Wordpress Plugin Check If Function Exists
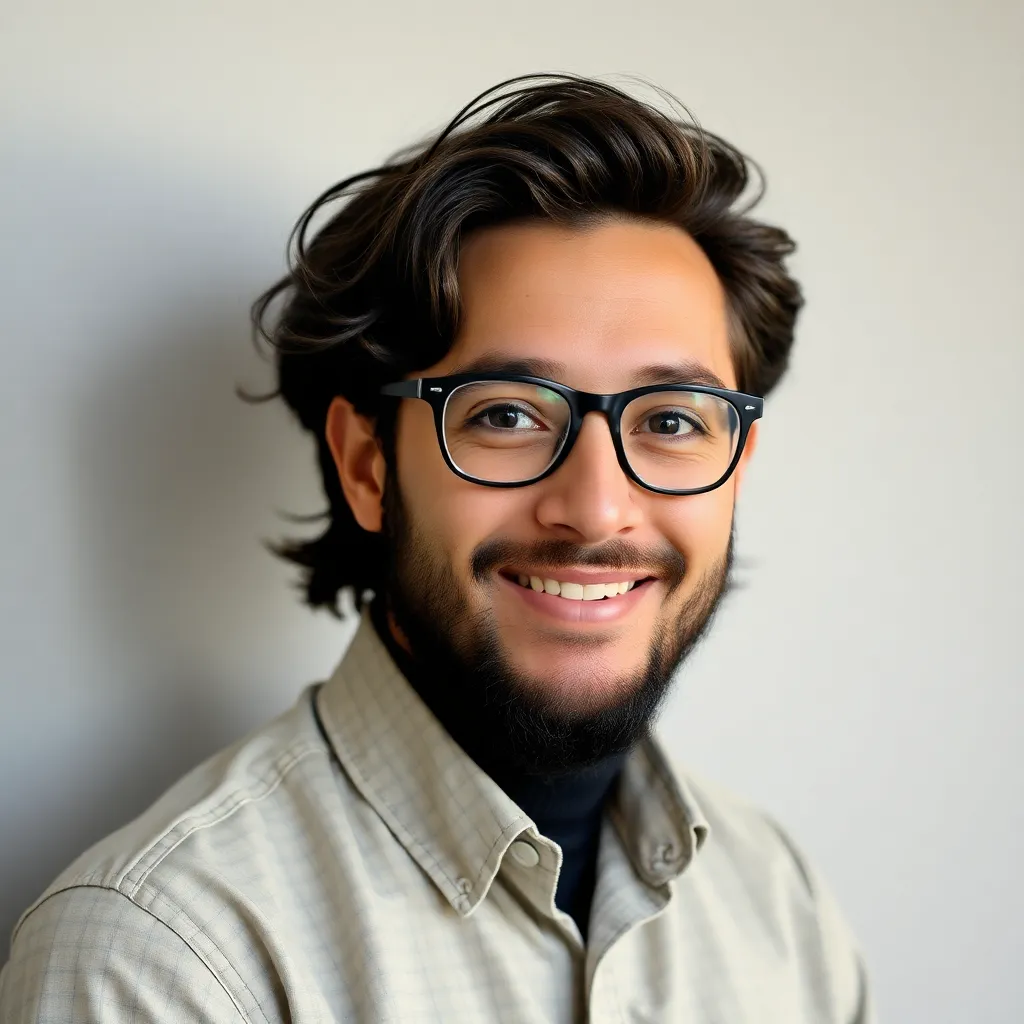
Kalali
May 23, 2025 · 3 min read
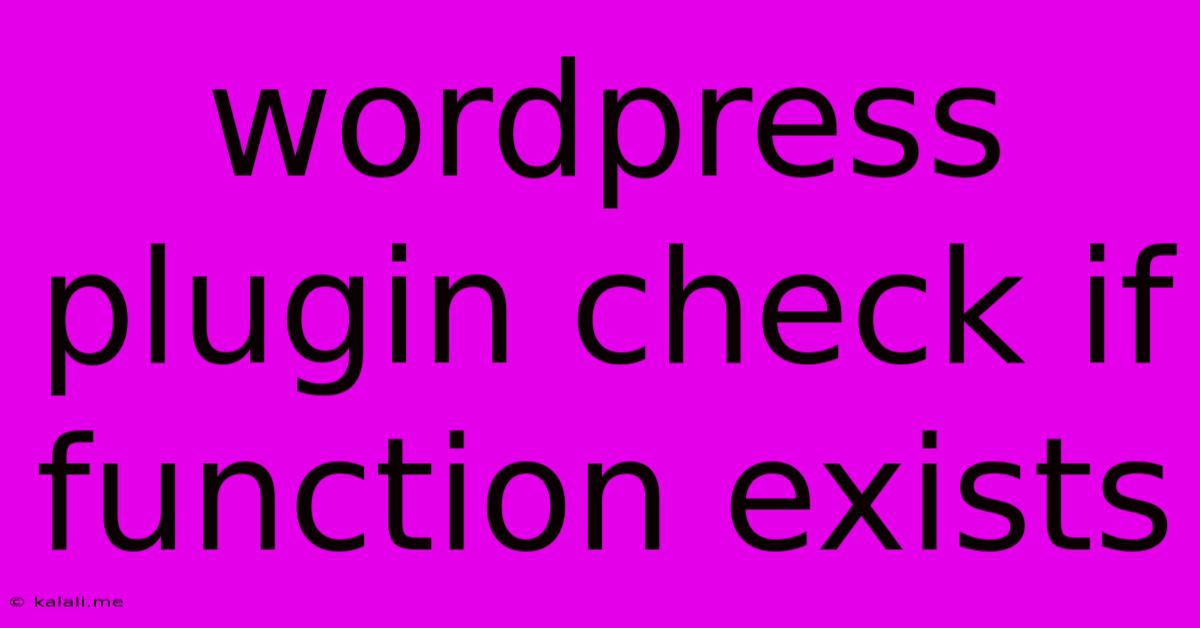
Table of Contents
Checking if a Function Exists in WordPress: A Guide to Avoiding Fatal Errors
WordPress plugins often rely on functions provided by other plugins or WordPress core. If a plugin tries to use a function that doesn't exist, it can lead to fatal errors, crashing your site. This article explores various methods to check if a function exists before using it within your WordPress plugins, ensuring smooth operation and preventing frustrating errors. This is crucial for writing robust and reliable WordPress plugins.
Why Checking for Function Existence is Crucial
Before diving into the methods, let's understand why checking for function existence is so important. Imagine your plugin depends on a function provided by another plugin. If that other plugin is deactivated or not installed, your plugin will fail to function correctly, potentially displaying a dreaded white screen of death or generating fatal error messages in your WordPress logs. This negatively impacts user experience and can even lead to site downtime. Properly checking for function existence prevents these issues.
Methods to Check for Function Existence in WordPress
WordPress offers several ways to gracefully handle the potential absence of a function:
1. function_exists()
This is the most common and recommended approach. The function_exists()
function is a built-in PHP function specifically designed for this purpose. It takes the function name as a string argument and returns true
if the function exists, and false
otherwise.
if ( function_exists( 'my_external_function' ) ) {
// Call the function if it exists
$result = my_external_function();
} else {
// Handle the case where the function doesn't exist
error_log( 'my_external_function() does not exist.' ); // Log the error for debugging
}
This example checks if my_external_function
exists before calling it. If the function is absent, an error is logged for debugging purposes. Replace my_external_function
with the actual function name you're checking. Remember to choose appropriate error handling based on your plugin's needs; you might display a notice to the admin or take other corrective actions.
2. is_callable()
The is_callable()
function offers a more comprehensive check. It not only checks if the function exists but also verifies if it's callable (meaning it's not a variable or something else that can't be called as a function).
if ( is_callable( 'my_external_function' ) ) {
// Call the function if it's callable
$result = my_external_function();
} else {
// Handle the case where the function is not callable
error_log( 'my_external_function() is not callable.' );
}
This is generally preferred if you need to ensure the function is truly executable.
3. Using a Plugin API (if applicable)
Some WordPress plugins expose their functionalities through an API. Instead of directly checking for a specific function, check if the API object or class exists and then use its methods. This is a more structured approach. This enhances modularity and reduces direct dependencies on specific function names.
Best Practices for Handling Missing Functions
- Graceful Degradation: Design your plugin to gracefully degrade if a dependent function is missing. Provide alternative functionality or a clear message to the user.
- Logging: Log errors to the WordPress debug log using
error_log()
. This is essential for debugging and identifying issues. - Conditional Logic: Use conditional statements (
if
,else
,elseif
) to handle different scenarios, based on whether functions are available. - Clear Error Messages: If a function is missing, provide informative messages to the administrator or user, explaining what's happening and possibly suggesting solutions (e.g., "Please install and activate Plugin X").
By implementing these checks and best practices, you significantly improve the robustness and reliability of your WordPress plugins, ensuring a positive experience for your users and preventing unexpected crashes or errors. Remember to always test your plugin thoroughly after implementing these checks.
Latest Posts
Latest Posts
-
How Much Is One Box Powdered Sugar
May 23, 2025
-
Was Paul A Member Of The Sanhedrin
May 23, 2025
-
Inner Measure Is Sup Of Closed Sets
May 23, 2025
-
Dragon Age Inquisition Hissing Wastes Key Fragments
May 23, 2025
-
Statute Of Limitations For Computer Misuse
May 23, 2025
Related Post
Thank you for visiting our website which covers about Wordpress Plugin Check If Function Exists . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.