Which Value Is An Output Of The Function
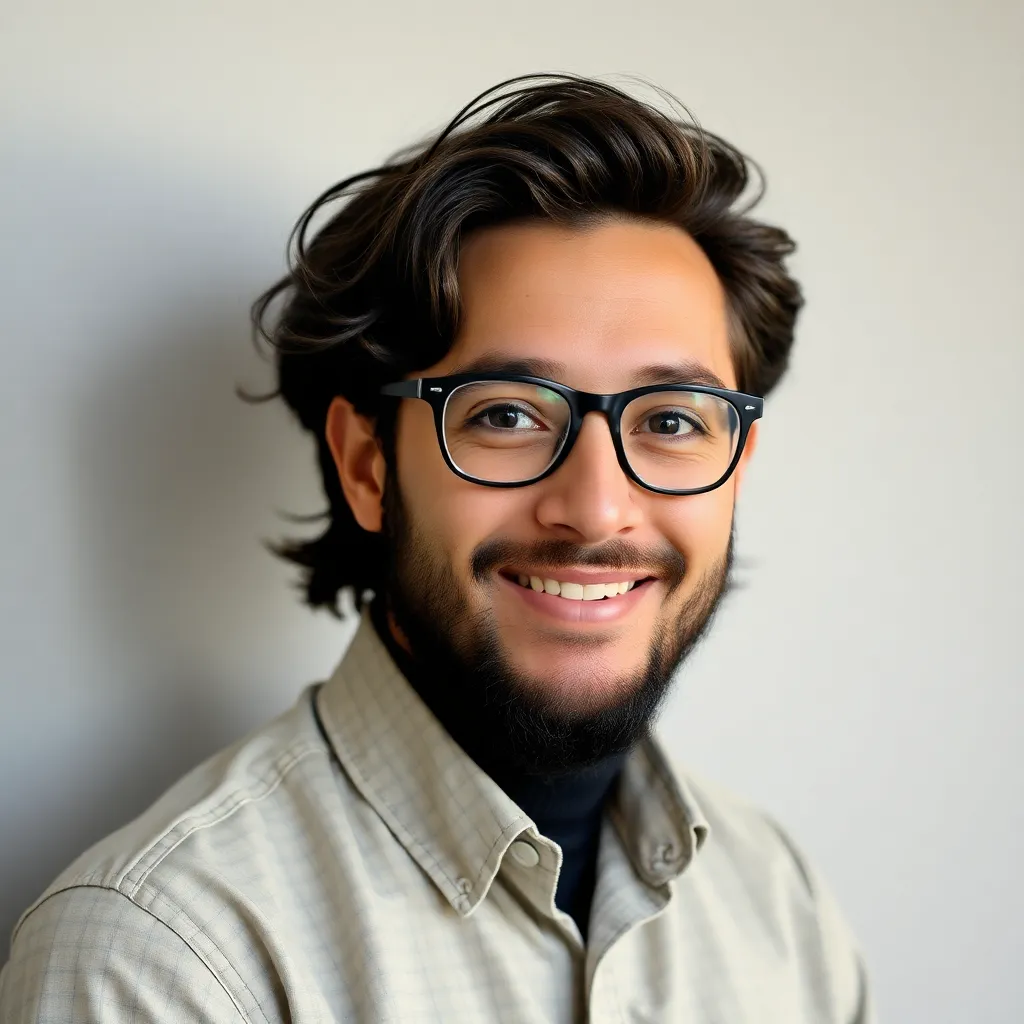
Kalali
Mar 30, 2025 · 5 min read
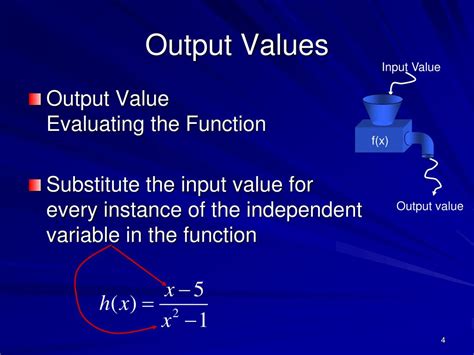
Table of Contents
Which Value is an Output of the Function? A Deep Dive into Function Outputs and Their Significance
Understanding function outputs is fundamental to programming and mathematics. A function, in its simplest form, takes an input, performs an operation, and produces an output. This seemingly straightforward process underpins complex algorithms and data manipulation. This comprehensive guide delves into the concept of function outputs, exploring various aspects, from basic definitions to advanced applications and troubleshooting common issues.
Understanding Functions and Their Outputs
A function is a self-contained block of code that performs a specific task. It accepts one or more inputs (arguments or parameters) and returns a single output value. The type of output depends entirely on the function's definition. The output can be a number, a string, a boolean value (true or false), a data structure (like a list or dictionary), or even another function (in the case of higher-order functions).
Key Components of a Function:
- Input: The data the function receives to perform its operation.
- Process: The set of instructions the function executes.
- Output: The result produced by the function after processing the input.
Example (Python):
def add_numbers(x, y):
"""This function adds two numbers and returns the sum."""
sum = x + y
return sum
result = add_numbers(5, 3) # result will be 8
print(result)
In this example, add_numbers
is the function name, x
and y
are the inputs, the +
operation is the process, and sum
is the output returned by the function. The return
statement is crucial; it specifies the value that the function sends back. Without a return
statement, the function implicitly returns None
.
Types of Function Outputs
The output of a function can vary widely depending on the function's purpose and design. Understanding these different types is crucial for effective programming.
Numerical Outputs
Many functions produce numerical outputs. These could represent calculations, measurements, or any other quantitative data.
Example (JavaScript):
function calculateArea(length, width) {
return length * width;
}
let area = calculateArea(10, 5); // area will be 50
console.log(area);
This JavaScript function calculates the area of a rectangle and returns a numerical value.
String Outputs
Functions can also produce text-based outputs. These are commonly used for displaying information, creating reports, or formatting data.
Example (C++):
#include
#include
std::string greet(std::string name) {
return "Hello, " + name + "!";
}
int main() {
std::string message = greet("Alice");
std::cout << message << std::endl; // Output: Hello, Alice!
return 0;
}
This C++ function takes a name as input and returns a greeting string.
Boolean Outputs
Boolean outputs represent true/false values. These are frequently used in conditional statements and logical operations.
Example (Java):
public class BooleanOutput {
public static boolean isEven(int number) {
return number % 2 == 0;
}
public static void main(String[] args) {
System.out.println(isEven(4)); // Output: true
System.out.println(isEven(7)); // Output: false
}
}
This Java function checks if a number is even and returns a boolean value.
Data Structures as Outputs
Functions can return more complex data structures, such as lists, arrays, dictionaries, or objects. This allows functions to manage and return multiple values simultaneously.
Example (Python):
def get_student_details(name, grades):
return {"name": name, "grades": grades}
student_data = get_student_details("Bob", [85, 92, 78])
print(student_data) # Output: {'name': 'Bob', 'grades': [85, 92, 78]}
This Python function returns a dictionary containing student information.
Void Functions and No Output
Some functions don't explicitly return a value. These are often called "void" functions (in languages like C++ and Java) or functions that implicitly return None
(in Python). Their primary purpose is to perform actions, such as modifying data or interacting with external systems. While they don't produce a direct output value, their actions indirectly affect the program's state.
Importance of Understanding Function Outputs
Grasping function outputs is critical for several reasons:
- Predictability: Knowing the expected output allows you to anticipate the behavior of your program.
- Debugging: When a function doesn't produce the expected output, understanding the function's logic helps pinpoint errors.
- Code Reusability: Well-defined functions with clear outputs make code modular and reusable.
- Data Manipulation: Functions are the backbone of data processing, and understanding their outputs is essential for managing data effectively.
- Program Flow Control: Function outputs are often used in conditional statements to control the program's execution path.
Troubleshooting Function Output Issues
When a function doesn't return the expected output, debugging is crucial. Here are some common causes and troubleshooting steps:
- Incorrect Input: Ensure the input values are correct and of the expected data type.
- Logic Errors: Carefully review the function's algorithm and logic to identify any flaws.
- Type Mismatches: Verify that the data types used within the function are consistent with the expected output type.
- Off-by-One Errors: Check for common indexing or boundary errors.
- Infinite Loops: If the function doesn't terminate, look for infinite loops in the code.
- Unhandled Exceptions: Use appropriate error handling mechanisms (like
try-except
blocks in Python) to manage potential exceptions.
Advanced Concepts Related to Function Outputs
Higher-Order Functions
Higher-order functions are functions that take other functions as inputs or return functions as outputs. These are powerful tools for creating flexible and reusable code.
Lambda Functions (Anonymous Functions)
Lambda functions are small, anonymous functions often used to create simple, one-line functions for use as arguments to other functions. Their output is determined by the expression they encapsulate.
Generators
Generators are a type of function that yields a sequence of values instead of returning a single value. Each yielded value is considered an output of the generator.
Recursion
Recursive functions call themselves within their definition. The output of a recursive function is the result of the base case(s) and the accumulation of results from recursive calls.
Conclusion
Understanding function outputs is paramount to successful programming. This guide has provided a comprehensive overview of function outputs, covering various types, their significance, troubleshooting techniques, and advanced concepts. By mastering these concepts, you can write more efficient, reliable, and maintainable code. Remember to always document your functions clearly, specifying the expected input types and output types to enhance code readability and facilitate collaboration. This detailed understanding will be invaluable in tackling increasingly complex programming challenges. Through diligent practice and a keen eye for detail, you can confidently master the art of working with function outputs and unlock their full potential in your programming endeavors.
Latest Posts
Latest Posts
-
What Is 1 2 Equivalent To In Fractions
Jul 06, 2025
-
How Do You Say Pork In Spanish
Jul 06, 2025
Related Post
Thank you for visiting our website which covers about Which Value Is An Output Of The Function . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.