulation tasks. Remember that careful data preparation and attention to detail are key to avoiding this frustrating issue.
Latest Posts
Latest Posts
-
What Is 1 2 Equivalent To In Fractions
Jul 06, 2025
-
How Do You Say Pork In Spanish
Jul 06, 2025
Related Post
Thank you for visiting our website which covers about Valueerror Columns Must Be Same Length As Key . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.