Route A Path Across A Grid Randomly
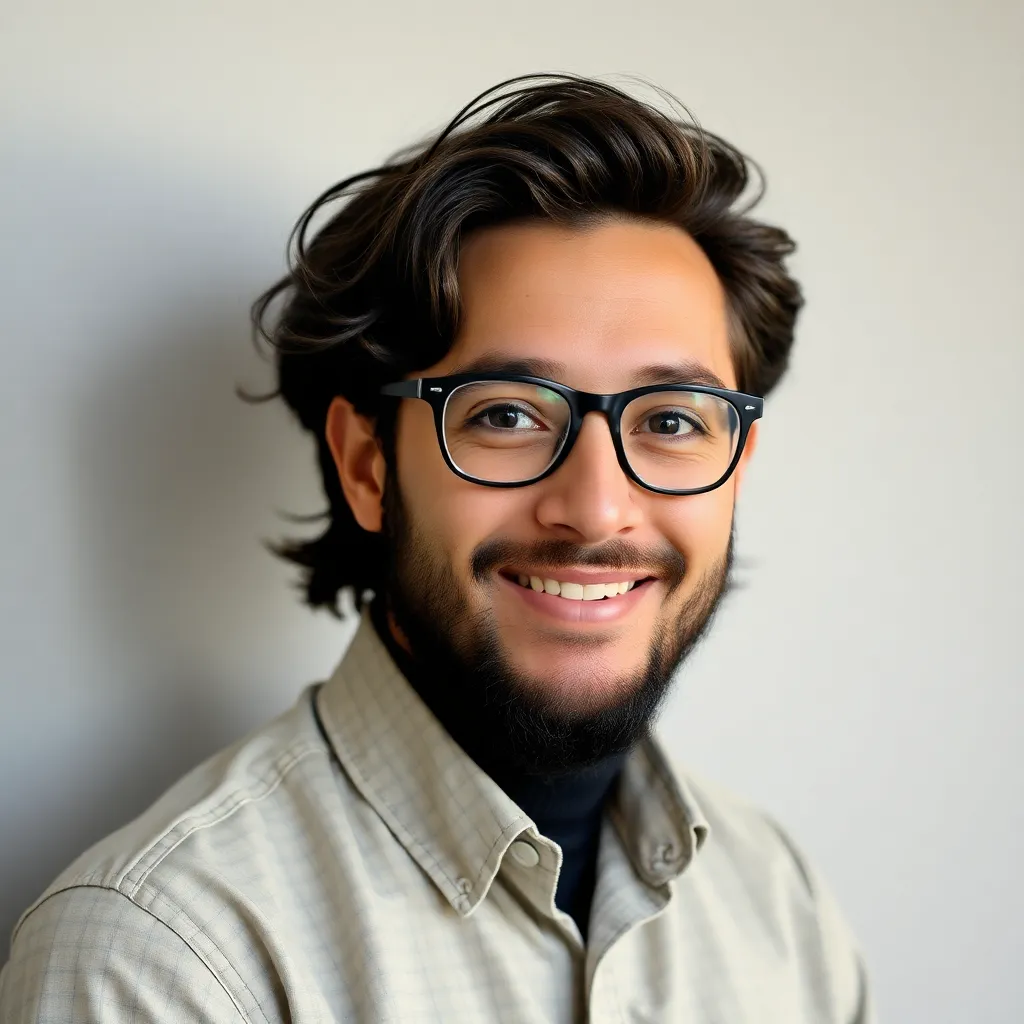
Kalali
May 24, 2025 · 3 min read
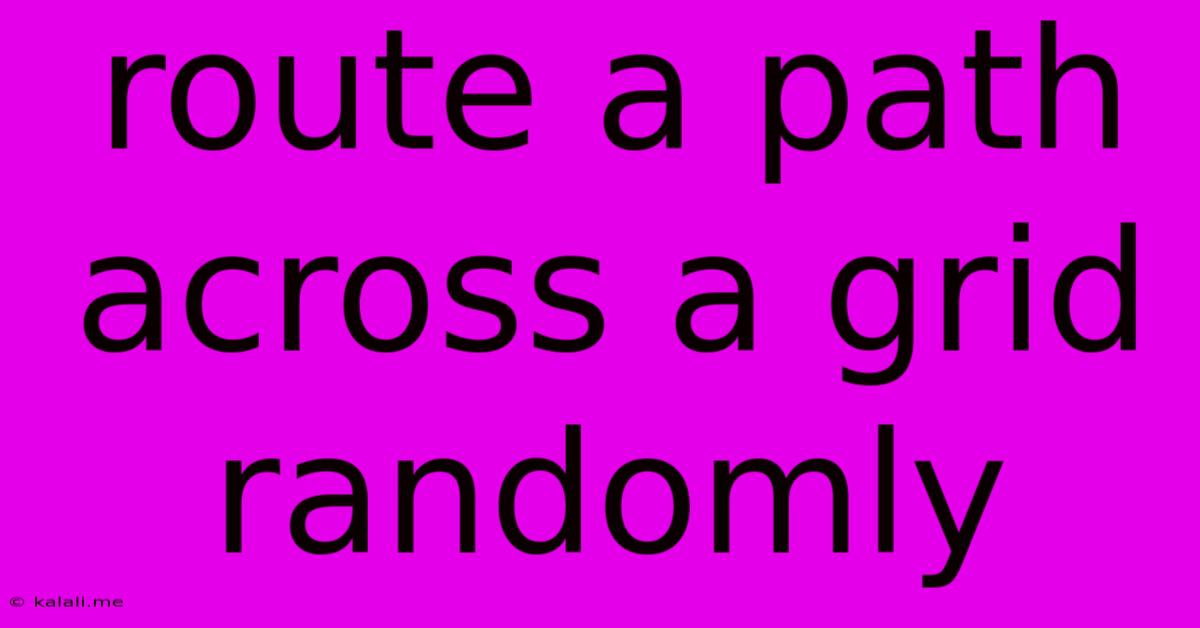
Table of Contents
Random Pathfinding Across a Grid: Algorithms and Implementation
Finding a random path across a grid is a common problem in various fields, from game development (creating random enemy movement) to network simulations (modeling unpredictable data flow). This article explores different approaches to generating these paths, focusing on algorithms and their practical implementation considerations. The core challenge lies in balancing randomness with the constraint of navigating a grid structure. Simply generating random coordinates is inadequate; we need a path that's both random and connected.
This article will cover different methods for creating a random path on a grid, explaining their advantages and disadvantages, and giving insights into their practical applications. We'll cover concepts like path smoothing and optimization, and provide code examples (pseudocode to avoid platform-specific dependencies) to illustrate the process.
Methods for Generating Random Paths
Several algorithms can achieve random pathfinding on a grid. The choice depends on the desired level of randomness and the specific constraints of the application. Here are a few popular methods:
1. Random Walk:
This is the simplest approach. Starting at a specified point, the algorithm randomly chooses one of the four (or eight, including diagonals) adjacent cells to move to, continuing until a target cell is reached or a maximum number of steps is exceeded. This method can produce paths that are quite erratic and may not efficiently reach the target.
- Advantages: Simple to implement.
- Disadvantages: Can be inefficient, leading to long or convoluted paths. May not reach the target.
Pseudocode:
function randomWalk(start, end):
path = [start]
current = start
while current != end and steps < maxSteps:
neighbors = getAdjacentCells(current)
next = randomChoice(neighbors)
path.append(next)
current = next
return path
2. Drunkard's Walk with Backtracking:
This method improves upon the basic random walk by allowing backtracking. If a chosen step leads to a dead end or an undesirable situation, the algorithm backtracks to a previous point and tries a different direction. This helps to avoid getting stuck.
- Advantages: Less likely to get stuck in dead ends compared to a simple random walk.
- Disadvantages: Still can generate inefficient or convoluted paths. Computationally more expensive than a simple random walk.
3. Recursive Backtracker Algorithm:
This approach is more sophisticated, commonly used in maze generation, and can also generate random paths. It operates by recursively exploring the grid, randomly choosing a direction to move and marking the path. This ensures connectivity, resulting in a single continuous path.
- Advantages: Guarantees a connected path. Produces more structured, less erratic paths than simple random walks.
- Disadvantages: More complex to implement than simpler methods.
4. A with Random Heuristic:*
This method utilizes the A* search algorithm, a widely used pathfinding algorithm, but incorporates a randomized heuristic function. The heuristic guides the search, but the randomness in the heuristic creates variability in the resulting path. This strikes a balance between efficiency and randomness.
- Advantages: Efficient in finding paths. The degree of randomness is controllable via the heuristic function.
- Disadvantages: More complex to implement than simpler methods. Requires understanding of the A* algorithm.
Optimizing Random Paths
Once a random path is generated, it might be beneficial to optimize it. This can involve techniques like:
- Path Smoothing: Removing unnecessary bends or zigzags in the path. This can be done by finding shortcuts between points on the path.
- Path Shortening: Finding a shorter path that still retains a degree of randomness.
Conclusion
Generating a random path across a grid involves a trade-off between randomness and efficiency. The choice of algorithm depends on the specific application and its requirements. Simple methods like random walks are easy to implement, while more sophisticated techniques like A* with random heuristics offer better control and efficiency. Post-processing techniques like path smoothing can further refine the generated paths. Remember to consider the computational cost and the desired level of randomness when selecting an algorithm.
Latest Posts
Latest Posts
-
What Is Half Of 1 4 Teaspoon
Jul 02, 2025
-
How Many Cups In A Pound Of Hamburger Meat
Jul 02, 2025
-
Imagery Or Figurative Language From Romeo And Juliet
Jul 02, 2025
-
What Is A Quarter Of A Million
Jul 02, 2025
-
Which Of The Following Is True Concerning A Dao
Jul 02, 2025
Related Post
Thank you for visiting our website which covers about Route A Path Across A Grid Randomly . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.