Google Sheets Script If Variable Equals Any Of A List
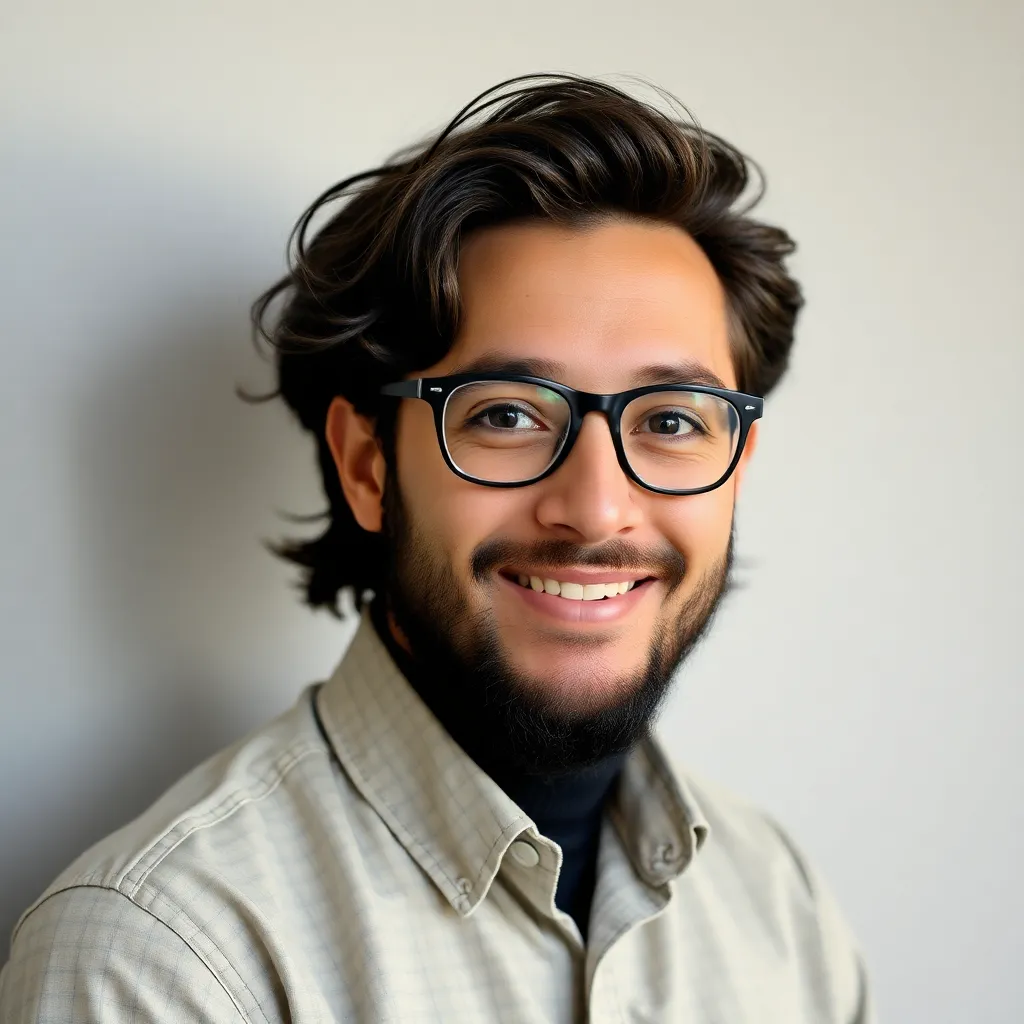
Kalali
May 23, 2025 · 3 min read
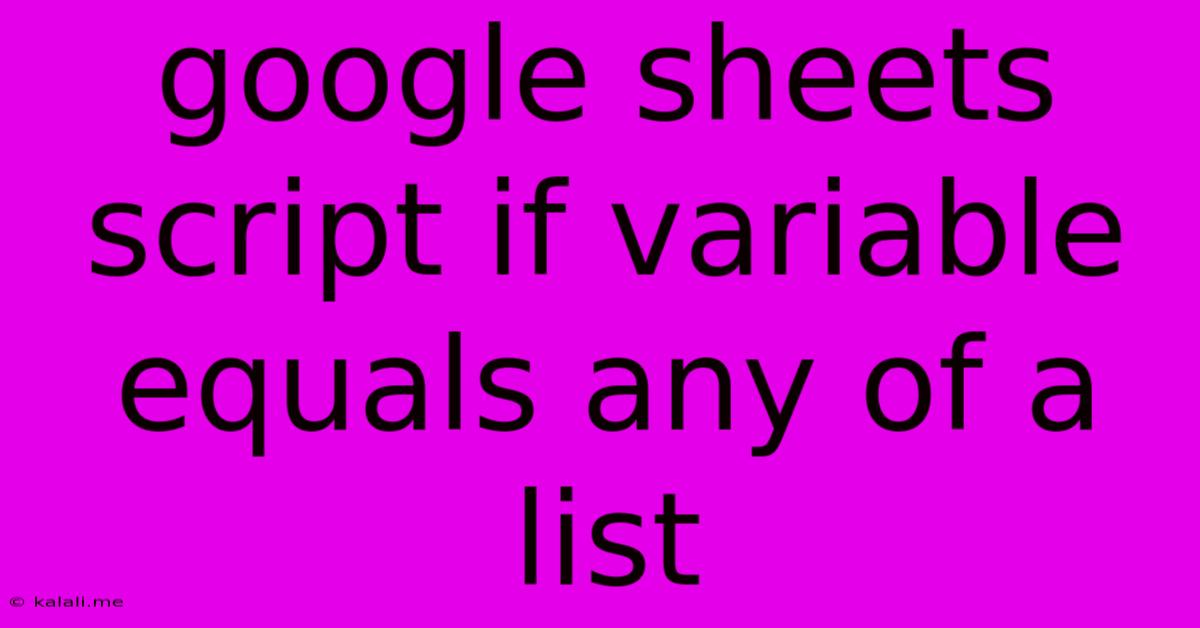
Table of Contents
Google Sheets Script: Checking if a Variable Equals Any Item in a List
This article explores how to efficiently check if a variable's value matches any element within a predefined list in Google Apps Script, specifically within the context of Google Sheets. This is a common task in scripting, useful for conditional logic, data validation, and automating workflows. We'll cover several methods, comparing their efficiency and readability. This guide is perfect for anyone working with Google Sheets and needs to implement robust conditional checks.
Why this is important: Efficiently comparing a variable against a list is crucial for automating tasks in Google Sheets. Whether you're processing data, validating user input, or building more complex applications, this technique forms the foundation for many scripts. Understanding different approaches allows you to choose the most suitable method based on your specific needs and data size.
Method 1: Using indexOf()
The simplest and often most efficient approach for smaller lists involves using the indexOf()
method. This method searches for the specified value within an array and returns its index if found; otherwise, it returns -1.
function checkValueInList(variableToCheck, myList) {
if (myList.indexOf(variableToCheck) !== -1) {
return true; // Value found in the list
} else {
return false; // Value not found
}
}
// Example usage:
let myVariable = "apple";
let myList = ["apple", "banana", "orange"];
let isInList = checkValueInList(myVariable, myList);
Logger.log(isInList); // Output: true
myVariable = "grape";
isInList = checkValueInList(myVariable, myList);
Logger.log(isInList); // Output: false
This method is concise and readily understandable. However, for extremely large lists, its performance might degrade slightly.
Method 2: Using includes()
(Modern Approach)
The includes()
method offers a more modern and arguably more readable approach. It directly returns true
if the list contains the specified value, and false
otherwise.
function checkValueInListIncludes(variableToCheck, myList) {
return myList.includes(variableToCheck);
}
//Example Usage (same as above, but using includes())
let myVariable = "apple";
let myList = ["apple", "banana", "orange"];
let isInList = checkValueInListIncludes(myVariable, myList);
Logger.log(isInList); // Output: true
myVariable = "grape";
isInList = checkValueInListIncludes(myVariable, myList);
Logger.log(isInList); // Output: false
includes()
provides a cleaner syntax, making the code easier to read and maintain. Performance is generally comparable to indexOf()
for most use cases.
Method 3: Iterating through the List (for complex comparisons)
For more complex scenarios where you need to perform more than a simple equality check (e.g., case-insensitive comparison, partial matches), iterating through the list provides greater flexibility.
function checkValueInListIteration(variableToCheck, myList) {
for (let i = 0; i < myList.length; i++) {
if (myList[i].toLowerCase() === variableToCheck.toLowerCase()) { //Case-insensitive example
return true;
}
}
return false;
}
//Example Usage (Case-insensitive comparison)
let myVariable = "Apple";
let myList = ["apple", "banana", "orange"];
let isInList = checkValueInListIteration(myVariable, myList);
Logger.log(isInList); // Output: true
This approach allows for customized comparison logic but is generally less efficient than indexOf()
or includes()
for simple equality checks.
Choosing the Right Method
- For simple equality checks and small to medium-sized lists: Use
includes()
for its readability and generally good performance. - For very large lists: Benchmark both
indexOf()
andincludes()
to determine which performs best in your specific environment. - For complex comparison logic: Iterate through the list, allowing for custom comparison rules.
Remember to consider the size of your list and the complexity of your comparison when selecting the most appropriate method for your Google Sheets script. This will ensure efficient and maintainable code.
Latest Posts
Latest Posts
-
What Did Moses Write About Jesus
May 24, 2025
-
Wire Size For 125 Amp Sub Panel
May 24, 2025
-
Teritoney Operator Handle Null In Apex
May 24, 2025
-
What Is The Mle Of Geometric
May 24, 2025
-
Where Do You Put Up Meaning
May 24, 2025
Related Post
Thank you for visiting our website which covers about Google Sheets Script If Variable Equals Any Of A List . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.