Return Same Instance Of A Class
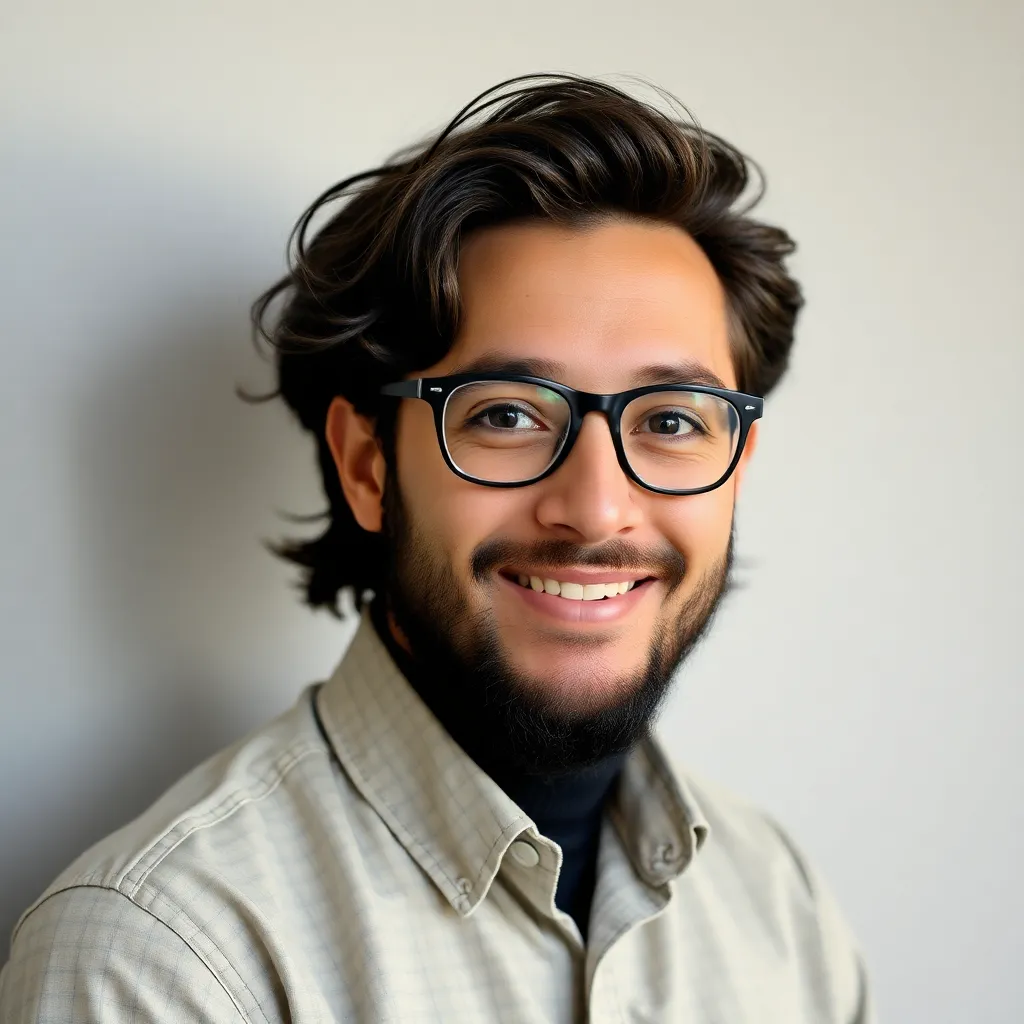
Kalali
May 24, 2025 · 3 min read
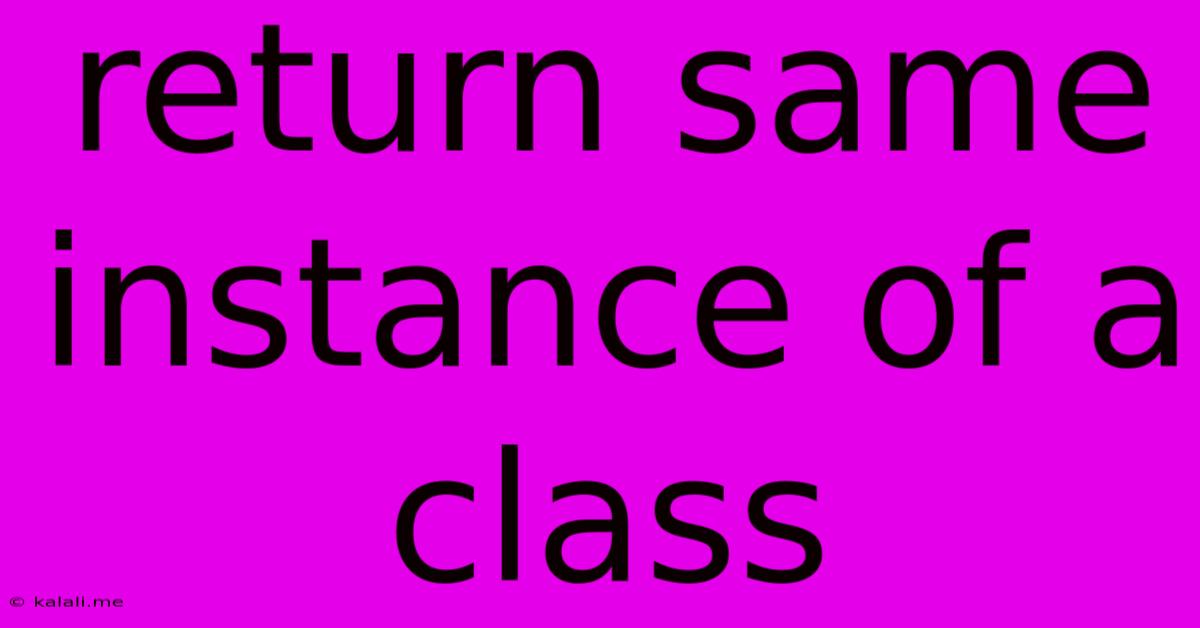
Table of Contents
Returning the Same Instance of a Class: Singleton Pattern and Beyond
This article delves into the concept of returning the same instance of a class in various programming scenarios. This is a crucial design pattern in software development, often employed to manage resources efficiently and ensure consistent behavior. While the Singleton pattern is the most common approach, other methods achieve similar results. This guide explains these methods, their advantages and disadvantages, and when to use them.
The core idea is to restrict the instantiation of a class to one single object. This is particularly useful when you need a single point of access for a specific resource, like a database connection, a logging service, or a configuration manager. Failing to implement this correctly can lead to resource conflicts and unpredictable behavior in your application.
Understanding the Singleton Pattern
The Singleton pattern is a creational design pattern that ensures only one instance of a class is created and provides a global point of access to it. This is often achieved through a private constructor, a static method to return the instance, and a static variable to hold the instance.
Here's a basic example in Python:
class Singleton:
__instance = None
def __init__(self):
if Singleton.__instance is not None:
raise Exception("This class is a singleton!")
else:
Singleton.__instance = self
@staticmethod
def get_instance():
if Singleton.__instance is None:
Singleton()
return Singleton.__instance
# Example usage
s1 = Singleton.get_instance()
s2 = Singleton.get_instance()
print(s1 is s2) # Output: True
This example shows that s1
and s2
both point to the same object. The private constructor prevents direct instantiation, and the get_instance
method ensures that only one instance is ever created.
Advantages of the Singleton Pattern:
- Controlled Access: Provides a single point of access to the object, simplifying management and preventing conflicts.
- Resource Management: Ideal for managing shared resources like database connections, preventing multiple connections.
- State Management: Allows easy management of a single global state.
Disadvantages of the Singleton Pattern:
- Testability: Can make unit testing difficult, as it's harder to isolate and mock the singleton.
- Tight Coupling: Creates tight coupling between the singleton and its users, reducing flexibility.
- Global State: Introduces global state, which can make code harder to understand and debug, especially in larger projects.
Alternatives to the Singleton Pattern
While the Singleton pattern is frequently used, alternatives can be more suitable depending on the context.
-
Dependency Injection: Instead of relying on a global singleton, you can inject the necessary dependencies into the classes that need them. This promotes loose coupling and improved testability.
-
Static Methods: If you only need to perform static operations without maintaining state, static methods can replace the need for a singleton.
-
Module-level variables: For simple, non-complex scenarios, a module-level variable can suffice to hold a single instance of a class. However, this approach lacks the explicit control offered by the singleton pattern.
Choosing the Right Approach
The best approach for returning the same instance of a class depends heavily on your specific needs and the context of your application. Consider the following factors:
- Complexity of the class: For simple classes, a module-level variable might suffice. For more complex classes managing shared resources, the singleton pattern, or dependency injection, might be necessary.
- Testability requirements: Dependency injection generally provides better testability.
- Scalability and maintainability: Singletons can be problematic in large, complex projects.
By carefully considering these factors, you can select the most appropriate method for managing single instances of your classes, ensuring efficiency, maintainability, and robustness in your software. Remember that while the Singleton pattern is powerful, it’s not a universal solution and should be used judiciously.
Latest Posts
Latest Posts
-
What Did Moses Write About Jesus
May 24, 2025
-
Wire Size For 125 Amp Sub Panel
May 24, 2025
-
Teritoney Operator Handle Null In Apex
May 24, 2025
-
What Is The Mle Of Geometric
May 24, 2025
-
Where Do You Put Up Meaning
May 24, 2025
Related Post
Thank you for visiting our website which covers about Return Same Instance Of A Class . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.