Valueerror: Setting An Array Element With A Sequence.
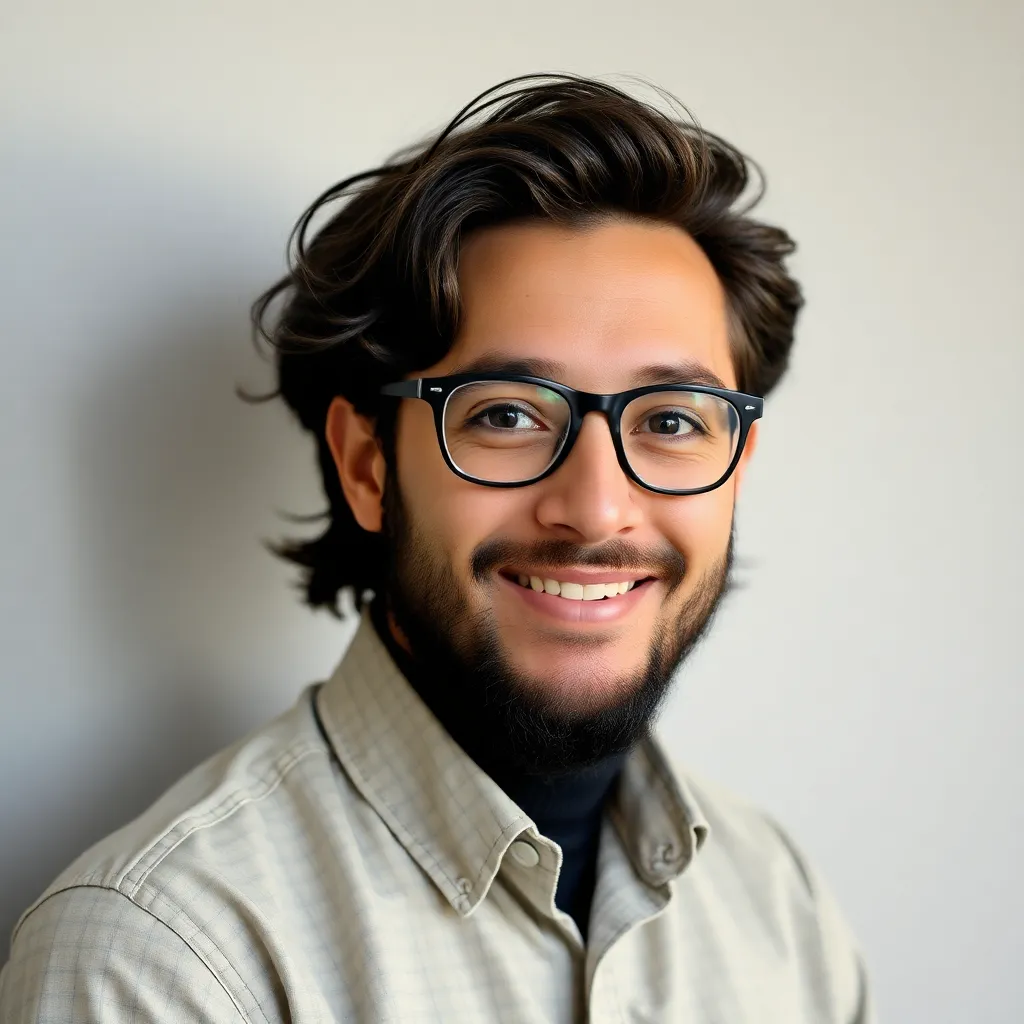
Kalali
May 21, 2025 · 4 min read
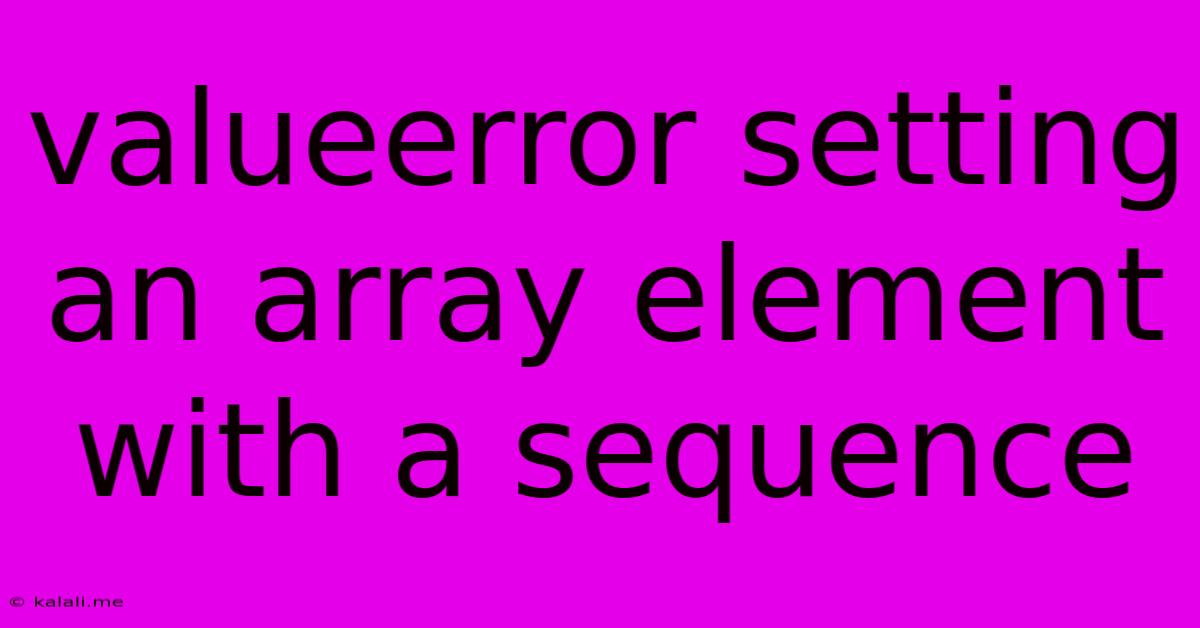
Table of Contents
ValueError: Setting an array element with a sequence – A Comprehensive Guide
The dreaded ValueError: setting an array element with a sequence
error in Python often leaves developers scratching their heads. This error typically arises when you try to assign a sequence (like a list or tuple) to a single element within a NumPy array that's expecting a scalar value. This article will dissect this error, explain its causes, and provide practical solutions to overcome it. Understanding this error is crucial for efficient data manipulation in Python, especially when working with numerical computation.
This error, ValueError: setting an array element with a sequence
, is common when working with NumPy arrays. It signifies an attempt to place a sequence (e.g., a list, tuple, or another array) into a single cell of an array designed for scalar values (single numbers, strings, etc.). NumPy arrays are designed for efficiency and consistency; each element within the array should be of the same data type.
Understanding the Root Cause
The fundamental problem lies in the mismatch between the expected data type of the array element and the data type you're trying to assign. NumPy arrays are homogeneous – all elements must be of the same data type. When you try to assign a sequence, NumPy interprets this as an attempt to place multiple values into a single location, resulting in the ValueError
.
Let's illustrate this with an example:
import numpy as np
my_array = np.array([1, 2, 3]) # A NumPy array of integers
try:
my_array[0] = [4, 5] # Attempting to assign a list to a single element
except ValueError as e:
print(f"Error: {e}")
This code will produce the ValueError: setting an array element with a sequence
because my_array[0]
expects a single integer, not a list.
Common Scenarios Leading to the Error
- Incorrect Data Type: Assigning a list or tuple to an array expecting integers or floats.
- Nested Arrays: Improperly handling nested arrays during array creation or manipulation.
- Data Loading Issues: Loading data from a file or database where the data structure doesn't match the array's expected shape or dtype.
- Inconsistent Data: Mixing scalar values and sequences within the same array during creation.
Effective Solutions and Workarounds
The solution depends on your intended outcome. Here are several strategies:
- Reshape the Array: If you intend to store the sequence as a sub-array, reshape your existing array or create a new one with the appropriate dimensions.
my_array = np.array([[1, 2, 3]]) # Reshape to a 2D array to accommodate the list
my_array[0] = [4, 5, 6] # This works!
print(my_array)
- Use
np.append
ornp.concatenate
: For adding elements to the end of an array, usenp.append
ornp.concatenate
. These functions are designed to handle adding elements to arrays, maintaining the data type consistency.
my_array = np.array([1, 2, 3])
my_array = np.append(my_array, [4, 5]) # Append a list
print(my_array)
my_array = np.concatenate((my_array, [6,7])) # Concatenate arrays
print(my_array)
-
Create a New Array with the Correct Shape: If you're dealing with a more complex data structure, it's often simpler to create a new NumPy array with the correct dimensions from the start. This avoids potential issues with data type mismatches. Utilize functions like
np.zeros
,np.ones
, ornp.empty
to initialize the array with the correct shape and data type. -
Data Preprocessing: Before assigning data to a NumPy array, thoroughly preprocess your data to ensure consistency in data types. This might involve converting lists or other sequences to appropriate scalar values.
-
Check
dtype
: Always check your array'sdtype
attribute (my_array.dtype
) to confirm the expected data type.
Best Practices to Prevent the Error
- Plan your array structure: Before populating your NumPy array, carefully plan the array’s shape and data type to match your data.
- Validate input data: Validate your input data before assigning it to the array to ensure it's of the correct type.
- Use appropriate NumPy functions: Utilize the functions designed for array manipulation (
np.append
,np.concatenate
,np.reshape
, etc.) instead of directly assigning sequences. - Debugging techniques: Use print statements or debuggers to inspect the data types of your arrays and variables to pinpoint the source of the error.
By understanding the root causes and applying the solutions outlined above, you can effectively overcome the ValueError: setting an array element with a sequence
error and write more robust and efficient NumPy code. Remember to carefully plan your array structures and preprocess your data to avoid this common pitfall.
Latest Posts
Latest Posts
-
When Performing A Self Rescue When Should You Swim To Shore
Jul 15, 2025
-
How Many Decaliters Are In A Liter
Jul 15, 2025
-
What Note Sits In The Middle Of The Grand Staff
Jul 15, 2025
-
Did Lynette Shave Her Head In Real Life
Jul 15, 2025
-
How Many Yards Are In 45 Feet
Jul 15, 2025
Related Post
Thank you for visiting our website which covers about Valueerror: Setting An Array Element With A Sequence. . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.