Programmatically Append File Names In A Folder
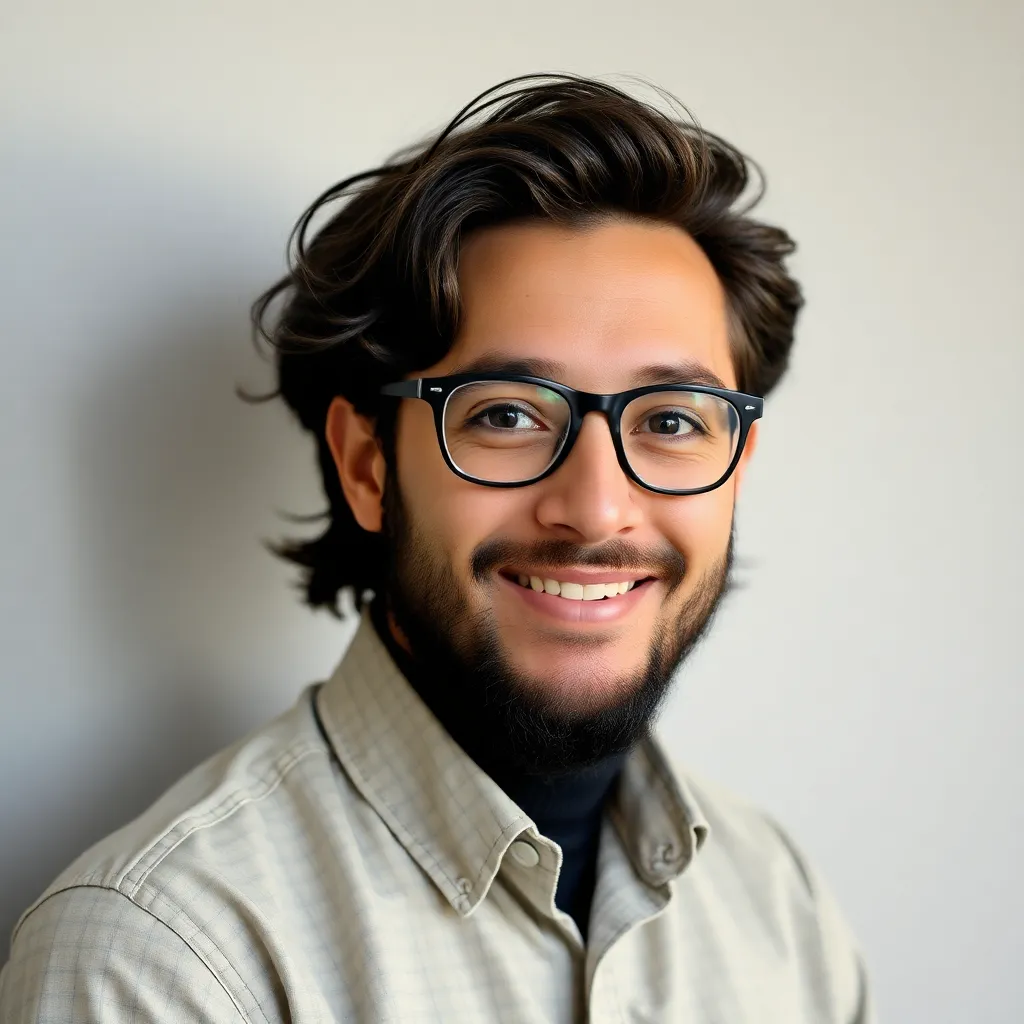
Kalali
May 23, 2025 · 3 min read
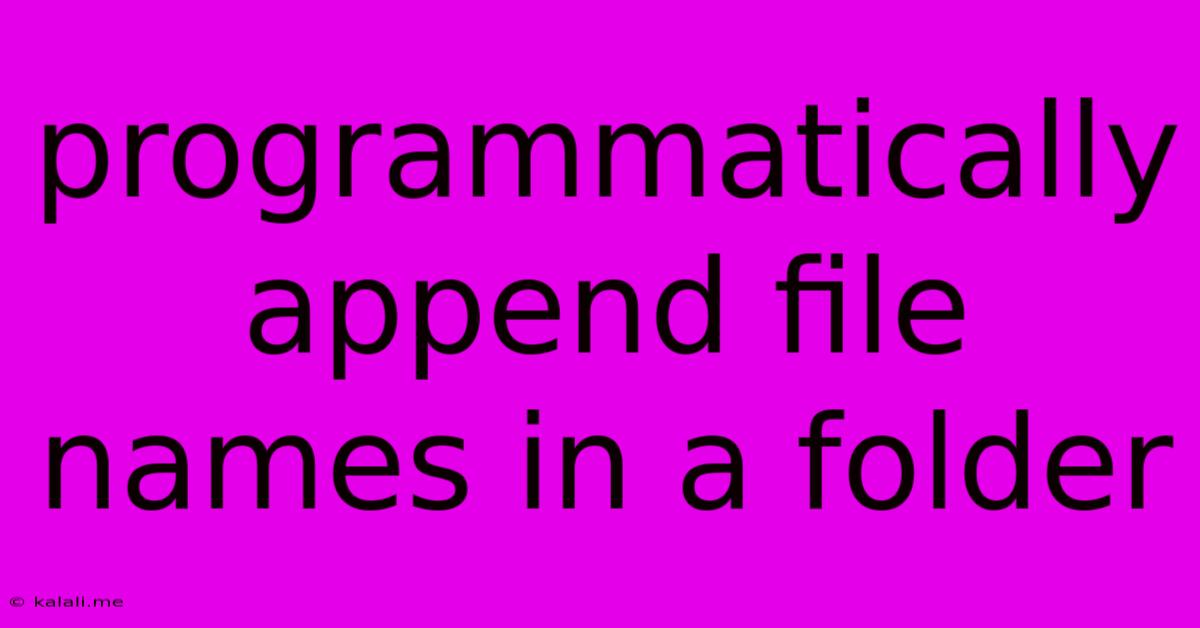
Table of Contents
Programmatically Appending File Names in a Folder: A Comprehensive Guide
This article will guide you through the process of programmatically appending text to filenames within a specified folder. This is a common task in various scripting and programming scenarios, such as batch processing, data management, and file organization. We'll explore solutions using Python, a versatile language well-suited for this type of task. Understanding how to manipulate filenames programmatically is crucial for automating workflows and improving efficiency.
Why Programmatically Append to Filenames?
Appending to filenames offers several advantages over manual renaming:
- Efficiency: Automating the process saves significant time and effort when dealing with a large number of files.
- Consistency: Ensures consistent naming conventions across all files.
- Automation: Integrates seamlessly into larger workflows and scripts.
- Data Management: Facilitates organization and categorization of files based on specific criteria.
Python Solution: A Step-by-Step Guide
Python, with its rich libraries, offers an elegant solution for this task. We'll primarily use the os
and shutil
modules. Let's assume you want to append "_processed" to all .txt
files in a specific directory.
First, ensure you have Python installed. You can then proceed with the following code:
import os
import shutil
def append_to_filenames(directory, extension, append_text):
"""
Appends text to filenames within a specified directory.
Args:
directory: The path to the directory containing the files.
extension: The file extension to target (e.g., ".txt").
append_text: The text to append to the filenames.
"""
for filename in os.listdir(directory):
if filename.endswith(extension):
base, ext = os.path.splitext(filename)
new_filename = base + append_text + ext
source = os.path.join(directory, filename)
destination = os.path.join(directory, new_filename)
os.rename(source, destination)
# Example usage:
directory_path = "/path/to/your/directory" # Replace with your directory path
file_extension = ".txt"
text_to_append = "_processed"
append_to_filenames(directory_path, file_extension, text_to_append)
Remember to replace /path/to/your/directory
with the actual path to your folder. This script iterates through all files in the specified directory, checks if they have the desired extension, and then appends the specified text to the filename before the extension using os.rename
. The shutil
module is not strictly necessary here, as os.rename
handles the renaming efficiently.
Handling Errors and Edge Cases
Robust scripts anticipate potential errors. Here's an improved version:
import os
import shutil
# ... (append_to_filenames function remains the same) ...
try:
append_to_filenames(directory_path, file_extension, text_to_append)
print("Filenames successfully updated.")
except FileNotFoundError:
print(f"Error: Directory '{directory_path}' not found.")
except Exception as e:
print(f"An error occurred: {e}")
This version includes error handling for cases where the directory doesn't exist or other unexpected errors occur during the process.
Beyond Basic Appending
This fundamental approach can be expanded upon:
- Different Append Locations: Modify the code to append text at the beginning or within the filename.
- Multiple Extensions: Use a list of extensions instead of a single string.
- Conditional Appending: Add logic to append only to files meeting specific criteria (e.g., based on file size or modification date).
- Using Regular Expressions: Employ regular expressions for more complex filename manipulations.
This comprehensive guide provides a solid foundation for programmatically appending text to filenames. Remember to always back up your files before running any scripts that modify them. With Python's flexibility and power, you can adapt these techniques to suit your specific file management needs.
Latest Posts
Latest Posts
-
Prove Difference Of Cubes Is Even
May 23, 2025
-
Do You Need Id To Ride The Greyhound Bus
May 23, 2025
-
Ipod Wont Turn On But It Gets Hot
May 23, 2025
-
Water Based Poly Over Oil Stain
May 23, 2025
-
Use Continuity To Evaluate The Limit
May 23, 2025
Related Post
Thank you for visiting our website which covers about Programmatically Append File Names In A Folder . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.