Bash Read File Line By Line
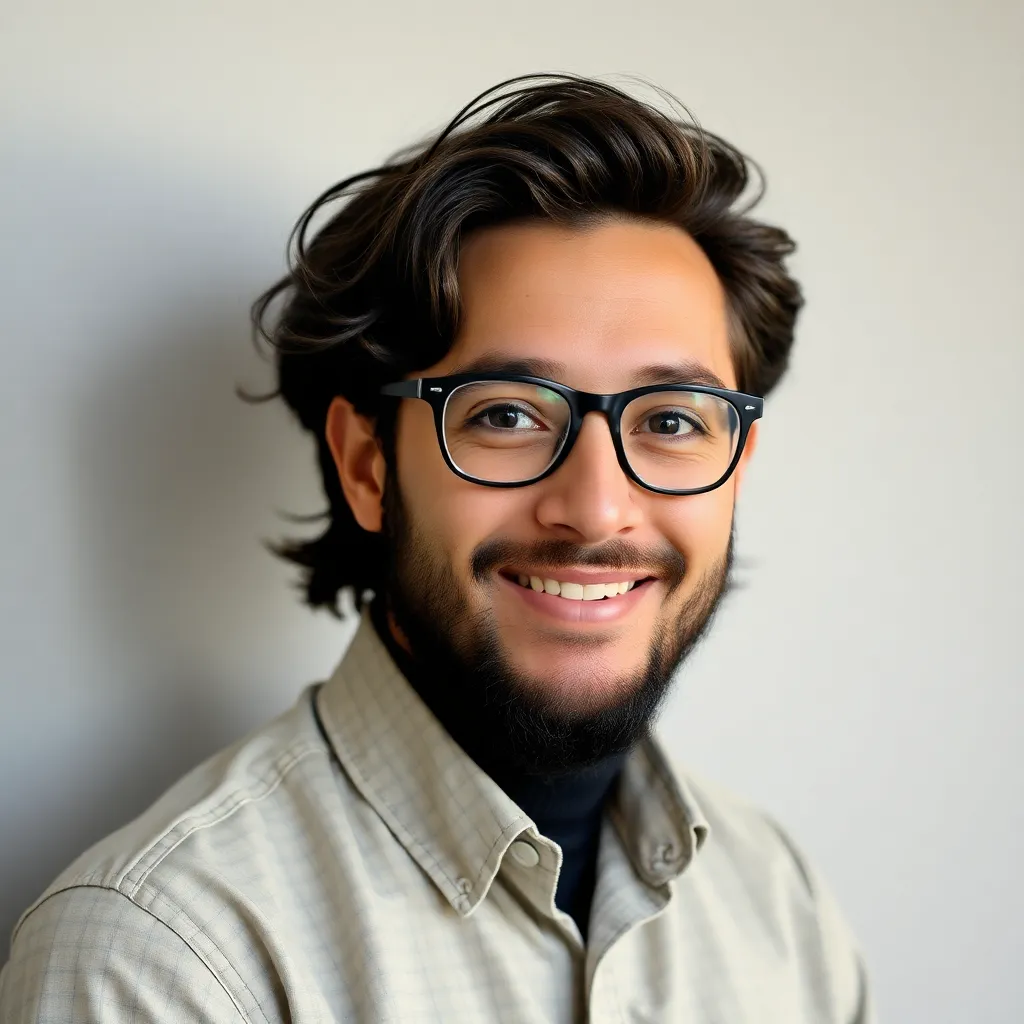
Kalali
May 19, 2025 · 3 min read
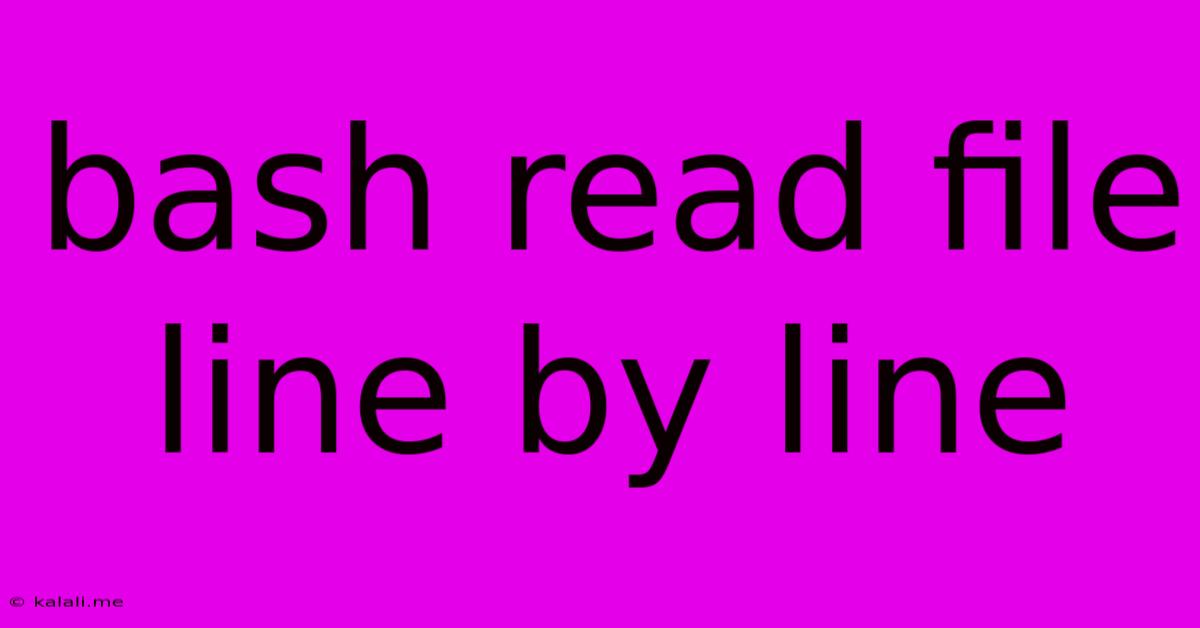
Table of Contents
Bash Read File Line by Line: A Comprehensive Guide
Reading files line by line is a fundamental task in any scripting language, and Bash is no exception. This guide will walk you through several effective methods for achieving this in Bash, covering different scenarios and best practices. Understanding how to efficiently process files line by line is crucial for automating tasks, data processing, and system administration. This article will explore various techniques, from basic loops to more advanced approaches using while
loops and read
with input redirection.
Why Read Files Line by Line in Bash?
Processing files line by line offers several advantages:
- Memory Efficiency: Reading an entire file into memory at once can be problematic for large files, potentially leading to crashes or slow performance. Line-by-line processing is significantly more memory-efficient.
- Flexibility: It allows for individual processing of each line, enabling more nuanced control over data manipulation and filtering.
- Error Handling: Easier to handle errors associated with specific lines, allowing the script to continue processing even if a problem occurs on a single line.
- Specific Data Extraction: Simplifies the task of extracting specific information from files based on patterns or criteria within individual lines.
Method 1: Using while
loop with read
This is arguably the most common and efficient method for reading files line by line in Bash. The while
loop continues as long as the read
command successfully reads a line.
#!/bin/bash
while IFS= read -r line; do
# Process each line here
echo "Processing line: $line"
done < "my_file.txt"
IFS=
: Prevents leading/trailing whitespace from affecting the line.-r
: Prevents backslash escapes from being interpreted. This is crucial for handling files with special characters.< "my_file.txt"
: Redirects the contents ofmy_file.txt
to thewhile
loop's input. Replace"my_file.txt"
with your actual file name.
Method 2: Using while
loop with read
and error handling
This enhances the previous method by incorporating error handling. It checks the return status of read
to gracefully handle cases where the file might not exist or is empty.
#!/bin/bash
while IFS= read -r line || [[ -n "$line" ]]; do
# Process each line here
echo "Processing line: $line"
done < "my_file.txt" || echo "Error reading file: my_file.txt"
|| [[ -n "$line" ]]
: This condition ensures the loop continues even ifread
fails and there's still data in theline
variable (this handles the last line without a newline).|| echo "Error reading file: my_file.txt"
: Prints an error message if the file reading process encounters an issue.
Method 3: Handling empty lines
Sometimes, you might need to specifically handle empty lines within your file. This modified version addresses that:
#!/bin/bash
while IFS= read -r line; do
# Check for empty lines
if [[ -z "$line" ]]; then
echo "Empty line encountered."
else
# Process non-empty lines
echo "Processing line: $line"
fi
done < "my_file.txt"
if [[ -z "$line" ]]
: This condition checks if the line is empty.
Choosing the Right Method
The best method depends on your specific needs. For simple tasks, Method 1 suffices. For robustness and error handling, Method 2 is recommended. If empty lines require special attention, Method 3 is the best choice. Remember to always replace "my_file.txt"
with the actual path to your file.
This guide provides a thorough understanding of reading files line by line in Bash, equipping you with the necessary knowledge for various scripting tasks. By understanding these techniques, you'll be able to efficiently and effectively process data from files within your Bash scripts. Remember to always prioritize error handling and efficient memory management when working with large files.
Latest Posts
Latest Posts
-
Red And Black Wiring Light Switch
May 19, 2025
-
How To Light A Candle Without Lighter
May 19, 2025
-
Rear Sub Frame Corroded But Not Seriously Weakened
May 19, 2025
-
Set Google As My Homepage On Iphone
May 19, 2025
-
1 Litre Of Water Weight In Kg
May 19, 2025
Related Post
Thank you for visiting our website which covers about Bash Read File Line By Line . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.