Bash Loop Through Lines In File
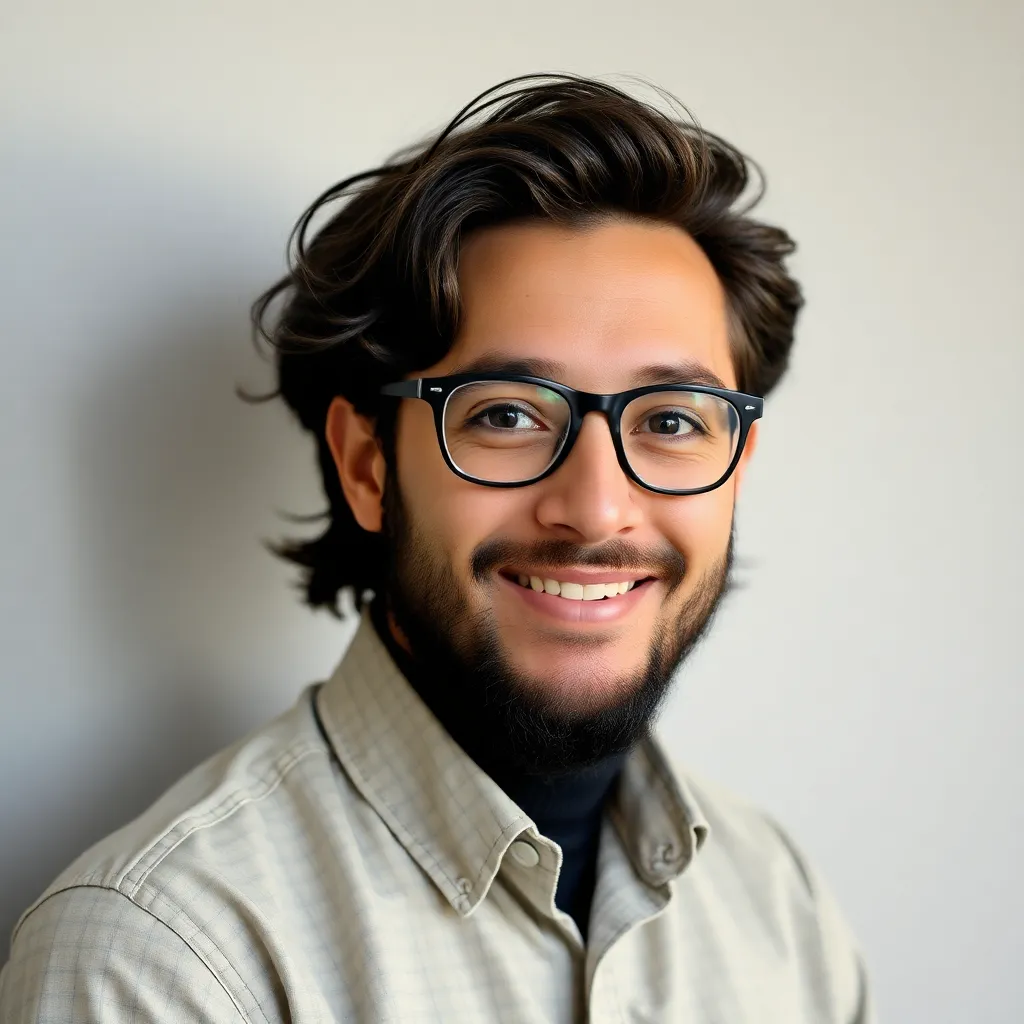
Kalali
May 27, 2025 · 3 min read
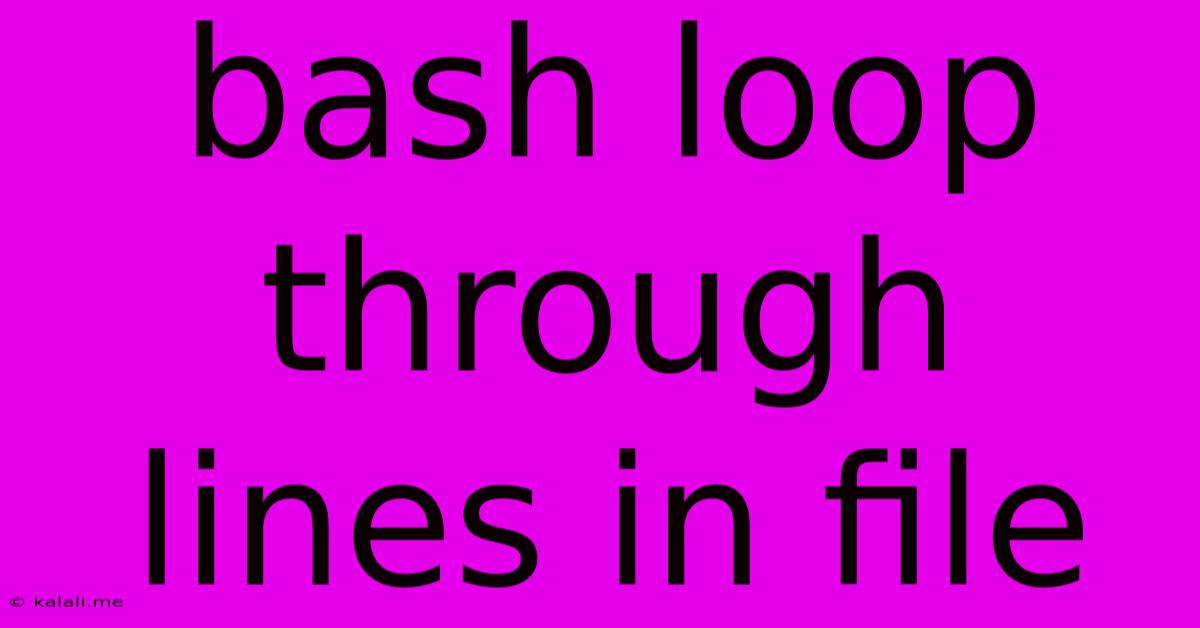
Table of Contents
Bash Loop Through Lines in File: A Comprehensive Guide
This article provides a comprehensive guide on how to iterate through lines in a file using Bash scripting. Learning this fundamental skill is crucial for automating tasks and processing data efficiently in a Linux environment. We'll cover several methods, each with its strengths and weaknesses, allowing you to choose the best approach for your specific needs. This guide is perfect for beginners and intermediate users alike, offering practical examples and explanations.
Why Loop Through Lines in a File?
Bash scripting, combined with file processing, enables automation of repetitive tasks. Imagine needing to process a large log file, extract specific data from a CSV, or modify thousands of configuration files. Looping through lines in a file allows you to efficiently handle these scenarios without manual intervention. Common use cases include data cleaning, log analysis, and automated system administration.
Methods for Iterating Through Lines
We'll explore three primary methods for traversing file lines: while read
, for loop
, and mapfile
.
1. The while read
Loop
This is arguably the most common and arguably the easiest method. It's incredibly straightforward and easy to understand, making it ideal for beginners.
while IFS= read -r line; do
# Process each line here
echo "Processing: $line"
done < "my_file.txt"
IFS=
: Prevents word splitting. This is crucial to handle lines with spaces correctly.-r
: Prevents backslash escapes from being interpreted. This ensures that the line is read literally.< "my_file.txt"
: This redirects the content ofmy_file.txt
to thewhile
loop's standard input.
2. The for
Loop
The for
loop offers a slightly more concise syntax, especially when you don't need advanced line manipulation.
for line in $(cat "my_file.txt"); do
# Process each line here
echo "Processing: $line"
done
Important Note: While this approach appears simpler, it's less robust than while read
. It's vulnerable to word splitting and globbing if lines contain spaces or special characters. Therefore, the while read
method is generally preferred for its reliability.
3. Using mapfile
(Bash 4.0 and later)
mapfile
is a powerful command available in Bash 4.0 and later. It reads the entire file into an array, allowing for efficient random access. This is beneficial when you need to revisit specific lines later in your script.
mapfile -t lines < "my_file.txt"
for i in "${!lines[@]}"; do
echo "Line $((i+1)): ${lines[i]}"
done
mapfile -t lines
: Reads the file into an array namedlines
. The-t
option removes trailing newlines."${!lines[@]}"
: This iterates through the indices of the array, providing a cleaner way to access each line.
Handling Empty Lines and Special Characters
Empty lines can be handled using conditional statements within your loops:
while IFS= read -r line; do
if [[ -n "$line" ]]; then
# Process only non-empty lines
echo "Processing: $line"
fi
done < "my_file.txt"
For files containing special characters, using -r
with while read
is vital to avoid unexpected behavior.
Choosing the Right Method
- For simplicity and robustness, especially with complex lines, use the
while IFS= read -r line
loop. - For a quick and less robust solution, if lines are simple and don't contain spaces or special characters, the
for
loop might suffice. - If you need random access to lines or efficient processing of large files,
mapfile
is the best choice.
This comprehensive guide provides the knowledge and tools to effectively loop through lines in a file using Bash. Remember to select the method that best suits your specific requirements and always prioritize robustness and error handling. Mastering these techniques will significantly enhance your Bash scripting capabilities.
Latest Posts
Latest Posts
-
How Many Inches Is Half A Yard
Jul 03, 2025
-
How Old Are You If Your Born In 1996
Jul 03, 2025
-
How Many Water Bottles In 64 Ounces
Jul 03, 2025
-
How Many Cups Is 16 Oz Of Peanut Butter
Jul 03, 2025
-
How Long Is Mayonnaise Good For After Expiration Date
Jul 03, 2025
Related Post
Thank you for visiting our website which covers about Bash Loop Through Lines In File . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.